Write a Java Program to Calculate Profit or Loss with example. If the actual amount is less than the Sales amount, it is a profitable product. Else if the actual amount is greater than the Sale amount, the product is in Loss — otherwise, no profit, no loss.
Java Program to Calculate Profit or Loss Example 1
This program allows the user to enter the actual amount and Sales amount. By using those two values, this Java program checks whether the product is in profit or Loss using Else If Statement.
TIP: Java Else If statement checks the first condition if it is TRUE, it executes the statements inside that block. If the condition is FALSE, it checks the Next one (Else If condition) and so on.
// Java Program to Calculate Profit or Loss import java.util.Scanner; public class ProfitorLoss { private static Scanner sc; public static void main(String[] args) { float saleAmount, unitPrice, amount; sc = new Scanner(System.in); System.out.print(" Please Enter the Actual Product Cost : "); unitPrice = sc.nextFloat(); System.out.print(" Please Enter the Selling Price (Market Price) : "); saleAmount = sc.nextFloat(); if(saleAmount > unitPrice ) { amount = saleAmount - unitPrice; System.out.println("\n Profit Amount = " + amount); } else if(unitPrice > saleAmount) { amount = unitPrice - saleAmount; System.out.println("\n Loss Amount = " + amount); } else { System.out.println("\n No Profit No Loss! "); } } }
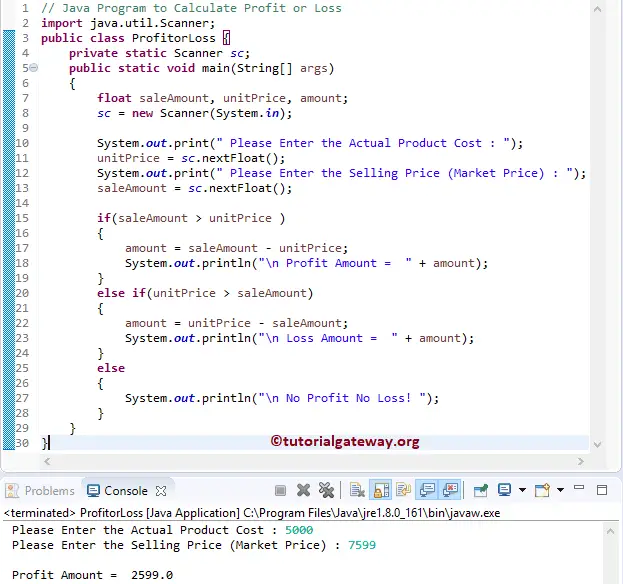
Let me try another value
Please Enter the Actual Product Cost : 5000
Please Enter the Selling Price (Market Price) : 3999
Loss Amount = 1001.0
another one
Please Enter the Actual Product Cost : 5000
Please Enter the Selling Price (Market Price) : 5000
No Profit No Loss!
Java Program to find Profit or Loss Example 2
This program is the same as the above profit loss example. But this time, we are creating a separate Java method to calculate the profit or loss.
// Java Program to Calculate Profit or Loss import java.util.Scanner; public class ProfitorLoss1 { private static Scanner sc; public static void main(String[] args) { float saleAmount, unitPrice; sc = new Scanner(System.in); System.out.print(" Please Enter the Actual Product Cost : "); unitPrice = sc.nextFloat(); System.out.print(" Please Enter the Selling Price (Market Price) : "); saleAmount = sc.nextFloat(); profitOrloss(saleAmount, unitPrice); } public static void profitOrloss(float saleAmount, float unitPrice) { float amount; if(saleAmount > unitPrice ) { amount = saleAmount - unitPrice; System.out.println("\n Profit Amount = " + amount); } else if(unitPrice > saleAmount) { amount = unitPrice - saleAmount; System.out.println("\n Loss Amount = " + amount); } else { System.out.println("\n No Profit No Loss! "); } } }
Java profit or loss output
Please Enter the Actual Product Cost : 2500
Please Enter the Selling Price (Market Price) : 1559
Loss Amount = 941.0