Write a Java program to print 1 and 0 in alternative rectangle columns using for loop.
import java.util.Scanner; public class AlternativeColumns1and01 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Numbers of Rows = "); int rows = sc.nextInt(); System.out.print("Enter Numbers of Columns = "); int columns = sc.nextInt(); System.out.println("Printing 1 and 0 Numbers in Alternative Columns"); for (int i = 1 ; i <= rows; i++ ) { for (int j = 1 ; j <= columns; j++ ) { if(j % 2 == 0) { System.out.printf("0 "); } else { System.out.printf("1 "); } } System.out.println(); } } }
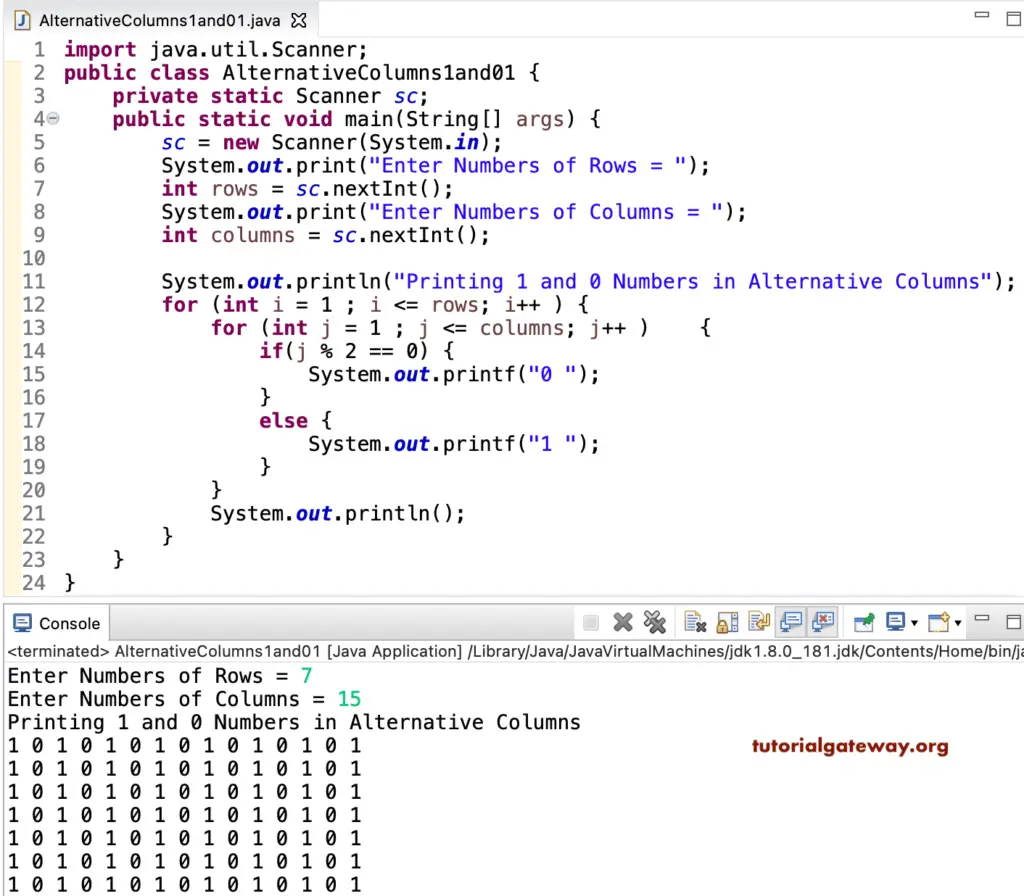
This Java example prints the rectangle of 1 and 0 in alternative columns using a while loop.
import java.util.Scanner; public class AlternativeColumns1and02 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Numbers of Rows and Columns = "); int rows = sc.nextInt(); int columns = sc.nextInt(); System.out.println("Printing 1 and 0 Numbers in Alternative Columns"); for (int i = 1 ; i <= rows; i++ ) { for (int j = 1 ; j <= columns; j++ ) { System.out.printf("%d", j% 2); } System.out.println(); } } }
Enter Numbers of Rows and Columns = 8 15
Printing 1 and 0 Numbers in Alternative Columns
101010101010101
101010101010101
101010101010101
101010101010101
101010101010101
101010101010101
101010101010101
101010101010101
Java Program to Print 1 and 0 in alternative columns of a rectangle using do while loop.
import java.util.Scanner; public class AlternativeColumns1and03 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i = 1, j; System.out.print("Enter Numbers of Rows and Columns = "); int rows = sc.nextInt(); int columns = sc.nextInt(); System.out.println("Printing 1 and 0 Numbers in Alternative Columns"); while ( i <= rows ) { j = 1 ; while( j <= columns ) { System.out.printf("%d", j% 2); j++; } System.out.println(); i++; } } }
Enter Numbers of Rows and Columns = 10 20
Printing 1 and 0 Numbers in Alternative Columns
10101010101010101010
10101010101010101010
10101010101010101010
10101010101010101010
10101010101010101010
10101010101010101010
10101010101010101010
10101010101010101010
10101010101010101010
10101010101010101010
This Java example prints the rectangle of 0 and 1 in alternative columns.
import java.util.Scanner; public class AlternativeColumns1and04 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Numbers of Rows and Columns = "); int rows = sc.nextInt(); int columns = sc.nextInt(); System.out.println("Printing 0 and 1 Numbers in Alternative Columns"); for (int i = 1 ; i <= rows; i++ ) { for (int j = 1 ; j <= columns; j++ ) { if(j % 2 == 0) { System.out.printf("1"); } else { System.out.printf("0"); } } System.out.println(); } } }
Enter Numbers of Rows and Columns = 14 28
Printing 0 and 1 Numbers in Alternative Columns
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101
0101010101010101010101010101