This article will show how to write a C Program to Print multiplication table using the nested For loop and While Loop with an example.
C Program to Print Multiplication Table using For Loop
In the example, we are going to write a program to generate or print a multiplication table using the nested For Loop.
#include <stdio.h> int main(void) { int i, j; printf("Multiplication Table of 8 and 9 are: \n"); for (i = 8; i < 10; i++) { for (j = 1; j <= 10; j++) { printf("%d * %d = %d\n",i ,j, i*j); } printf("\n ==========\n"); } return 0; }

This C program prints multiplication table for 8 and 9.
In the first for loop, i initialized to value 8, and then it will check whether i is less than 10. This condition will return to True until i reach 10. If this condition is True, then it will enter into second; otherwise, it will exit from it.
for (i = 8; i < 10; i++)
In the second for loop, j initialized to value 1. Then it will check whether i is less than or equal to 10. This condition will return True until j reaches 11. The concept of nesting one for inside another is called Nested For Loops.
If you find it difficult to understand the concept, Please refer to the For loop article in C programming.
for (j = 1; j <= 10; j++)
Iteration 1: i = 8 means the condition is True, so the C Program compiler will enter the second. Within the Second For, j = 1, and the condition j <= 10 is True, so the statement inside the loop will print
8 * 1 = 8
Now the value of j is incremented to 2
8 * 2 = 16
C Program to Print Multiplication Table will repeat the for loop iteration process up to 10.
Now the value of j is 11, and the condition fails. So, it will exit from the second.
NOTE: It will only exit from the inner but not from the entire loop.
Iteration 2: i = 9, and the condition inside the code is True, so repeat the above process
Iteration 3: i = 10, and the condition is False. For loop is terminated, there is No need to check the second one.
C Program to Print Multiplication Table using While Loop
In this example, we are going to write a program for multiplication tables using While Loop. Within this program, the first two statements will ask the user to enter any integer value less than 10, and we are assigning the user-specified value to i using scanf.
Next, we used the While and For Loop to iterate the values. Please refer to While in C to understand the while loop functionality.
#include <stdio.h> int main(void) { int i, j =1; printf("Please enter any Positive Integer Less than 10 = "); scanf("%d", &i); printf("==========\n"); while(i <= 10) { j = 1; while(j <= 10) { printf(" %d * %d = %d\n",i ,j, i * j); j++; } printf("==========\n"); i++; } return 0; }
Multiplication Table using a while Loop output.
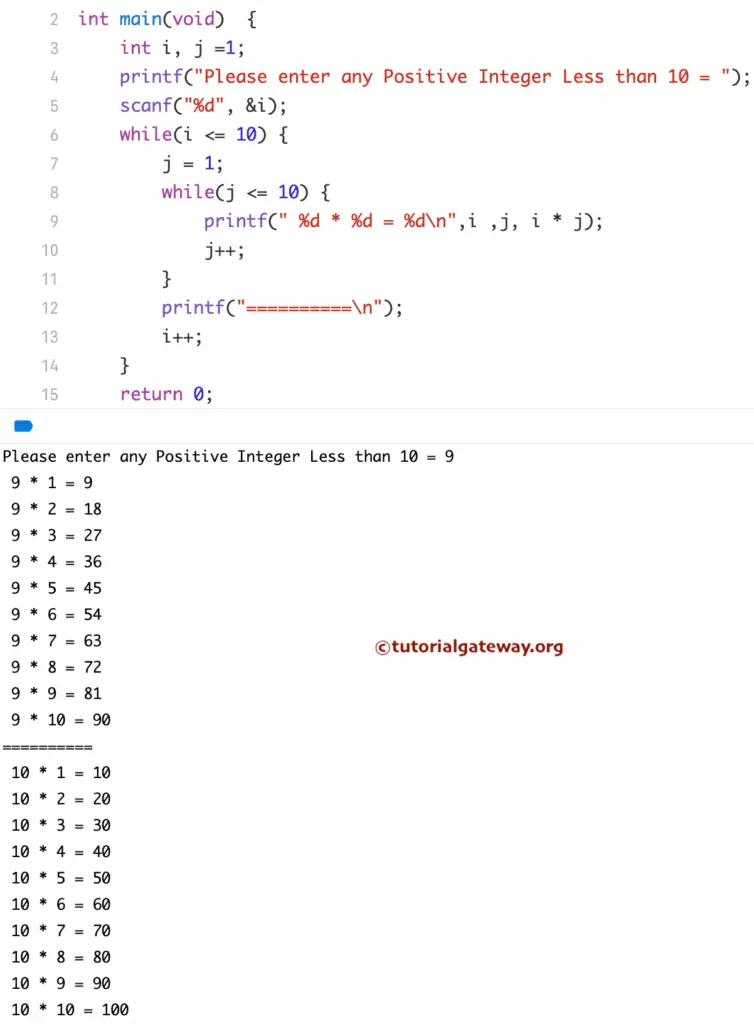