Write a Java program to find Last Digit of a Number with example.
Java Program to find Last Digit of a Number Example 1
This program allows the user to enter any number. Next, this Java program returns the Last Digit of a user-entered value.
// Java Program to find Last Digit of a Number import java.util.Scanner; public class LastDigit1 { private static Scanner sc; public static void main(String[] args) { int number, last_digit; sc = new Scanner(System.in); System.out.print(" Please Enter any Number that you wish : "); number = sc.nextInt(); last_digit = number % 10; System.out.println("\n The Last Digit of a Given Number " + number + " = " + last_digit); } }
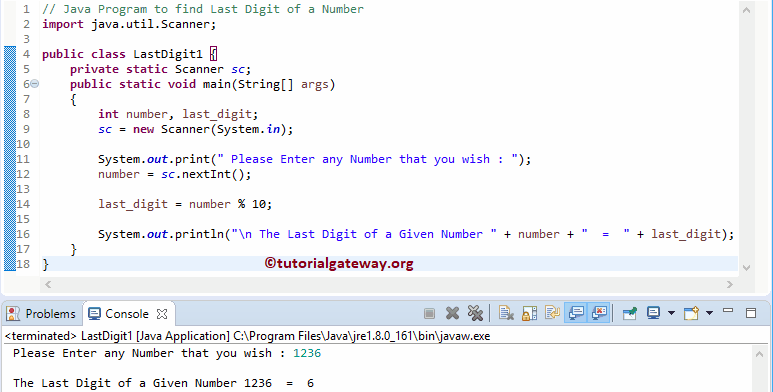
number = 1236
last_digit = number % 10
last_digit = 1236 % 10 = 6
Java Program for Last Digit of a Number Example 2
This Java program is the same as the above last digit example. But this time, we are creating a separate Java method to find the last Digit of the user entered value.
// Java Program to find Last Digit of a Number import java.util.Scanner; public class LastDigit2 { private static Scanner sc; public static void main(String[] args) { int number, last_digit; sc = new Scanner(System.in); System.out.print(" Please Enter any Number that you wish : "); number = sc.nextInt(); last_digit = lastDigit(number); System.out.println("\n The Last Digit of a Given Number " + number + " = " + last_digit); } public static int lastDigit(int num) { return num % 10; } }
Java last digit in a number output
Please Enter any Number that you wish : 4569
The Last Digit of a Given Number 4569 = 9