Write a Java Program to Count Alphabets Digits and Special Characters in a String with an example. In this Java count digits, alphabets, and special characters in a string example, we first used for loop to iterate aldisp_str. Inside the loop, to keep the code simple, we assigned (ch = aldisp_str.charAt(i)) each character to ch. Next, we used the Else If statement.
Within the first if condition (ch >= ‘a’ && ch <= ‘z’ || ch >= ‘A’ && ch <= ‘Z’ ), we check whether the character is an alphabet. If true, we increment the alph value. In the else if statement, we used (ch >= ‘0’ && ch <= ‘9’) to check whether the character is a digit, and if true, increment the digit value. Else, increment the special character value.
import java.util.Scanner; public class CountAlpDigiSpl1 { private static Scanner sc; public static void main(String[] args) { String aldisp_str; int i, alph, digi, spl; alph = digi = spl = 0; char ch; sc= new Scanner(System.in); System.out.print("\nPlease Enter Alpha Numeric Special String = "); aldisp_str = sc.nextLine(); for(i = 0; i < aldisp_str.length(); i++) { ch = aldisp_str.charAt(i); if(ch >= 'a' && ch <= 'z' || ch >= 'A' && ch <= 'Z' ) { alph++; } else if(ch >= '0' && ch <= '9') { digi++; } else { spl++; } } System.out.println("\nNumber of Alphabet Characters = " + alph); System.out.println("Number of Digit Characters = " + digi); System.out.println("Number of Special Characters = " + spl); } }
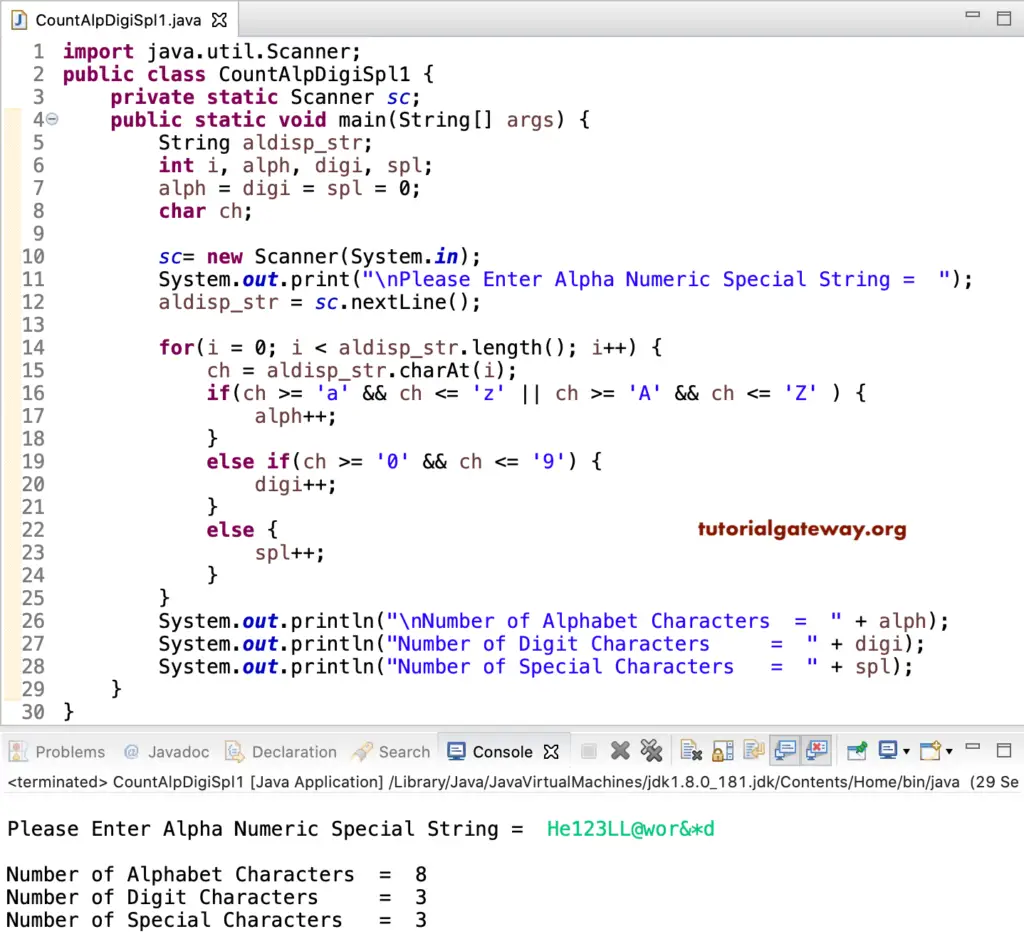
Java Program to Count Alphabets Digits and Special Characters in a String using While loop
import java.util.Scanner; public class CountAlpDigiSpl2 { private static Scanner sc; public static void main(String[] args) { String aldisp_str; int i, alph, digi, spl; i = alph = digi = spl = 0; char ch; sc= new Scanner(System.in); System.out.print("\nPlease Enter Alpha Numeric Special String = "); aldisp_str = sc.nextLine(); while(i < aldisp_str.length()) { ch = aldisp_str.charAt(i); if(ch >= 'a' && ch <= 'z' || ch >= 'A' && ch <= 'Z' ) { alph++; } else if(ch >= '0' && ch <= '9') { digi++; } else { spl++; } i++; } System.out.println("\nNumber of Alphabet Characters = " + alph); System.out.println("Number of Digit Characters = " + digi); System.out.println("Number of Special Characters = " + spl); } }
Please Enter Alpha Numeric Special String = 9In12&*belo 33j^%&*(?hi
Number of Alphabet Characters = 9
Number of Digit Characters = 5
Number of Special Characters = 9
In this Java count digits, alphabets, and special characters in a string example, we compare the ASCII values instead of comparing characters.
import java.util.Scanner; public class CountAlpDigiSpl3 { private static Scanner sc; public static void main(String[] args) { String aldisp_str; int i, alph, digi, spl; i = alph = digi = spl = 0; int asci; sc= new Scanner(System.in); System.out.print("\nPlease Enter Alpha Numeric Special String = "); aldisp_str = sc.nextLine(); for(i = 0; i < aldisp_str.length(); i++) { asci = aldisp_str.codePointAt(i); if(asci >= 48 && asci <= 57 ) { digi++; } else if(asci >= 65 && asci <= 90 || asci >= 97 && asci <= 122) { alph++; } else { spl++; } } System.out.println("\nNumber of Alphabet Characters = " + alph); System.out.println("Number of Digit Characters = " + digi); System.out.println("Number of Special Characters = " + spl); } }
Please Enter Alpha Numeric Special String = Ja@#va2020!world2.0
Number of Alphabet Characters = 9
Number of Digit Characters = 6
Number of Special Characters = 4
In Java, we have a built-in function isdigit (Character.isDigit(ch) to check the character is a digit or not. isAlphabetic function (Character.isAlphabetic(ch)) to check the character is a alphabet. If both those conditions are false, then the character is special.
import java.util.Scanner; public class CountAlpDigiSpl4 { private static Scanner sc; public static void main(String[] args) { String aldisp_str; int i, alph, digi, spl; alph = digi = spl = 0; char ch; sc= new Scanner(System.in); System.out.print("\nPlease Enter Alpha Numeric Special String = "); aldisp_str = sc.nextLine(); for(i = 0; i < aldisp_str.length(); i++) { ch = aldisp_str.charAt(i); if(Character.isDigit(ch)) { digi++; } else if(Character.isAlphabetic(ch)) { alph++; } else { spl++; } } System.out.println("\nNumber of Alphabet Characters = " + alph); System.out.println("Number of Digit Characters = " + digi); System.out.println("Number of Special Characters = " + spl); } }
Please Enter Alpha Numeric Special String = !@34*(Monday0?
Number of Alphabet Characters = 6
Number of Digit Characters = 3
Number of Special Characters = 5
This Java code to Count Alphabets Digits and Special Characters in a String is the same as the above. Here, we separated the Alphabets, Digits, and special character’s logic using Java functions.
import java.util.Scanner; public class CountAlpDigiSpl5 { private static Scanner sc; public static void main(String[] args) { String aldisp_str; sc= new Scanner(System.in); System.out.print("\nPlease Enter Alpha Numeric Special String = "); aldisp_str = sc.nextLine(); CountDigitsAlphabetsSpecials(aldisp_str); } public static void CountDigitsAlphabetsSpecials(String aldisp_str) { char ch; int alph, digi, spl; alph = digi = spl = 0; for(int i = 0; i < aldisp_str.length(); i++) { ch = aldisp_str.charAt(i); if(Character.isDigit(ch)) { digi++; } else if(Character.isAlphabetic(ch)) { alph++; } else { spl++; } } System.out.println("\nNumber of Alphabet Characters = " + alph); System.out.println("Number of Digit Characters = " + digi); System.out.println("Number of Special Characters = " + spl); } }
Please Enter Alpha Numeric Special String = Sep14<>?temb@r
Number of Alphabet Characters = 8
Number of Digit Characters = 2
Number of Special Characters = 4