Write a Java Program to Count the Total Occurrence of Character in a String with an example. In this total occurrence of a string character example, we used the While loop to iterate the calStr string from start to end.
Within the loop, we compare each calStr string letter (charAt()) with ch. If they are equal, we incremented the charCount. Finally, we are printing the charCount as an output.
import java.util.Scanner; public class CountAllCharOccurence1 { private static Scanner sc; public static void main(String[] args) { String calStr; char ch; int i = 0, charCount = 0; sc= new Scanner(System.in); System.out.print("\nPlease Enter String to Count all Char Occurrence = "); calStr = sc.nextLine(); System.out.print("\nEnter the Character to Find = "); ch = sc.next().charAt(0); while(i < calStr.length()) { if(calStr.charAt(i) == ch) { charCount++; } i++; } System.out.format("\nTotal Number of time %c has found = %d ", ch, charCount); } }
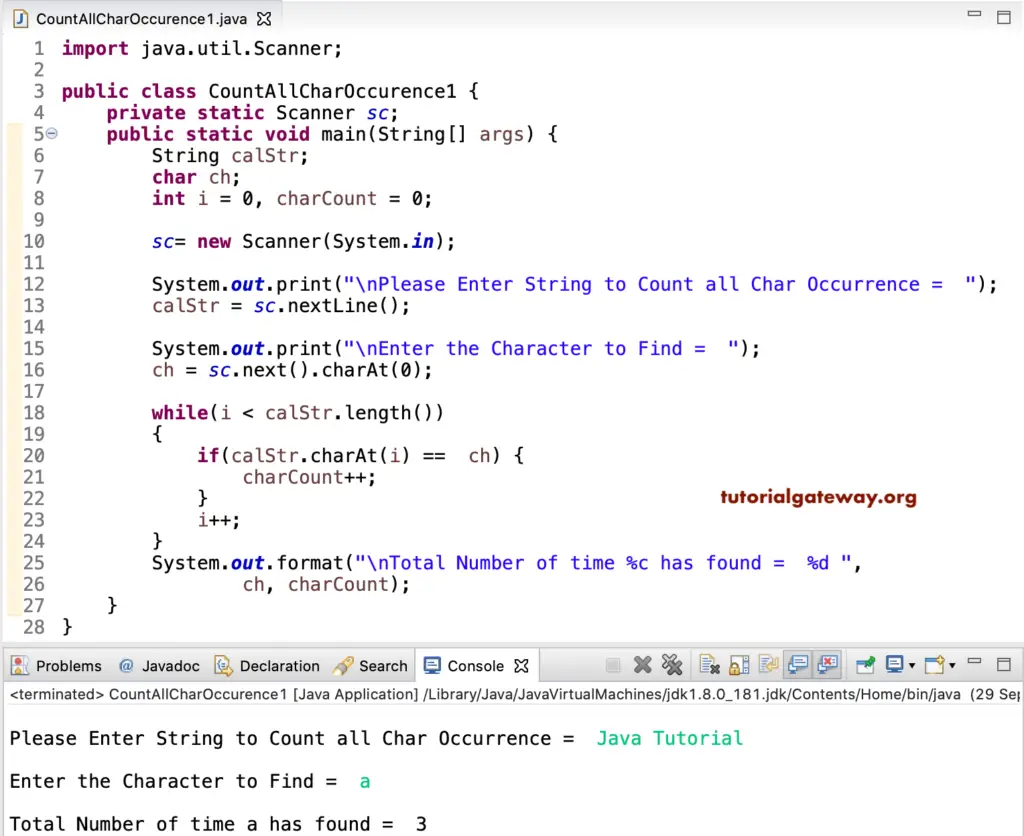
Java Program to Count Total Occurrence of Character in a String using for loop
This count total occurrence of a character example is the same as the above. In this Java example, we replaced the While loop with For Loop.
import java.util.Scanner; public class CntAllChOcc32 { private static Scanner sc; public static void main(String[] args) { String calStr; char ch; int i, charCount = 0; sc= new Scanner(System.in); System.out.print("\nPlease Enter String = "); calStr = sc.nextLine(); System.out.print("\nEnter the Character to Find = "); ch = sc.next().charAt(0); for(i = 0; i < calStr.length(); i++) { if(calStr.charAt(i) == ch) { charCount++; } } System.out.format("\nTotal Number of time %c has found = %d ", ch, charCount); } }
Please Enter String = hello world
Enter the Character to Find = l
Total Number of time l has found = 3
Java Program to Count Total Occurrence of Character in a String using Functions
Here, we created a CountAllCharOcc function that returns the character count.
import java.util.Scanner; public class CntAllChOcc3 { private static Scanner sc; public static void main(String[] args) { sc= new Scanner(System.in); System.out.print("\nPlease Enter Text = "); String calStr = sc.nextLine(); System.out.print("\nEnter the Letter to Find = "); char ch = sc.next().charAt(0); int charCount = CntAllCharOcc(calStr, ch); System.out.format("\nTotal Number of time %c has found = %d ", ch, charCount); } public static int CntAllCharOcc(String calStr, char ch) { int i, charCount = 0; for(i = 0; i < calStr.length(); i++) { if(calStr.charAt(i) == ch) { charCount++; } } return charCount; } }
Please Enter Text = tutorial gateway
Enter the letter to Find = t
Total Number of time t has found = 3
Please refer to the following string programs.
- Find All Occurrences of a Character in a String
- First Character Occurrence in a String
- Replace First Character Occurrence in a String
- Remove First Character Occurrence in a String
- Last Character Occurrence in a String
- Replace Last Character Occurrence in a String
- Remove the Last Character Occurrence in a String
- First and Last Character in a String
- Remove The First and Last Character in a String
- Maximum Occurring Character in a String
- Minimum Occurring Character in a String
- Frequency of each Character in a String
- Remove All Occurrences of a Character in a String