Write a Java program to count frequency of each element in an array using for loop.
package NumPrograms; import java.util.Arrays; import java.util.Scanner; public class ArrayCountOccrenceAll1 { private static Scanner sc; public static void main(String[] args) { int Size, i, j, count; sc = new Scanner(System.in); System.out.print("Please Enter Frequency Array size = "); Size = sc.nextInt(); int[] arr = new int[Size]; int[] freq = new int[Size]; Arrays.fill(freq, -1); System.out.format("Enter Frequency Array %d elements : ", Size); for(i = 0; i < Size; i++) { arr[i] = sc.nextInt(); } for(i = 0; i < arr.length; i++) { count = 1; for(j = i + 1; j < arr.length; j++) { if(arr[i] == arr[j]) { count++; freq[j] = 0; } } if(freq[i] != 0) { freq[i] = count; } } System.out.println("The Frequency of All the Elements in this Array "); for(i = 0; i < arr.length; i++) { if(freq[i] != 0) { System.out.println(arr[i] + " Occurs " + freq[i] + " Times."); } } } }
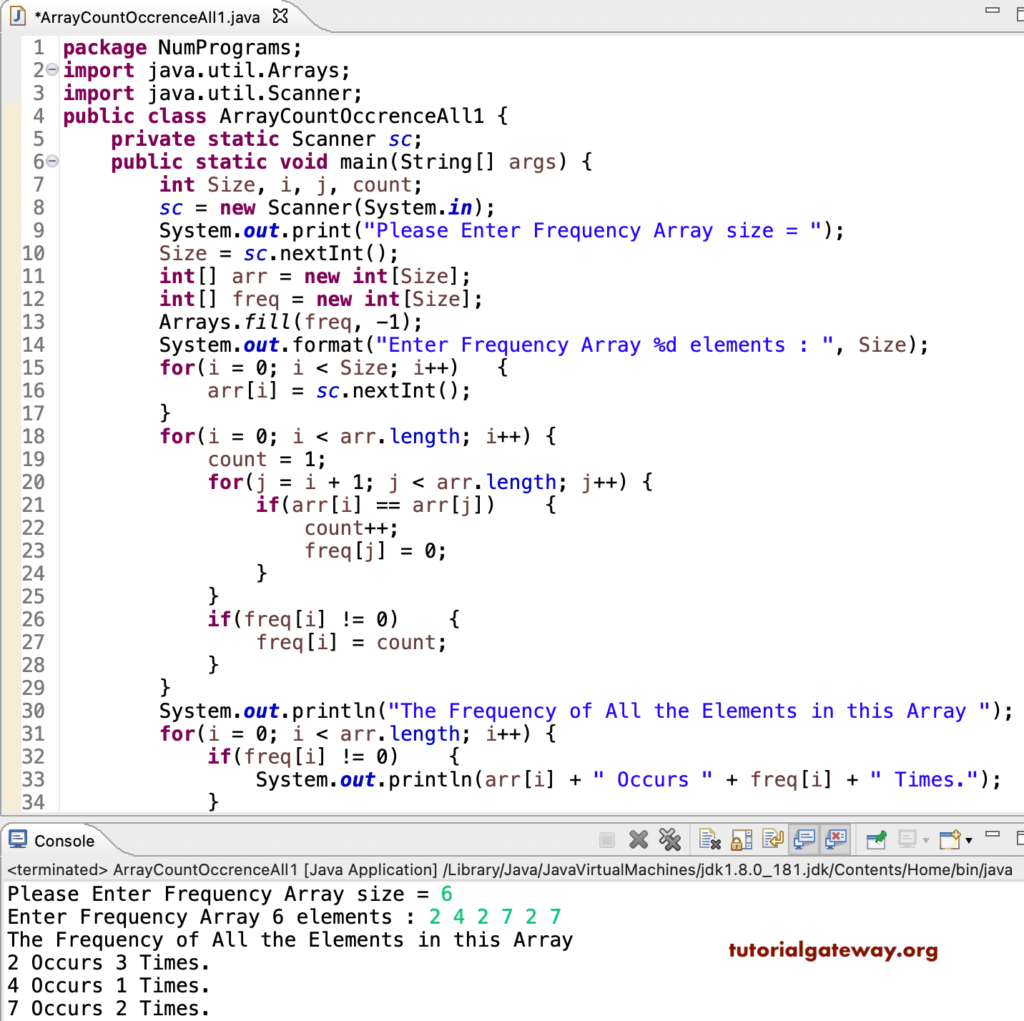
This program counts the frequency of each element or all elements in an array using HashMap.
package NumPrograms; import java.util.*; public class ArrayCountOccrenceAll2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter size = "); int Size = sc.nextInt(); int[] arr = new int[Size]; System.out.format("Enter %d elements : ", Size); for(int i = 0; i < Size; i++) { arr[i] = sc.nextInt(); } System.out.println("The Frequency of All the Elements in this Array "); arrFreq(arr); } static void arrFreq(int arr[]) { Map<Integer, Integer> countFreq = new HashMap<>(); for(int i : arr) { if(countFreq.containsKey(i)) { countFreq.put(i, countFreq.get(i) + 1); } else { countFreq.put(i, 1); } } for(Map.Entry<Integer, Integer> entry: countFreq.entrySet()) { System.out.println(entry.getKey() + " Occurs " + entry.getValue() + " Times."); } } }
Please Enter size = 7
Enter 7 elements : 22 9 22 5 9 11 14
The Frequency of All the Elements in this Array
5 Occurs 1 Times.
22 Occurs 2 Times.
9 Occurs 2 Times.
11 Occurs 1 Times.
14 Occurs 1 Times.