Write a Java Program to find the LCM of Two Numbers using the While Loop and recursive method. According to Mathematics, the LCM (Least Common Multiple) of two or more integers is the smallest positive integer divisible by the assigned integer values (without remainder).
For example, the LCM of 2 and 3 is 6 because 6 is the smallest positive integer divisible by 2 and 3.
Java Program to find LCM of Two Numbers using While Loop
This Java program allows the user to enter two positive integer values. Next, in this program, we are using the While Loop and a temporary variable to calculate the LCM of those two positive integers.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int n1, n2, Temp, GCD = 0, LM = 0; sc = new Scanner(System.in); System.out.print("Please Enter the First Integer Value : "); n1 = sc.nextInt(); System.out.print("Please Enter the Second Integer Value : "); n2 = sc.nextInt(); int a = n1; int b = n2; while(n2 != 0) { Temp = n2; n2 = n1 % n2; n1 = Temp; } GCD = n1; System.out.println("\nGCD of " + a + " and " + b + " = " + GCD); LM = (a * b) / GCD; System.out.println("LCM of " + a + " and " + b + " = " + LM); } }
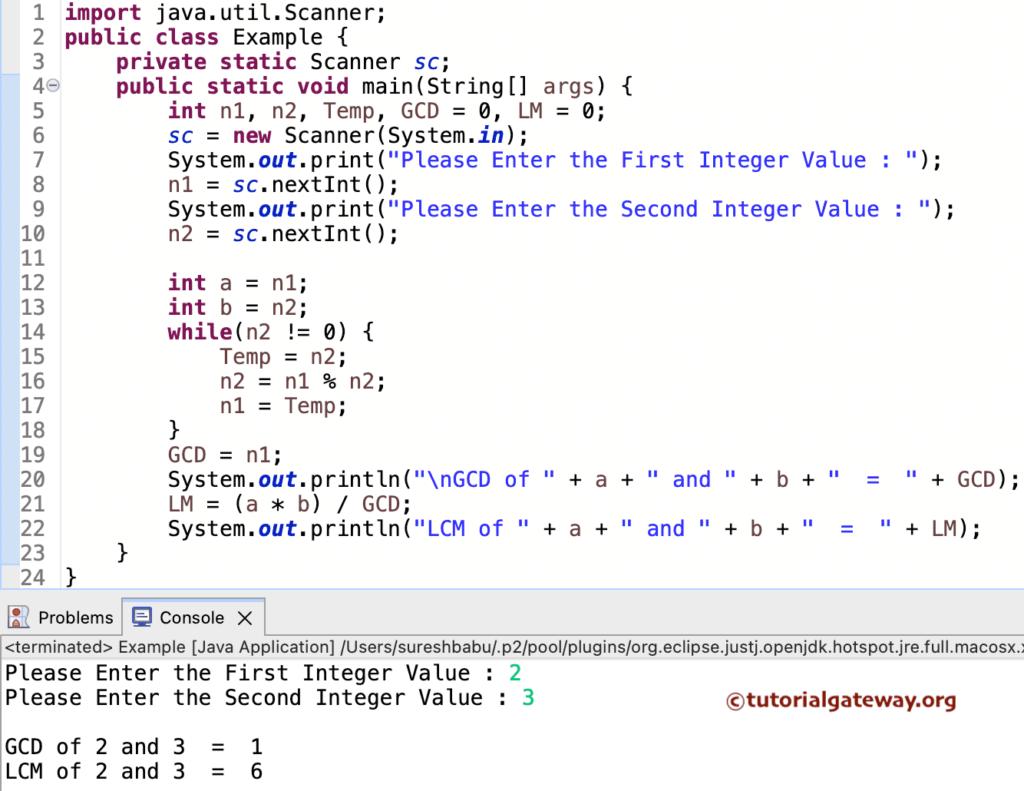
Analysis
n1 = 2 and n2 = 3
The while loop will iterate until the condition inside it, i.e., n2 != 0, is false. So, let me show you the code execution in iteration-wise.
First Iteration: n2 ! = 0 means 3 != 0 is true.
- temp = 3
- n2 = 2 % 3 = 1
- n1 = temp = 3
Second Iteration: 1 != 0 is true.
- temp = 1
- n2 = 3 % 1 = 0
- n1 = 1
The condition while (n2 != 0) becomes False. So, Javac exits from the while loop.
GCD = n1 = 1
LCM = (2 * 3) / 1 = 6.
Java Program to find LCM of Two Numbers without using Temp
This program calculates the Least Common Multiple without using any temporary variable.
import java.util.Scanner; public class LCMofTwo2 { private static Scanner sc; public static void main(String[] args) { int Num1, Num2, GCD = 0, LCM = 0; sc = new Scanner(System.in); System.out.print(" Please Enter the First Integer Value : "); Num1 = sc.nextInt(); System.out.print(" Please Enter the Second Integer Value : "); Num2 = sc.nextInt(); int a = Num1; int b = Num2; while(Num2 != 0) { if(Num1 > Num2) { Num1 = Num1 - Num2; } else { Num2 = Num2 - Num1; } } GCD = Num1; System.out.println("\n GCD of " + a + " and " + b + " = " + GCD); LCM = (a * b) / GCD; System.out.println("\n LCM of " + a + " and " + b + " = " + LCM); } }
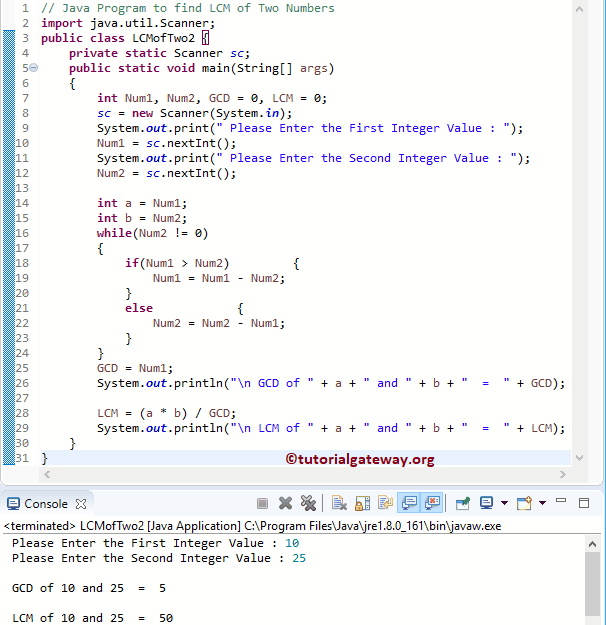
Java program to find LCM of Two Numbers using Recursive Function
This program calculates the Greatest Common Divisor by calling the HCFofTwo function recursively. Next, this program finds the Least Common Multiple from GCD.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int n1, n2, GCD = 0, LM = 0; sc = new Scanner(System.in); System.out.print("Please Enter the First Integer Value : "); n1 = sc.nextInt(); System.out.print("Please Enter the Second Integer Value : "); n2 = sc.nextInt(); GCD = HCFofTwo(n1, n2); System.out.println("\nGCD of " + n1 + " and " + n2 + " = " + GCD); LM = (n1 * n2) / GCD; System.out.println("LCM of " + n1 + " and " + n2 + " = " + LM); } public static int HCFofTwo(int n1, int n2) { if(n2 == 0) { return n1; } else { return HCFofTwo(n2, n1 % n2); } } }
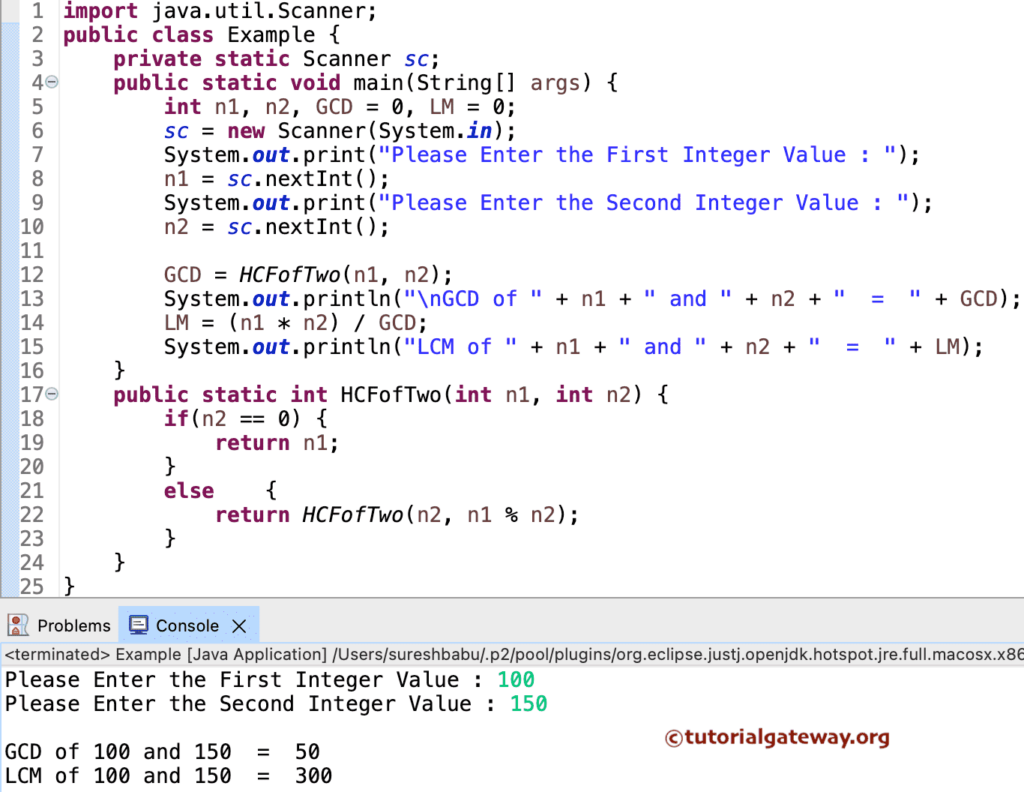