Write a Java program to add two binary numbers with an example. As we know, binary numbers are the combination of 1’s and 0’s. Thus, adding two means
- 0 + 0 = 0
- 0 + 1 = 1
- 1 + 0 = 1
- 1 + 1 = 10. Here, 1 will carry forward.
- 1 (carry forwarded number) + 1 + 1 = 11 and the 1 will carry forward.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.println("Enter First and Second Numbers = "); long b1 = sc.nextLong(); long b2 = sc.nextLong(); int i = 0, carry = 0; int[] sum = new int[10]; while(b1 != 0 || b2 != 0) { sum[i++] = (int)((b1 % 10 + b2 % 10 + carry) % 2); carry = (int)((b1 % 10 + b2 % 10 + carry) / 2); b1 /= 10; b2 /= 10; } if(carry != 0) { sum[i++] = carry; } --i; System.out.print("\nThe Total of the above Two = "); while(i >= 0) { System.out.print(sum[i--]); } System.out.println(); } }
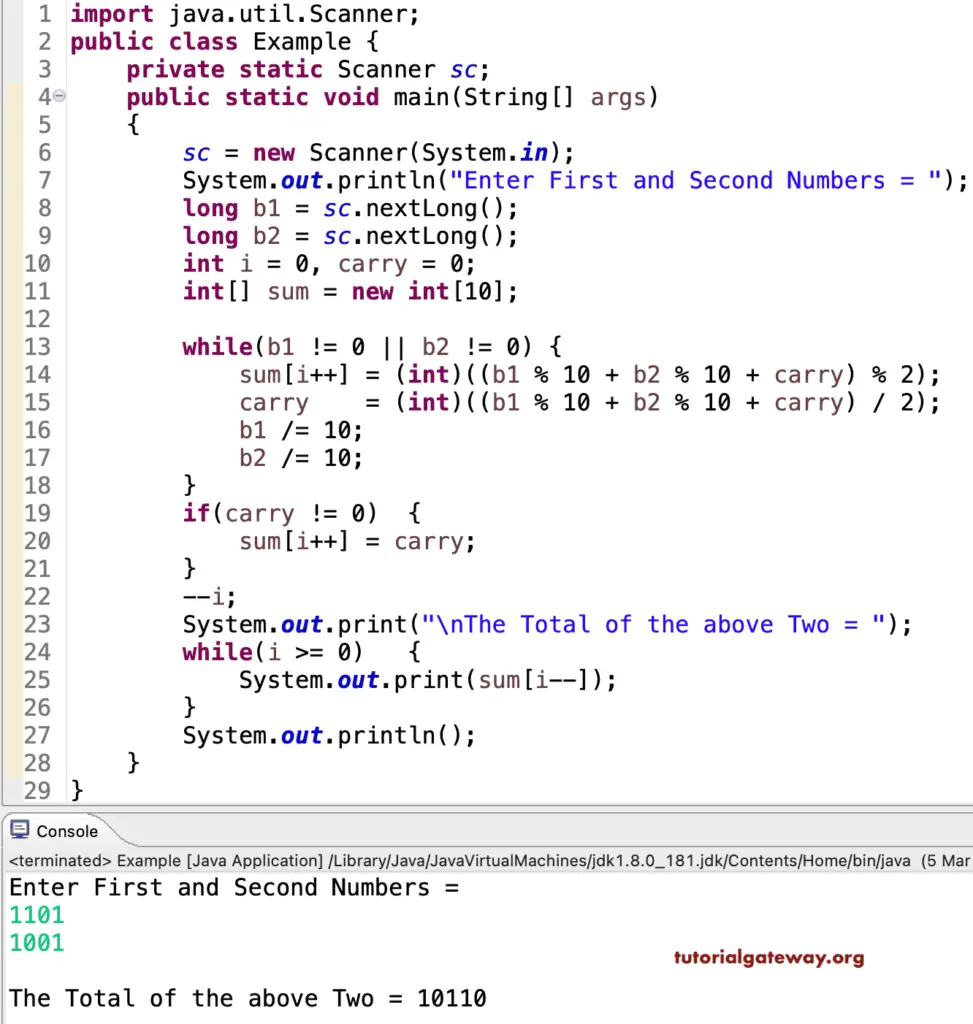
This Java program converts the binary to integer and adds two numbers. If the two of them are of string data type, we can use the Integer parseInt method to convert them to integers and add those two integer values. Next, we can convert them back to a binary number using the toBinaryString.
import java.util.Scanner; public class Example2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.println("Enter The First and Second Numbers = "); String b1 = sc.nextLine(); String b2 = sc.nextLine(); int num1 = Integer.parseInt(b1, 2); int num2 = Integer.parseInt(b2, 2); int output = num1 + num2; System.out.print("\nThe Sum = "); System.out.print(Integer.toBinaryString(output)); } }
Enter The First and Second Numbers =
101010
111111
The Sum = 1101001
This program helps to add two binary numbers using the for loop. Please refer to Java programs.
import java.util.Scanner; public class Example3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.println("Enter The First and Second Numbers = "); long b1 = sc.nextLong(); long b2 = sc.nextLong(); int i, carry = 0; int[] sum = new int[10]; for(i = 0; b1 != 0 || b2 != 0; b1 /= 10, b2 /= 10) { sum[i++] = (int)((b1 % 10 + b2 % 10 + carry) % 2); carry = (int)((b1 % 10 + b2 % 10 + carry) / 2); } if(carry != 0) { sum[i++] = carry; } --i; System.out.print("\nThe Sum = "); while(i >= 0) { System.out.print(sum[i--]); } System.out.println(); } }
Enter The First and Second Numbers =
110101
010111
The Sum = 1001100