Write a Java program to check whether a character is Vowel or Consonant using if-else with an example. The If condition checks whether the user entered character is a, e, i, o, u, A, E, I, O, U. If it is True, it is a Vowel; other, its a consonant.
import java.util.Scanner; public class CharVowelorConsonant1 { private static Scanner sc; public static void main(String[] args) { char ch; sc= new Scanner(System.in); System.out.print("Please Enter any Character = "); ch = sc.next().charAt(0); if(ch == 'a' || ch == 'e' || ch == 'i' || ch <= 'o' || ch == 'u' || ch == 'A' || ch == 'E' || ch == 'I' || ch <= 'O' || ch == 'U') { System.out.println(ch + " is Vowel"); } else { System.out.println(ch + " is Consonant"); } } }
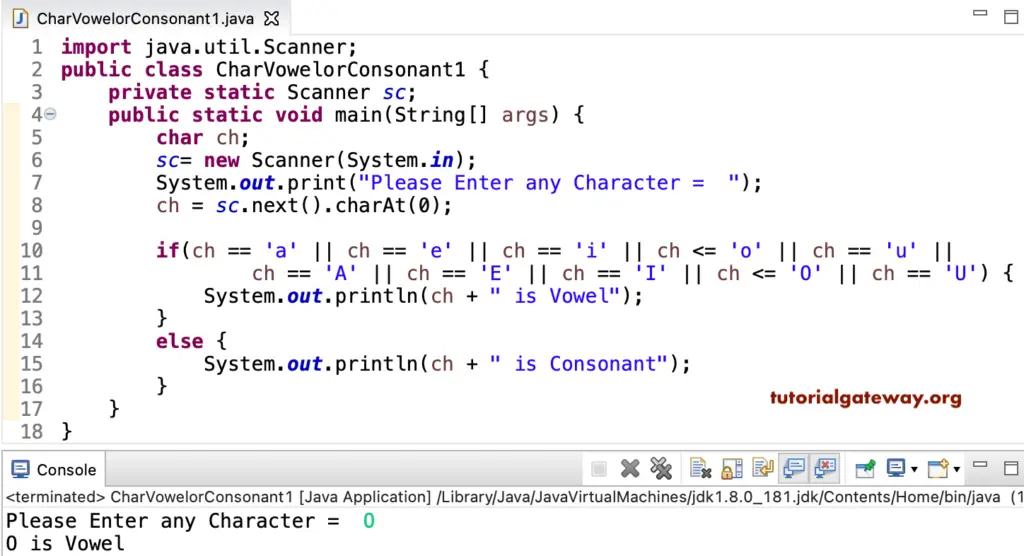
Java Program to Check Whether a Character is Vowel or Consonant using a Switch Case
Please refer to the If Else, Else If, Switch Case, and ASCII Codes to understand this Java example.
import java.util.Scanner; public class Example2 { private static Scanner sc; public static void main(String[] args) { char ch; sc= new Scanner(System.in); System.out.print("\nPlease Enter any Character = "); ch = sc.next().charAt(0); switch(ch) { case 'a': case 'e': case 'i': case 'o': case 'u': case 'A': case 'E': case 'I': case 'O': case 'U': System.out.println(ch + " is Vowel"); break; default: System.out.println(ch + " is Consonant"); } } }
Please Enter any Character = g
g is Consonant
Please Enter any Character = U
U is Vowel
In this Java Program to check whether the character is Vowel or Consonant example; first, we check whether the ASCII value of a given character equals 65, 69, 73, 79, 85, 97, 101, 105, 111, or 117. Because their ASCII codes belong to upper and lowercase vowels.
If it is true, it prints the Vowel statement. Next, we find whether the character is between 65 to 90 or 97 to 122 to check the consonant.
import java.util.Scanner; public class Example3 { private static Scanner sc; public static void main(String[] args) { char ch; sc= new Scanner(System.in); System.out.print("\nPlease Enter any Char = "); ch = sc.next().charAt(0); if(ch == 65 || ch == 69 || ch == 73 || ch == 79 || ch == 85 || ch == 97 || ch == 101 || ch == 105 || ch == 111 || ch == 117) { System.out.println(ch + " is Vowel"); } else if ((ch >= 65 && ch <= 90) || (ch >= 97 && ch <= 122)){ System.out.println(ch + " is Consonant"); } else { System.out.println("Please enter Valid Letter"); } } }
Please Enter any Char = k
k is Consonant
Please Enter any Char = 9
Please enter Valid Letter
Please Enter any Char = i
i is Vowel