Write a Java Program to Find the Second Largest Array Number with an example or find the second largest element or item in a given array.
Java Program to Find the Second Largest Array Number using for loop
This Java array example allows users to enter the secLrg_arr size and the items. Next, the for loop iterates the array items. Within the loop, we use the Else If statement to find the largest and the second largest event in this secLrg_arr.
import java.util.Scanner; public class Simple { private static Scanner sc; public static void main(String[] args) { int Size, i, first, snd, lg_Position = 0; sc = new Scanner(System.in); System.out.print("\nPlease Enter the SEC LRG size : "); Size = sc.nextInt(); int[] secLrg_arr = new int[Size]; System.out.format("\nEnter SEC LRG %d elements : ", Size); for(i = 0; i < Size; i++) { secLrg_arr[i] = sc.nextInt(); } first = snd = Integer.MIN_VALUE; for(i = 0; i < Size; i++) { if(secLrg_arr[i] > first) { snd = first; first = secLrg_arr[i]; lg_Position = i; } else if(secLrg_arr[i] > snd && secLrg_arr[i] < first) { snd = secLrg_arr[i]; } } System.out.format("\nMaximum Value in SEC LRG Array = %d", first); System.out.format("\nMaximum Value Index position = %d", lg_Position); System.out.format("\n\nSecond Largest Item in SEC LRG Array = %d", snd); } }
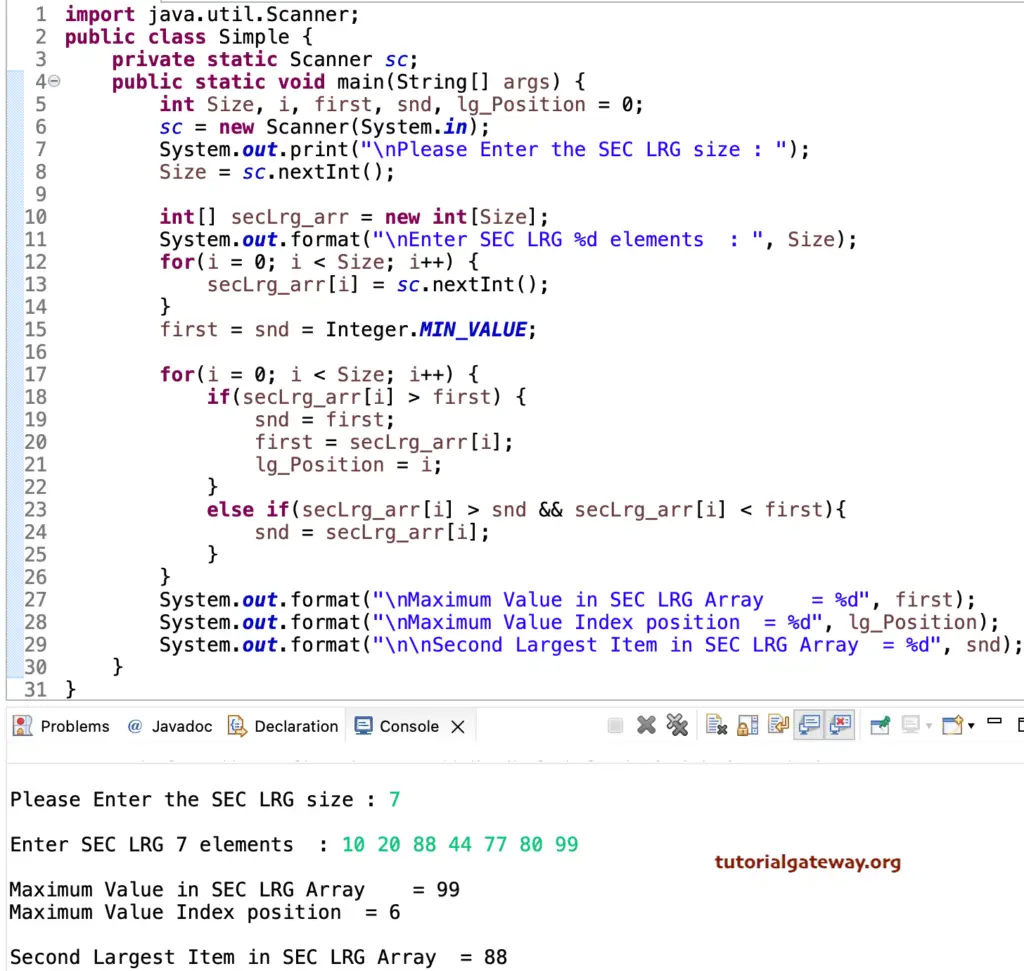
The Second Largest Array Number using functions
In this code, we created a separate SecLargElem function. It helps to find and return the second largest item in a given array.
package ArrayPrograms; import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int Sz, i; sc = new Scanner(System.in); System.out.print("\nPlease Enter the SEC LRG size : "); Sz = sc.nextInt(); int[] secLrg_arr = new int[Sz]; System.out.format("\nEnter SEC LRG %d elements : ", Sz); for(i = 0; i < Sz; i++) { secLrg_arr[i] = sc.nextInt(); } SecLargElem(secLrg_arr, Sz); } public static void SecLargElem(int[] secLrg_arr, int Sz) { int i, first, sec, lg_Position = 0; first = sec = Integer.MIN_VALUE; for(i = 0; i < Sz; i++) { if(secLrg_arr[i] > first) { sec = first; first = secLrg_arr[i]; lg_Position = i; } else if(secLrg_arr[i] > sec && secLrg_arr[i] < first) { sec = secLrg_arr[i]; } } System.out.format("\\nHighest Item in SEC LRG = %d", first); System.out.format("\nIndex position of Highest = %d", lg_Position); System.out.format("\n\nSecond Largest Item in SEC LRG = %d", sec); } }
Please Enter the SEC LRG size : 11
Enter SEC LRG 11 elements : 10 20 44 77 89 95 200 22 88 250 111
Highest Item in SEC LRG = 250
Index position of Highest = 9
Second Largest Item in SEC LRG = 200