Write a Java Program to Find Number of Days in a Month using Else If statement, and Switch Case with examples. The total number of days in :
- January, March, May, August, October, and December = 31 Days
- February = 28 or 29 Days
- April, June, September, and November = 30 Days
Java Program to Find Number of Days in a Month using Else If
This program asks the user to enter any number between 1 to 12, where 1 = January, 2 = February, 3 = March,………., and 12 = December. Based on the user entered integer value, the program prints the number of days in that particular month. To accomplish this goal, we are using Java Else If Statement.
// Java Program to Find Number of Days in a Month import java.util.Scanner; public class DaysinMonth1 { private static Scanner sc; public static void main(String[] args) { int month; sc = new Scanner(System.in); System.out.print(" Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : "); month = sc.nextInt(); if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12 ) { System.out.println("\n 31 Days in this Month"); } else if ( month == 4 || month == 6 || month == 9 || month == 11 ) { System.out.println("\n 30 Days in this Month"); } else if ( month == 2 ) { System.out.println("\n Either 28 or 29 Days in this Month"); } else System.out.println("\n Please enter Valid Number between 1 to 12"); } }
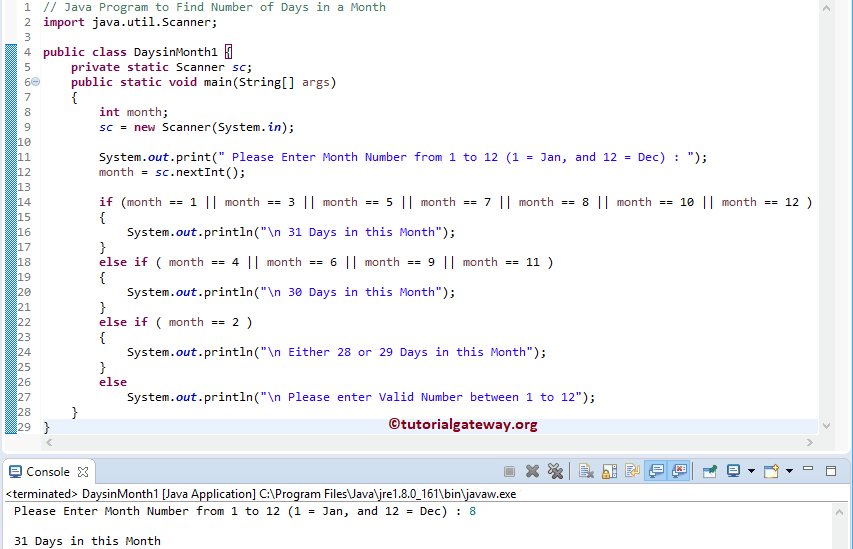
Let me enter the month = 11
Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : 11
Either 30 Days in this Month
Let me enter the month = 2
Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : 2
Either 28 or 29 Days in this Month
This time, we enter the wrong value: 15
Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : 15
Please enter Valid Number between 1 to 12
Java Program to Find Number of Days in a Month using Switch Case
Itis an ideal approach to deal with multiple conditions. In this Java program, we are using the Switch Case to return the number of days in a month.
// Java Program to Find Number of Days in a Month import java.util.Scanner; public class DaysinMonth2 { private static Scanner sc; public static void main(String[] args) { int month; sc = new Scanner(System.in); System.out.print(" Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : "); month = sc.nextInt(); switch(month) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: System.out.println("\n 31 Days in this Month"); break; case 4: case 6: case 9: case 11: System.out.println("\n 30 Days in this Month"); break; case 2: System.out.println("\n Either 28 or 29 Days in this Month"); break; default: System.out.println("\n Please enter Valid Number between 1 to 12"); } } }
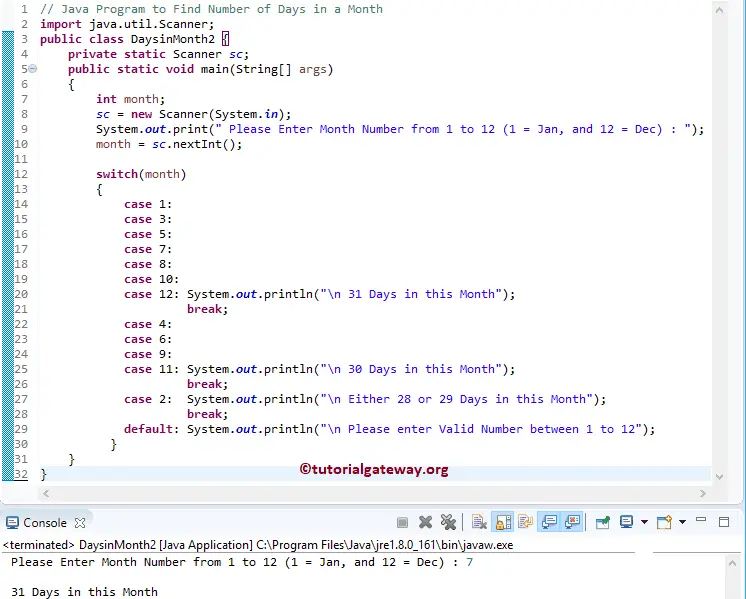
Let me try different value
Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : 4
30 Days in this Month
Java Program to get Number of Days in a Month using Method
This Java program to get number of days in a month is the same as the first example. But we separated the logic and placed it in a separate method.
// Java Program to Find Number of Days in a Month import java.util.Scanner; public class DaysinMonth3 { private static Scanner sc; public static void main(String[] args) { int month; sc = new Scanner(System.in); System.out.print(" Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : "); month = sc.nextInt(); DaysinaMonth(month); } public static void DaysinaMonth(int month) { if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12 ) { System.out.println("\n 31 Days in this Month"); } else if ( month == 4 || month == 6 || month == 9 || month == 11 ) { System.out.println("\n 30 Days in this Month"); } else if ( month == 2 ) { System.out.println("\n Either 28 or 29 Days in this Month"); } else System.out.println("\n Please enter Valid Number between 1 to 12"); } }
Please Enter Month Number from 1 to 12 (1 = Jan, and 12 = Dec) : 12
31 Days in this Month
Comments are closed.