How to write a C Program to Print Inverted Pyramid Star Pattern with example?. And also show you how to print an Inverted Pyramid with different symbols.
C Program to Print Inverted Pyramid Star Pattern using While Loop
This C program allows the user to enter the maximum number of rows he/she wants to print as an Inverted Pyramid Star pattern. Here, we are going to print the inverted Pyramid of * symbols until it reaches the user-specified rows.
#include <stdio.h> int main() { int Rows, i, j, k = 0; printf("Please Enter the Number of Rows: "); scanf("%d", &Rows); printf("Printing Inverted Pyramid Star Pattern \n \n"); for ( i = Rows ; i >= 1; i-- ) { for ( j = 0 ; j <= Rows-i; j++ ) { printf(" "); } k = 0; while (k != (2 * i - 1)) { printf("*"); k++; } printf("\n"); } return 0; }
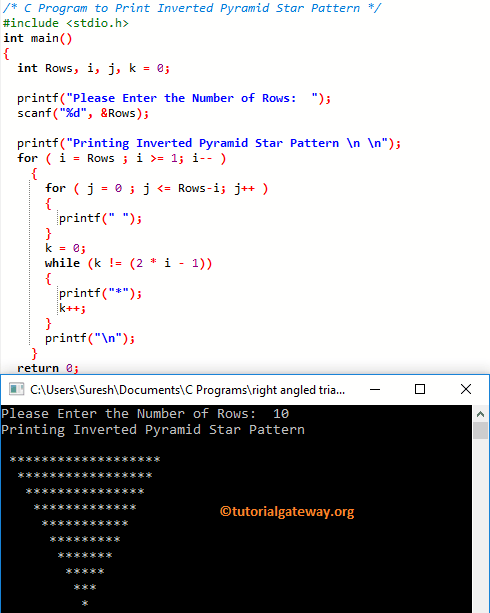
Let us see the Nested for loop
Outer Loop – First Iteration: From the above C Programming screenshot, you can observe that the value of i is ten, and Rows is ten, so the condition (i >= 1) is True. So, it will enter into the second for loop.
Inner For Loop – First Iteration
The j value is 1 and the condition (j <= 9) is True. So, it will start executing the statements inside the loop. So, it will start executing printf(” “) statements until the condition fails.
Inner While Loop – First Iteration
The k value is 0, and the condition k != 2*i – 1 is True. So, it will start executing the statements inside the loop. So, it will start executing printf(“*”) statements until the condition fails.
Next, the iteration will start from the beginning until both the Inner Loops and Outer loop conditions fail.
Program to Print Inverted Pyramid Star Pattern using For Loop
In this C program, we just replaced the While Loop with the For Loop. I suggest you refer For Loop article to understand the logic.
#include <stdio.h> int main() { int Rows, i, j, k = 0; printf("Please Enter the Number of Rows: "); scanf("%d", &Rows); for ( i = Rows ; i >= 1; i-- ) { for ( j = 0 ; j <= Rows-i; j++ ) { printf(" "); } for (k = 0; k < (2 * i - 1); k++) { printf("*"); } printf("\n"); } return 0; }
Please Enter the Number of Rows: 10
*******************
*****************
***************
*************
***********
*********
*******
*****
***
*
This C program allows the user to enter the Symbol and the number of rows he/she wants to print. It means that instead of printing pre-defined stars, it allows the user to enter their own character.
#include <stdio.h> int main() { int Rows, i, j, k; char Ch; printf("Please Enter any Symbol : "); scanf("%c", &Ch); printf("Please Enter the Number of Rows: "); scanf("%d", &Rows); for ( i = Rows ; i >= 1; i-- ) { for ( j = 0 ; j <= Rows-i; j++ ) { printf(" "); } k = 0; while (k != (2 * i - 1)) { printf("%c", Ch); k++; } printf("\n"); } return 0; }
Please Enter any Symbol : $
Please Enter the Number of Rows: 10
$$$$$$$$$$$$$$$$$$$
$$$$$$$$$$$$$$$$$
$$$$$$$$$$$$$$$
$$$$$$$$$$$$$
$$$$$$$$$$$
$$$$$$$$$
$$$$$$$
$$$$$
$$$
$