How to write a C Program to Print Natural Numbers from 1 to N using For Loop and While Loop?.
C Program to Print Natural Numbers from 1 to N using For Loop
This program allows the user to enter any integer value. Using For loop, we will print the list of natural numbers from 1 to user-entered value.
/* C Program to Print Natural Numbers from 1 to N using For Loop */ #include<stdio.h> int main() { int Number, i; printf("\n Please Enter any Integer Value : "); scanf("%d", &Number); printf("\n List of Natural Numbers from 1 to %d are \n", Number); for(i = 1; i <= Number; i++) { printf(" %d \t", i); } return 0; }
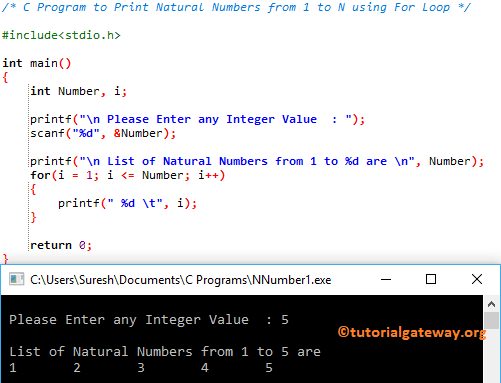
Within this C Program to display Natural Numbers from 1 to N example,
- The first printf statement will ask the user to enter an integer value, and the scanf statement will assign the user entered value to a Number variable.
- Next, we used For Loop to iterate between 1 and user-entered value. If you don’t know the For Loop, then please refer For loop in C Programming article for further reference.
- Within the For loop, we are printing the i values
- In the above C Programming example, user-entered value is five it means, 1 2 3 4 5
C Program to Print Natural Numbers from 1 to 100 using While Loop
This program is the same as above. We just replaced the For Loop with While Loop.
/* C Program to Print Natural Numbers from 1 to N using While Loop */ #include<stdio.h> int main() { int Number, i = 1; printf("\n Please Enter any Integer Value : "); scanf("%d", &Number); printf("\n List of Natural Numbers from 1 to %d are \n", Number); while(i <= Number) { printf(" %d \t", i); i++; } return 0; }
Please Enter any Integer Value : 15
List of Natural Numbers from 1 to 15 are
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
C Program to Print Natural Numbers within a Range
Instead of printing natural numbers from 1 to n, this program allows the user to enter both the Minimum and maximum value. Next, this C program prints natural numbers from Minimum to Maximum value.
/* C Program to Print Natural Numbers within a Range */ #include<stdio.h> int main() { int i, Starting_Value, End_Value; printf("\n Please Enter the Starting Value : "); scanf("%d", &Starting_Value); printf("\n Please Enter the End Value : "); scanf("%d", &End_Value); printf("\n List of Natural Numbers from %d to %d are \n", Starting_Value, End_Value); for(i = Starting_Value; i <= End_Value; i++) { printf(" %d \t", i); } return 0; }
Please Enter the Starting Value : 5
Please Enter the End Value : 45
List of Natural Numbers from 5 to 45 are
5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45