Write a Java program to find student Grade with example. For this, first, we have to calculate the Total and Percentage of given Subjects.
Java Program to Find Student Grade Example 1
This program helps the user to enter five different integer values for five subjects. Next, it finds the Total and Percentage of those Five Subjects.
For this, we are using the Java Arithmetic Operators to perform arithmetic operations. Next, we used the Java Else If statement to display the Grade.
// Java Program to Find Student Grade import java.util.Scanner; public class StudentGrade { private static Scanner sc; public static void main(String[] args) { int english, chemistry, computers, physics, maths; float total, Percentage; sc = new Scanner(System.in); System.out.print(" Please Enter the Five Subjects Marks : "); english = sc.nextInt(); chemistry = sc.nextInt(); computers = sc.nextInt(); physics = sc.nextInt(); maths = sc.nextInt(); total = english + chemistry + computers + physics + maths; Percentage = (total / 500) * 100; System.out.println(" Total Marks = " + total); System.out.println(" Marks Percentage = " + Percentage); if(Percentage >= 90) { System.out.println("\n Grade A"); } else if(Percentage >= 80) { System.out.println("\n Grade B"); } else if(Percentage >= 70) { System.out.println("\n Grade C"); } else if(Percentage >= 60) { System.out.println("\n Grade D"); } else if(Percentage >= 40) { System.out.println("\n Grade E"); } else { System.out.println("\n Fail"); } } }
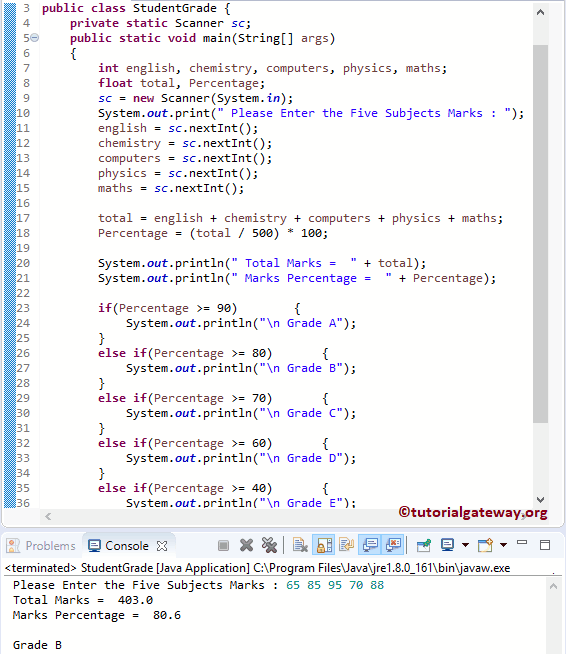
Java Program for Student Grade Example 2
This program is the same as above. But this time, we are creating a separate Java method to display the student grades.
// Java Program to Find Student Grade import java.util.Scanner; public class StudentGrade1 { private static Scanner sc; public static void main(String[] args) { int english, chemistry, computers, physics, maths; sc = new Scanner(System.in); System.out.print(" Please Enter the Five Subjects Marks : "); english = sc.nextInt(); chemistry = sc.nextInt(); computers = sc.nextInt(); physics = sc.nextInt(); maths = sc.nextInt(); studentGrade(english, chemistry, computers, physics, maths); } public static void studentGrade(int eng, int chem, int com, int phy, int math) { float total, Percentage; total = eng + chem + com + phy + math; Percentage = (total / 500) * 100; System.out.println(" Total Marks = " + total); System.out.println(" Marks Percentage = " + Percentage); if(Percentage >= 90) { System.out.println("\n Grade A"); } else if(Percentage >= 80) { System.out.println("\n Grade B"); } else if(Percentage >= 70) { System.out.println("\n Grade C"); } else if(Percentage >= 60) { System.out.println("\n Grade D"); } else if(Percentage >= 40) { System.out.println("\n Grade E"); } else { System.out.println("\n Fail"); } } }
Java student grades output
Please Enter the Five Subjects Marks : 80 80 70 85 94
Total Marks = 409.0
Marks Percentage = 81.8
Grade B