This article shows how to write a Java program to convert string to long. We can convert string to long using the parseLong() function and the Long.valueOf() function.
In this Java example, we declared two text values. Next, we used the Long.parseLong() function to convert the declared string values to long. Remember, if you parse the text input, the parseLong() function throws an error.
public class StringToLong { public static void main(String[] args) { String s1 = "2233440550"; String s2 = "1099908973"; long l1 = Long.parseLong(s1); long l2 = Long.parseLong(s2); System.out.println("Long.parseLong() result = " + l1); System.out.println("Long.parseLong() result = " + l2); } }
Long.parseLong() result = 2233440550
Long.parseLong() result = 1099908973
Java Program to Convert String to Long using valueOf
This string to long example is the same as the above. However, we used the Long.valueOf() function to convert string values to long data type.
public class StringToLong { public static void main(String[] args) { String s3 = "99933322222"; String s4 = "11199739989"; long l3 = Long.valueOf(s3); long l4 = Long.valueOf(s4); System.out.println("Long.valueOf() result = " + l3); System.out.println("Long.valueOf() result = " + l4); } }
Long.valueOf() result = 99933322222
Long.valueOf() result = 11199739989
Java Program to Convert String to Long using parseLong
This program allows users to enter values. Next, we used both the Long.parseLong() function and Long.valueOf() function on that user given value.
import java.util.Scanner; public class StringToLong { private static Scanner sc; public static void main(String[] args) { String str; sc= new Scanner(System.in); System.out.println("\n Please Enter any long Value : "); str = sc.nextLine(); long l5 = Long.parseLong(str); long l6 = Long.valueOf(str); System.out.println("Long.parseLong() result = " + l5); System.out.println("Long.valueOf() result = " + l6); } }
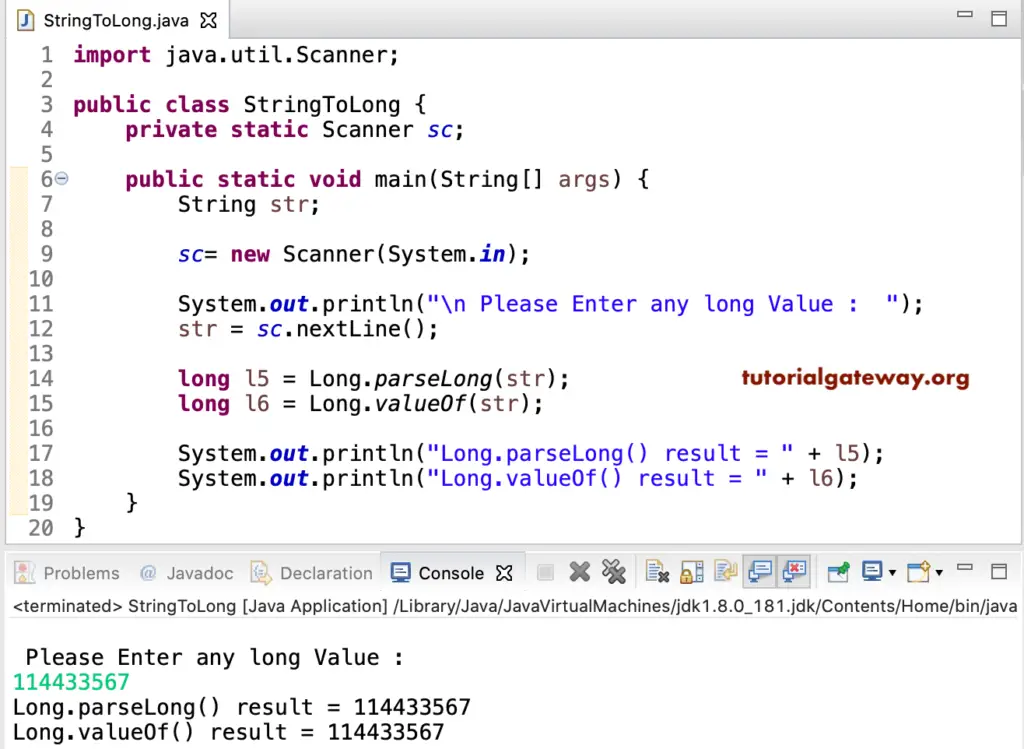