How to write a C Program to Print Prime Numbers from 1 to 100 or Minimum to a maximum or within a range and calculate the sum using For Loop and While Loop.
C Program to Print Prime Numbers from 1 to 100 Using While Loop
In this C program to return prime numbers from 1 to 100, we used the nested while loop along with multiple if statements to get the output.
If you don’t understand the While, please refer to WHILE LOOP
#include <stdio.h> int main() { int i, a = 1, count; while(a <= 100) { count = 0; i = 2; while(i <= a/2) { if(a%i == 0) { count++; break; } i++; } if(count == 0 && a != 1 ) { printf(" %d ", a); } a++; } return 0; }
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
It is the same as above, but we allows the user to choose the min and max value to print in between them.
#include <stdio.h> int main() { int i, Number, count, Minimum, Maximum; printf("\n Please Enter the Minimum & Maximum Values\n"); scanf("%d %d", &Minimum, &Maximum); Number = Minimum; printf("Prime Numbers Between %d and %d are:\n", Minimum, Maximum); while(Number <= Maximum) { count = 0; i = 2; while(i <= Number/2) { if(Number%i == 0) { count++; break; } i++; } if(count == 0 && Number != 1 ) { printf(" %d ", Number); } Number++; } return 0; }
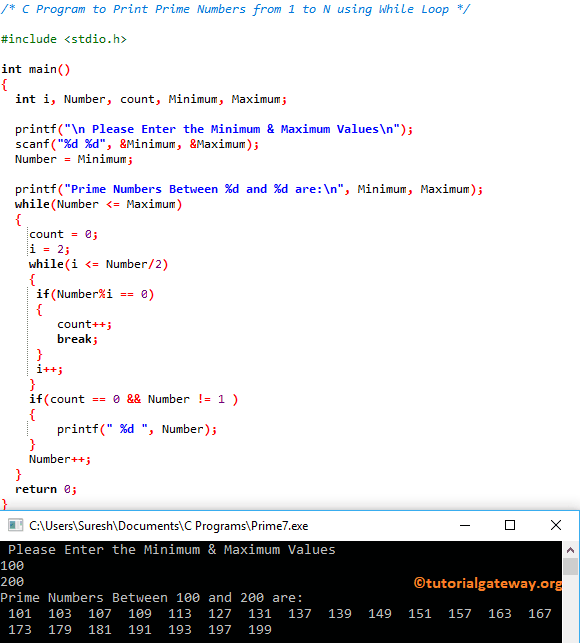
C Program to Print Prime Numbers from 1 to 100 Using For Loop
In this program, the first For Loop will make sure that the value is between them.
#include <stdio.h> int main() { int i, N, count; for(N = 1; N <= 100; N++) { count = 0; for (i = 2; i <= N/2; i++) { if(N%i == 0) { count++; break; } } if(count == 0 && N != 1 ) { printf(" %d ", N); } } return 0; }
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
TIP: We already explained the logic to check whether the given is Prime or not article in C language.
Instead of printing fixed values, you can allow the user to decide the minimum and maximum values. This program allows the user to enter Minimum and Maximum values.
Next, this program prints prime numbers between Minimum and Maximum values using For Loop.
#include <stdio.h> int main() { int i, val, count, Minimum, Maximum; printf("\n Please Enter the Minimum & Maximum Values\n"); scanf("%d %d", &Minimum, &Maximum); printf("Between %d and %d are:\n", Minimum, Maximum); for(val = Minimum; val <= Maximum; val++) { count = 0; for (i = 2; i <= val/2; i++) { if(val%i == 0) { count++; break; } } if(count == 0 && val != 1 ) { printf(" %d ", val); } } return 0; }
Please Enter the Minimum & Maximum Values
20
150
Between 20 and 150 are:
23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97 101 103 107 109 113 127 131 137 139 149
Sum of Prime Numbers from 1 to 100 using for loop
This Python program finds the prime numbers between 1 and 100 using for loop, and it’s going to adds them to calculate the sum.
#include <stdio.h> int main() { int i, Num, count, Sum = 0; for(Num = 1; Num <= 100; Num++) { count = 0; for (i = 2; i <= Num/2; i++) { if(Num%i == 0) { count++; break; } } if(count == 0 && Num != 1 ) { Sum = Sum + Num; } } printf(" Sum between 1 to 100 = %d", Sum); return 0; }
Sum between 1 to 100 = 1060
Instead of adding first 1 to 100, you can allow the user to decide the minimum and maximum values. This code allows the user to enter Minimum and Maximum values. Next, this C program finds the sum of prime numbers between Minimum and Maximum values using the For.
#include <stdio.h> int main() { int i, Num, count, Sum = 0, Minimum, Maximum; printf("\n Please Enter the Minimum & Maximum Values\n"); scanf("%d %d", &Minimum, &Maximum); for(Num = Minimum; Num <= Maximum; Num++) { count = 0; for (i = 2; i <= Num/2; i++) { if(Num%i == 0) { count++; break; } } if(count == 0 && Num != 1 ) { Sum = Sum + Num; } } printf(" Sum between %d and %d = %d", Minimum, Maximum, Sum); return 0; }
Please Enter the Minimum & Maximum Values
100
200
Sum between 100 and 200 = 3167
Comments are closed.