Write a Java Program to find the sum of each Matrix Row and Column with an example. Or Java Program to calculate the sum of each and every row and column in a given Matrix or multi-dimensional array. In this Java sum of Matrix row and column example, we declared a 3 * 3 SumOfRowCols_arr integer matrix with random values. Next, we used for loop to iterate the SumOfRowCols_arr Matrix items. Within the for loop, we are calculating the SumOfRowCols_arr Matrix sum of rows and columns.
public class SumOfMatrixRowsColumns { public static void main(String[] args) { int i, j, row_sum, column_sum; int[][] SumOfRowCols_arr = {{11, 21, 31}, {41, 51, 61}, {71, 81, 91}}; for(i = 0; i < SumOfRowCols_arr.length; i++) { row_sum = 0; column_sum = 0; for(j = 0; j < SumOfRowCols_arr[0].length; j++) { row_sum = row_sum + SumOfRowCols_arr[i][j]; column_sum = column_sum + SumOfRowCols_arr[j][i]; } System.out.println("\nThe Sum of Matrix Items " + "in Row " + i + " = " + row_sum); System.out.println("\nThe Sum of Matrix Items " + "in Column " + i + " = " + column_sum); } } }
Java Sum of Matrix Rows and Column output
The Sum of Matrix Items in Row 0 = 63
The Sum of Matrix Items in Column 0 = 123
The Sum of Matrix Items in Row 1 = 153
The Sum of Matrix Items in Column 1 = 153
The Sum of Matrix Items in Row 2 = 243
The Sum of Matrix Items in Column 2 = 183
Java Program to find Sum of Matrix Rows and Column example 2
This Java Matrix sum of rows and columns code is the same as the above. However, we used two separate for loops to Calculate the Sum of rows and columns. I suggest you refer to the Java Sum of each column and Java Sum of each Row examples.
public class SumOfMatrixRowsColumns { public static void main(String[] args) { int i, j, row_sum, column_sum; int[][] SumOfRowCols_arr = {{11, 21, 31}, {41, 51, 61}, {71, 81, 91}}; System.out.println("\nThe Sum of Each Row in a Matrix"); for(i = 0; i < SumOfRowCols_arr.length; i++) { row_sum = 0; for(j = 0; j < SumOfRowCols_arr[0].length; j++) { row_sum = row_sum + SumOfRowCols_arr[i][j]; } System.out.println("The Sum of Matrix Items " + "in Row " + i + " = " + row_sum); } System.out.println("\nThe Sum of Each Column in a Matrix"); for(i = 0; i < SumOfRowCols_arr.length; i++) { column_sum = 0; for(j = 0; j < SumOfRowCols_arr[0].length; j++) { column_sum = column_sum + SumOfRowCols_arr[j][i]; } System.out.println("The Sum of Matrix Items " + "in Column " + i + " = " + column_sum); } } }
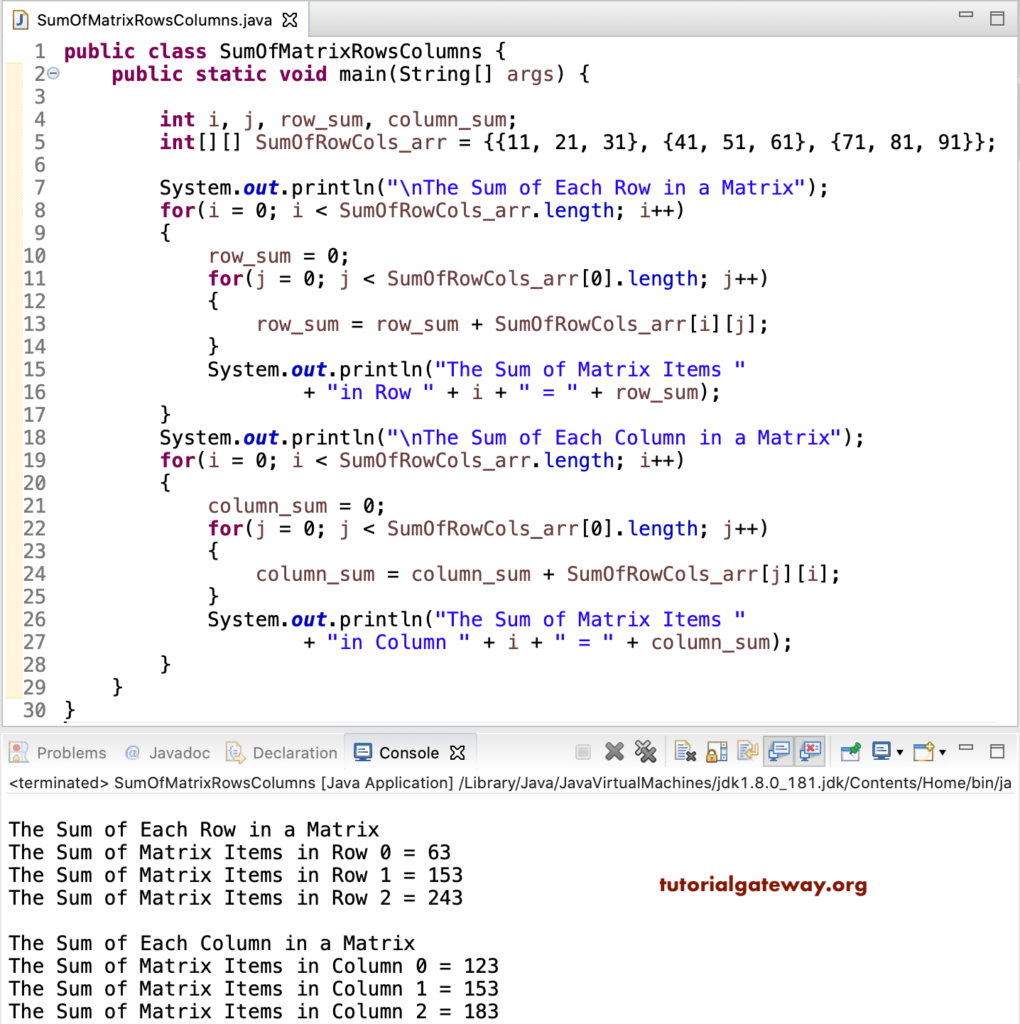
This Java Matrix sum of columns and rows code is the same as the above. However, this Java code for sum of matrix column and rows allows the user to enter the rows, columns, and the matrix items.
import java.util.Scanner; public class SumOfMatrixRowsColumns { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns, row_sum, column_sum; sc= new Scanner(System.in); System.out.println("\n Enter Matrix Rows and Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] SumOfRowCols_arr = new int[rows][columns]; System.out.println("\n Please Enter the Matrix Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { SumOfRowCols_arr[i][j] = sc.nextInt(); } } System.out.println("\nThe Sum of Each Row in a Matrix"); for(i = 0; i < SumOfRowCols_arr.length; i++) { row_sum = 0; for(j = 0; j < SumOfRowCols_arr[0].length; j++) { row_sum = row_sum + SumOfRowCols_arr[i][j]; } System.out.println("The Sum of Matrix Items " + "in Row " + i + " = " + row_sum); } System.out.println("\nThe Sum of Each Column in a Matrix"); for(i = 0; i < SumOfRowCols_arr.length; i++) { column_sum = 0; for(j = 0; j < SumOfRowCols_arr[0].length; j++) { column_sum = column_sum + SumOfRowCols_arr[j][i]; } System.out.println("The Sum of Matrix Items " + "in Column " + i + " = " + column_sum); } } }
Enter Matrix Rows and Columns :
3 3
Please Enter the Matrix Items :
15 25 35
45 55 65
75 85 95
The Sum of Each Row in a Matrix
The Sum of Matrix Items in Row 0 = 75
The Sum of Matrix Items in Row 1 = 165
The Sum of Matrix Items in Row 2 = 255
The Sum of Each Column in a Matrix
The Sum of Matrix Items in Column 0 = 135
The Sum of Matrix Items in Column 1 = 165
The Sum of Matrix Items in Column 2 = 195