Write a Java Program for Strong Number using While Loop, For Loop, and Functions. We also show how to print Strong Numbers between 1 to n. If the sum of the factorial of each digit is equal to the given number, then it is called a Strong Number.
Java Program for Strong Number using While Loop
This program for a strong number in java allows the user to enter any integer value. Next, it checks whether the given number is strong or not using While Loop.
// Java program for Strong Number import java.util.Scanner; public class StrongNumber1 { private static Scanner sc; public static void main(String[] args) { int Number, Temp, Reminder, Sum = 0, i, Factorial; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); Number = sc.nextInt(); Temp = Number; while( Temp > 0) { Factorial = 1; i = 1; Reminder = Temp % 10; while (i <= Reminder) { Factorial = Factorial * i; i++; } System.out.println(" The Factorial of " + Reminder + " = " + Factorial); Sum = Sum + Factorial; Temp = Temp /10; } System.out.println(" The Sum of the Factorials of a Given Number " + Number + " = " + Sum); if ( Number == Sum ) { System.out.println("\n " + Number + " is a Strong Number"); } else { System.out.println("\n " + Number + " is Not a Strong Number"); } } }
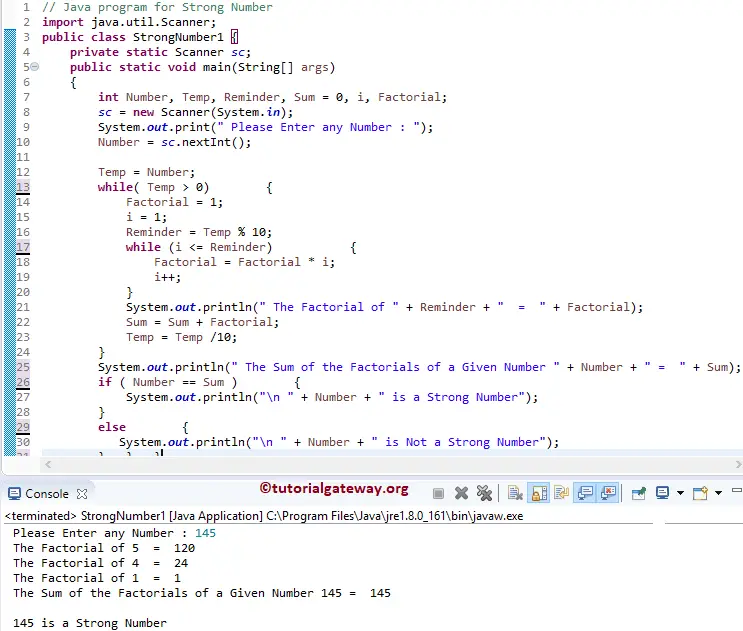
User Entered value for this Java strong number program: Number = 145 and Sum = 0
Temp = Number = 145
First Iteration: while( Temp > 0)
Reminder = Temp % 10 => 145 % 10
Reminder = 5
Next, it enters into the Inner While loop. Here, it calculates the factorial of 5 = 120. Please refer Factorial Program article in Java.
Sum = Sum +120 => 0 + 120
Sum = 120
Temp = Temp /10 => 145 /10
Temp = 14
Second Iteration: while( 14 > 0)
From the first Iteration, the values of both Temp and Sum has changed as Temp = 14 and Sum = 120
Reminder = 14 % 10 = 4
Next, it calculates the factorial of 4, and that is 24.
Sum = 120 + 24
Sum = 144
Temp = 14 /10
Temp = 1
Third Iteration: while( 1 > 0)
From the Second Iteration: Temp = 1 and Sum = 144
Reminder = 1 % 10 = 0
Next, the factorial of 1 is 1
Sum = 144 + 1
Sum = 145
Temp = 1 / 10
Temp = 0
Here, Temp = 0. So, the condition inside the While Loop fail
if ( Number == Sum ) => if(145 = 145) – Condition is True. So, the given number is a Strong Number
Java Program for Strong Number using For Loop
This Java program strong number allows the user to enter any integer value. Next, it checks whether the given number is strong or not using Java For Loop.
We just replaced the While loop in the above Java strong number example with the For loop. If you don’t understand the For Loop, please refer: FOR LOOP.
import java.util.Scanner; public class Example2 { private static Scanner sc; public static void main(String[] args) { int Number, Temp, Reminder, Sum = 0, i, Factorial; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); Number = sc.nextInt(); for(Temp = Number; Temp > 0; Temp = Temp /10) { Factorial = 1; Reminder = Temp % 10; for(i = 1; i <= Reminder; i++) { Factorial = Factorial * i; } System.out.println(" The Factorial of " + Reminder + " = " + Factorial); Sum = Sum + Factorial; } System.out.println(" The Sum of the Factorials " + Number + " = " + Sum); if ( Number == Sum ) { System.out.println("\n " + Number + " is a Strong Number"); } else { System.out.println("\n " + Number + " is Not"); } } }
Please Enter any Number : 40585
The Factorial of 5 = 120
The Factorial of 8 = 40320
The Factorial of 5 = 120
The Factorial of 0 = 1
The Factorial of 4 = 24
The Sum of the Factorials 40585 = 40585
40585 is a Strong Number
Java Program for Strong Number using Method
This program for a strong number is the same as the first example. But we separated the Java strong number logic and placed it in a separate method.
import java.util.Scanner; public class Example3 { private static Scanner sc; public static void main(String[] args) { int Number, Temp, Reminder, Sum = 0, Factorial; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); Number = sc.nextInt(); for(Temp = Number; Temp > 0; Temp = Temp /10) { Factorial = 1; Reminder = Temp % 10; // Calling Calculate_factorial Function Factorial = Factorial(Reminder); System.out.println(" The Factorial of " + Reminder + " = " + Factorial); Sum = Sum + Factorial; } System.out.println(" The Sum of the Factorials of a Given Number " + Number + " = " + Sum); if ( Number == Sum ) { System.out.println("\n " + Number + " is a Strong Number"); } else { System.out.println("\n " + Number + " is Not"); } } public static int Factorial(int num) { if (num == 0 || num == 1) return 1; else return num * Factorial (num -1); } }
Please Enter any Number : 145
The Factorial of 5 = 120
The Factorial of 4 = 24
The Factorial of 1 = 1
The Sum of the Factorials of a Given Number 145 = 145
145 is a Strong Number
Java Program to Print Strong Numbers from 1 to N
This strong number program accepts the Minimum and maximum values from the user. Next, it prints the list of strong numbers between the minimum and maximum value.
import java.util.Scanner; public class PrintStrongNumbers1 { private static Scanner sc; public static void main(String[] args) { int Number, minimum, maximum, Temp, Sum = 0; sc = new Scanner(System.in); System.out.print(" Please Enter the Minimum value : "); minimum = sc.nextInt(); System.out.print(" Please Enter the Maximum value : "); maximum = sc.nextInt(); for(Number = minimum; Number <= maximum; Number++) { Temp = Number; Sum = StNum(Temp); if (Number == Sum ) { System.out.println(" " + Number + " is a Strong Number"); } } } public static int Factorial(int num) { if (num == 0 || num == 1) return 1; else return num * Factorial (num -1); } public static int StNum(int num) { int Temp, Reminder, Sum = 0, Factorial; for(Temp = num; Temp > 0; Temp = Temp /10) { Factorial = 1; Reminder = Temp % 10; Factorial = Factorial(Reminder); Sum = Sum + Factorial; } return Sum; } }
Java Strong Numbers from 1 to N output
Please Enter the Minimum value : 10
Please Enter the Maximum value : 100000
145 is a Strong Number
40585 is a Strong Number