Write a Java program for bubble sort in descending order. This Java example uses nested for loop to perform bubble sort on the array to sort them in descending order.
package Remaining; import java.util.Scanner; public class BubbleSortDesc1 { private static Scanner sc; public static void main(String[] args) { int i, j, Size, temp; sc = new Scanner(System.in); System.out.print("Enter the Bubble Sort Array size = "); Size = sc.nextInt(); int[] arr = new int[Size]; System.out.format("Enter Bubble Sort Array %d elements = ", Size); for(i = 0; i < Size; i++) { arr[i] = sc.nextInt(); } for(i = 0; i < arr.length; i++) { for(j = 0; j < arr.length - i - 1; j++) { if(arr[j] < arr[j + 1]) { temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } System.out.println("Bubble Sort to Sort Integers in Descending Order"); for(i = 0; i < arr.length; i++) { System.out.println(arr[i]); } } }
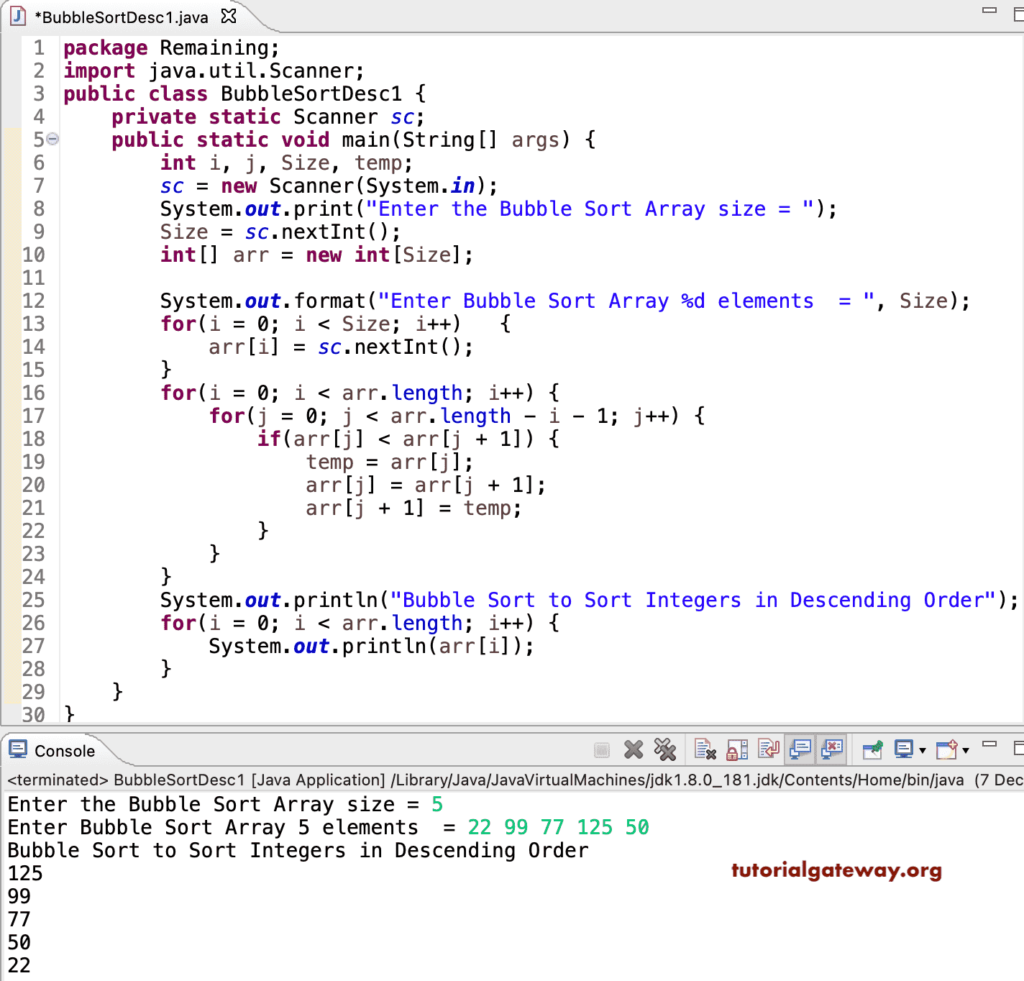
Java program to perform bubble sort on arrays in descending order using a while loop.
package Remaining; import java.util.Scanner; public class BubSrtDesc2 { private static Scanner sc; public static void main(String[] args) { int i, j, Size, temp; sc = new Scanner(System.in); System.out.print("Enter the size = "); Size = sc.nextInt(); int[] arr = new int[Size]; System.out.format("Enter %d elements = ", Size); i = 0; while(i < Size) { arr[i] = sc.nextInt(); i++; } i = 0; while(i < arr.length) { j = 0; while(j < arr.length - i - 1) { if(arr[j] < arr[j + 1]) { temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } j++; } i++; } System.out.println("Result"); i = 0; while(i < arr.length) { System.out.println(arr[i]); i++; } } }
Enter the size = 9
Enter 9 elements = 22 99 55 77 13 88 125 66 30
Result
125
99
88
77
66
55
30
22
13