Write a C program to print right triangle characters pattern or alphabets pattern using for loop.
#include <stdio.h> int main() { int rows; printf("Enter Right Triangle of Characters Rows = "); scanf("%d", &rows); printf("Right Angled Triangle of Characters Pattern\n"); int alphabet = 65; for (int i = 1; i <= rows; i++) { for (int j = 0; j <= (2 * i - 2); j++) { printf("%c ", alphabet + j); } printf("\n"); } }
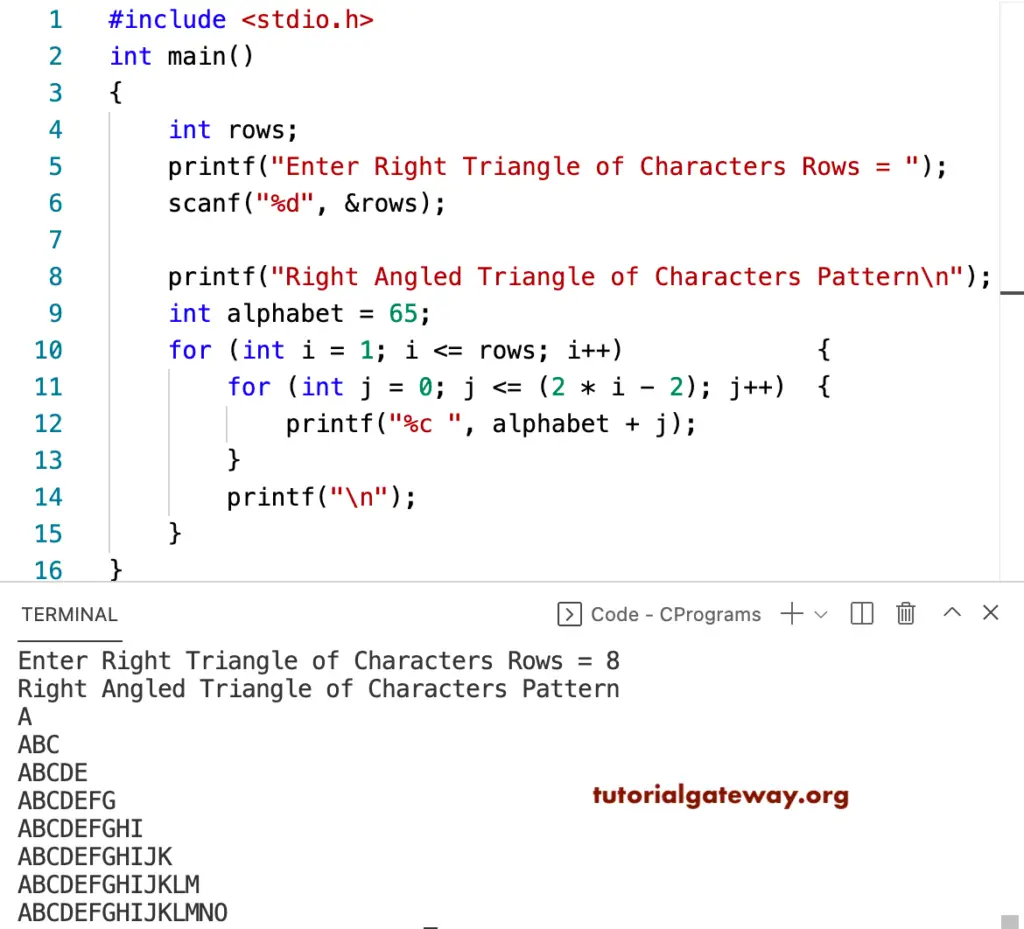
This C program prints the right angled triangle pattern of characters using a while loop.
#include <stdio.h> int main() { int i, j, alphabet, rows; printf("Enter Right Triangle of Characters Rows = "); scanf("%d", &rows); printf("Right Angled Triangle of Characters Pattern\n"); alphabet = 65; i = 1; while (i <= rows) { j = 0; while (j <= (2 * i - 2)) { printf("%c ", alphabet + j); j++; } printf("\n"); i++; } }
Enter Right Triangle of Characters Rows = 14
Right Angled Triangle of Characters Pattern
A
A B C
A B C D E
A B C D E F G
A B C D E F G H I
A B C D E F G H I J K
A B C D E F G H I J K L M
A B C D E F G H I J K L M N O
A B C D E F G H I J K L M N O P Q
A B C D E F G H I J K L M N O P Q R S
A B C D E F G H I J K L M N O P Q R S T U
A B C D E F G H I J K L M N O P Q R S T U V W
A B C D E F G H I J K L M N O P Q R S T U V W X Y
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [
This C example uses the do while loop to print the right angled triangle characters pattern.
#include <stdio.h> int main() { int i, j, alphabet, rows; printf("Enter Right Triangle of Characters Rows = "); scanf("%d", &rows); printf("Right Angled Triangle of Characters Pattern\n"); alphabet = 65; i = 1; do { j = 0; do { printf("%c ", alphabet + j); } while (++j <= (2 * i - 2)); printf("\n"); } while (++i <= rows); }
Enter Right Triangle of Characters Rows = 17
Right Angled Triangle of Characters Pattern
A
A B C
A B C D E
A B C D E F G
A B C D E F G H I
A B C D E F G H I J K
A B C D E F G H I J K L M
A B C D E F G H I J K L M N O
A B C D E F G H I J K L M N O P Q
A B C D E F G H I J K L M N O P Q R S
A B C D E F G H I J K L M N O P Q R S T U
A B C D E F G H I J K L M N O P Q R S T U V W
A B C D E F G H I J K L M N O P Q R S T U V W X Y
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [ \ ]
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [ \ ] ^ _
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [ \ ] ^ _ ` a