Write a Java Program to Find Cube of a Number with example.
Java Program to Find Cube of a Number Example 1
This Java program enables the user to enter an integer value. Then this Java program calculates cube of that number using Arithmetic Operator.
// Java Program to Find Cube of a Number import java.util.Scanner; public class CubeofNumber { private static Scanner sc; public static void main(String[] args) { int number, cube; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); number = sc.nextInt(); cube = number * number * number; System.out.println("\n The Cube of a Given Number " + number + " = " + cube); } }
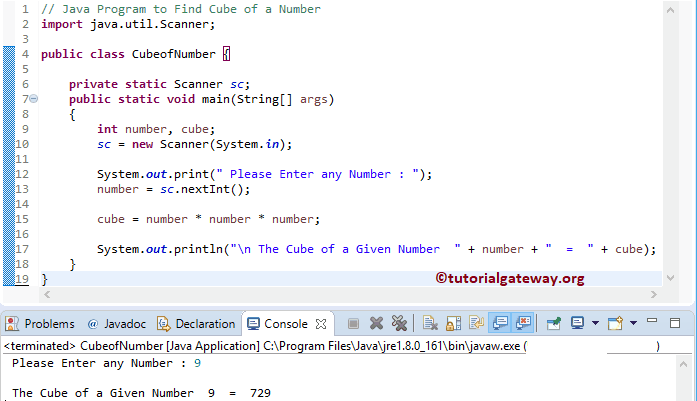
Java Program to Find Cube of a Number Example 2
This Java program is the same as above. But this time, we are creating a separate Java method to calculate the cube of that number.
// Java Program to Find Cube of a Number import java.util.Scanner; public class CubeofNumber1 { private static Scanner sc; public static void main(String[] args) { int number, cube; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); number = sc.nextInt(); cube = calCube(number); System.out.println("\n The Cube of a Given Number " + number + " = " + cube); } public static int calCube(int num) { return num * num * num; } }
Java cube of a number output
Please Enter any Number : 5
The Cube of a Given Number 5 = 125