Write a program to add two Matrices with an example. Or write a Java program to add two multi-dimensional arrays using a for loop and print them in Matrix format.
A = [ a11 a12 a21 a22 ]
B = [ b11 b12 b21 b22 ]
A + B = [a11 + b11 a12 + b12 a21 + b21 a22 + b22 ]
Java program to add two Matrices using for loop
In this Java example, we declared two integer matrixes with random values. Next, we used the For Loop to iterate and add each row and column value of X to the Y matrix. Later, we used another for loop to print the final output.
public class addTwoMatrix { public static void main(String[] args) { int[][] x = {{10, 20, 30}, {40, 50, 60}, {70, 80, 90}}; int[][] y = {{ 5, 15, 25}, {35, 45, 55}, {65, 75, 85}}; int[][] sum = new int[3][3]; int i, j; for(i = 0; i < x.length; i++) { for(j = 0; j < x[0].length; j++) { sum[i][j] = x[i][j] + y[i][j]; } } System.out.println("------ The addition of two Matrices ------"); for(i = 0; i < x.length; i++) { for(j = 0; j < x[0].length; j++) { System.out.format("%d \t", sum[i][j]); } System.out.println(""); } } }
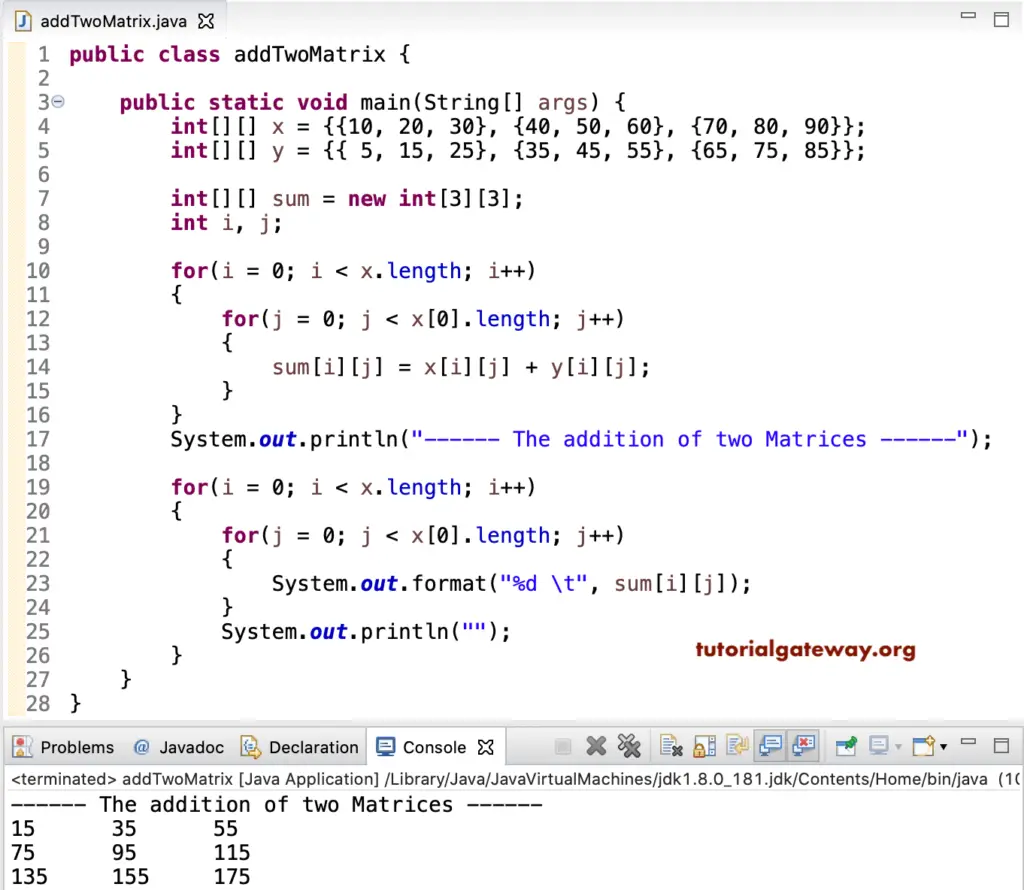
Java program to add two Matrices example 2
This Java example is the same as above. However, this Java code allows the user to enter the total number of rows and columns and then asks for the items. Please refer to the C Program to Sum Mats article to understand the iteration-wise program execution.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns; sc= new Scanner(System.in); System.out.println("\n Please Enter Rows and Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] arr1 = new int[rows][columns]; int[][] arr2 = new int[rows][columns]; System.out.println("\n Please Enter the First Mat Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr1[i][j] = sc.nextInt(); } } System.out.println("\n Please Enter the Second Mat Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr2[i][j] = sc.nextInt(); } } System.out.println("\n-----The Sum of two Matrixes----- "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { System.out.format("%d \t", (arr1[i][j] + arr2[i][j])); } System.out.println(""); } } }
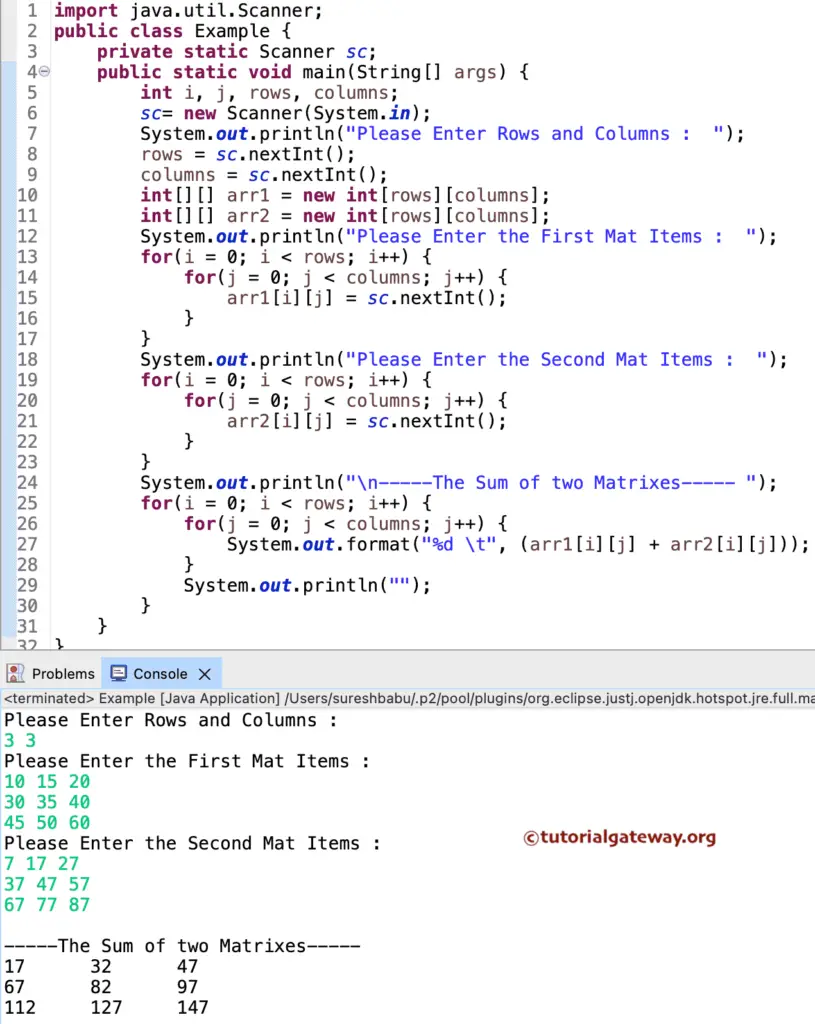