Write a Java Program to Calculate Compound Interest with an example. The formula behind Compound Interest calculation:
Future CI = Principal Amount * ( 1 + Rate of Interest )Number of years)
The above formula is used to calculate the Future because it contains both the Principal Amount and CI. To get the Compound Interest, use the below formula:
CI = Future CI – Principal Amount
Java Program to Calculate Compound Interest Example
This program allows the user to enter the Principal Amount, Interest Rate, and the total number of years. By using those values, this program finds the compound Interest using the above-specified formula.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { double PAmount, ROI, TimePeriod, FutureCI, CI; sc = new Scanner(System.in); System.out.print(" Please Enter the Principal Amount : "); PAmount = sc.nextDouble(); System.out.print(" Please Enter the Rate Of Interest : "); ROI = sc.nextDouble(); System.out.print(" Please Enter the Time Period in Years : "); TimePeriod = sc.nextDouble(); FutureCI = PAmount * (Math.pow(( 1 + ROI/100), TimePeriod)); CI = FutureCI - PAmount; System.out.println("\n The Future for Principal Amount " + PAmount + " is = " + FutureCI); System.out.println(" The Actual for Principal Amount " + PAmount + " is = " + CI); } }
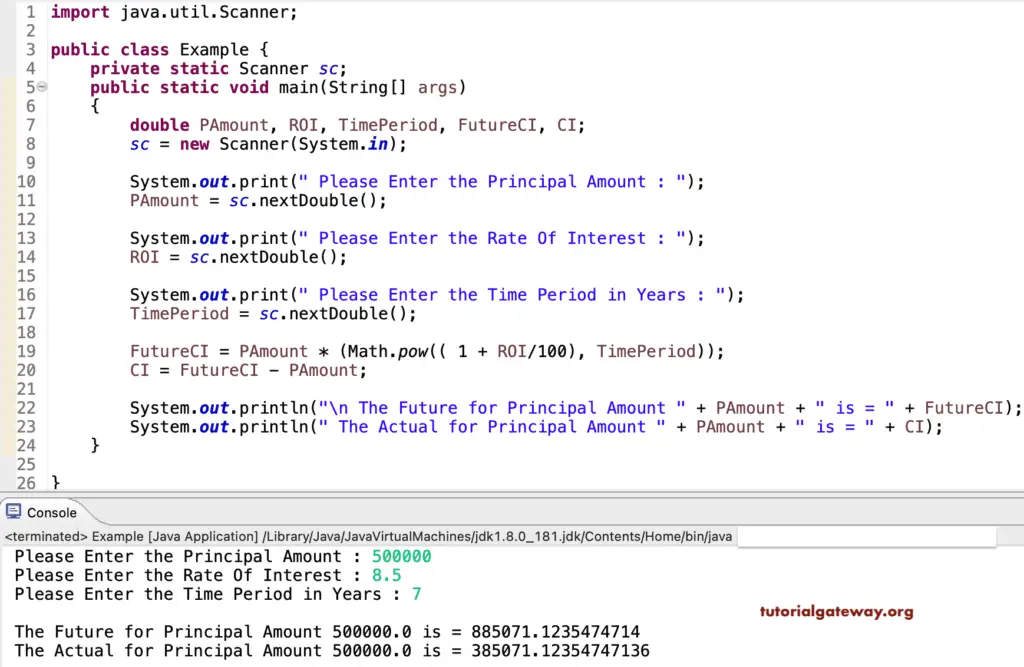
Java Program to Calculate Compound Interest using Functions
This program is the same as above. But this time, we are creating a separate method to calculate the compound interest.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { double PAmount, ROI, TimePeriod; sc = new Scanner(System.in); System.out.print(" Please Enter the Principal Amount : "); PAmount = sc.nextDouble(); System.out.print(" Please Enter the ROI : "); ROI = sc.nextDouble(); System.out.print(" Please Enter the Time Period in Years : "); TimePeriod = sc.nextDouble(); calCmpI(PAmount, ROI, TimePeriod); } public static void calCmpI(double PAmount, double ROI, double TimePeriod) { double FutureCI, CI; FutureCI = PAmount * (Math.pow(( 1 + ROI/100), TimePeriod)); CI = FutureCI - PAmount; System.out.println("\n Future for " + PAmount + " is = " + FutureCI); System.out.println(" Actual for " + PAmount + " is = " + CI); } }
Please Enter the Principal Amount : 1400000
Please Enter the ROI : 9.5
Please Enter the Time Period in Years : 7
Future for 1400000.0 is = 2642572.2488883743
Actual for 1400000.0 is = 1242572.2488883743