Write a C Program to Remove First Occurrence of a Character in a String with example.
C Program to Remove First Occurrence of a Character in a String Example 1
This C program allows the user to enter a string (or character array), and a character value. Next, it will search and remove the first occurrence of a character inside a string.
/* C Program to Remove First Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i, len; i = 0; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Remove : "); scanf("%c", &ch); len = strlen(str); while(i < len && str[i] != ch) { i++; } while(i < len) { str[i] = str[i + 1]; i++; } printf("\n The Final String after Removing First occurrence of '%c' = %s ", ch, str); return 0; }
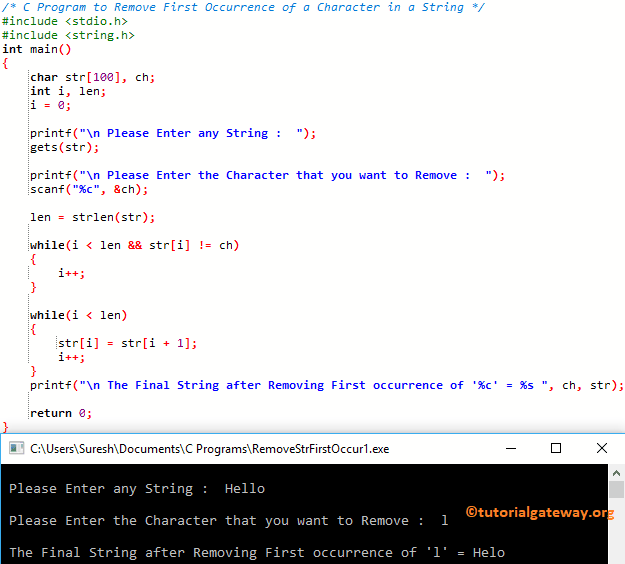
User entered values in this C Program
str[] = Hello
ch = l
While Loop First Iteration: while(i < len && str[i] != ch)
=> while(0 < 5 && H != l) – Condition is True
i will be incremented
Second Iteration: while(1 < 5 && e != l) – Condition is True
i will increment
Third Iteration: while(2 < 5 && l != l)
Condition is False. So, it will exit from the While Loop. Remember, i value is 2
i will increment
Next, the compiler will enter into the second while loop
Second While Loop First Iteration: while(i < len)
=> while(2 < 5) – Condition is True
str[i] = str[i + 1]
str[2] = str[3]
str[2] = l
i value incremented
Second Iteration: while(3 < 5)
str[3] = str[4]
str[3] = o
i will increment
Third Iteration: while(4 < 5)
str[4] = str[5]
str[4] = ‘\0’
i will increment
Fourth Iteration: while(5 < 5)
Condition Fails. So, the compiler will exit from the While Loop
Next, we used the printf statement to print the final string.
printf("\n The Final String after Removing First occurrence of '%c' = %s ", ch, str);
Program to Remove First Occurrence of a Character in a String Example 2
This program to remove first character occurrence is the same as above. Here, we just replaced the While Loop with For Loop.
/* C Program to Remove First Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i, len; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Remove : "); scanf("%c", &ch); len = strlen(str); for(i = 0; i < len && str[i] != ch; i++); while(i < len) { str[i] = str[i + 1]; i++; } printf("\n The Final String after Removing First occurrence of '%c' = %s ", ch, str); return 0; }
Please Enter any String : tutorial gateway
Please Enter the Character that you want to Remove : a
The Final String after Removing First occurrence of 'a' = tutoril gateway
Program to Remove First Occurrence of a Character in a String Example 3
This program to remove the first character occurrence is the same as the first example. But this time, we used the Functions concept to separate the logic.
/* C Program to Remove First Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> void Remove_FirstOccurrence(char *str, char ch); int main() { char str[100], ch; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Remove : "); scanf("%c", &ch); Remove_FirstOccurrence(str, ch); printf("\n The Final String after Removing First occurrence of '%c' = %s ", ch, str); return 0; } void Remove_FirstOccurrence(char *str, char ch) { int i, len; len = strlen(str); for(i = 0; i < len && str[i] != ch; i++); while(i < len) { str[i] = str[i + 1]; i++; } }
Please Enter any String : c programming
Please Enter the Character that you want to Remove : g
The Final String after Removing First occurrence of 'g' = c proramming