Write a C program to print the Hollow Right Pascal Triangle Star Pattern using for loop, while loop, and functions with an example. The below Hollow Right Pascal program uses the nested for loops to print the star pattern.
#include<stdio.h> void loopcode(int rows, int i) { for (int j = 1; j <= i; j++) { if (j == 1 || j == i ) printf("* "); else printf(" "); } printf("\n"); } int main(void) { int i, rows; printf("Enter Rows for Hollow Right Pascal Triangle = "); scanf("%d", &rows); for (i = 0; i < rows; i++) { loopcode(rows, i); } for (i = rows; i >= 0; i--) { loopcode(rows, i); } }
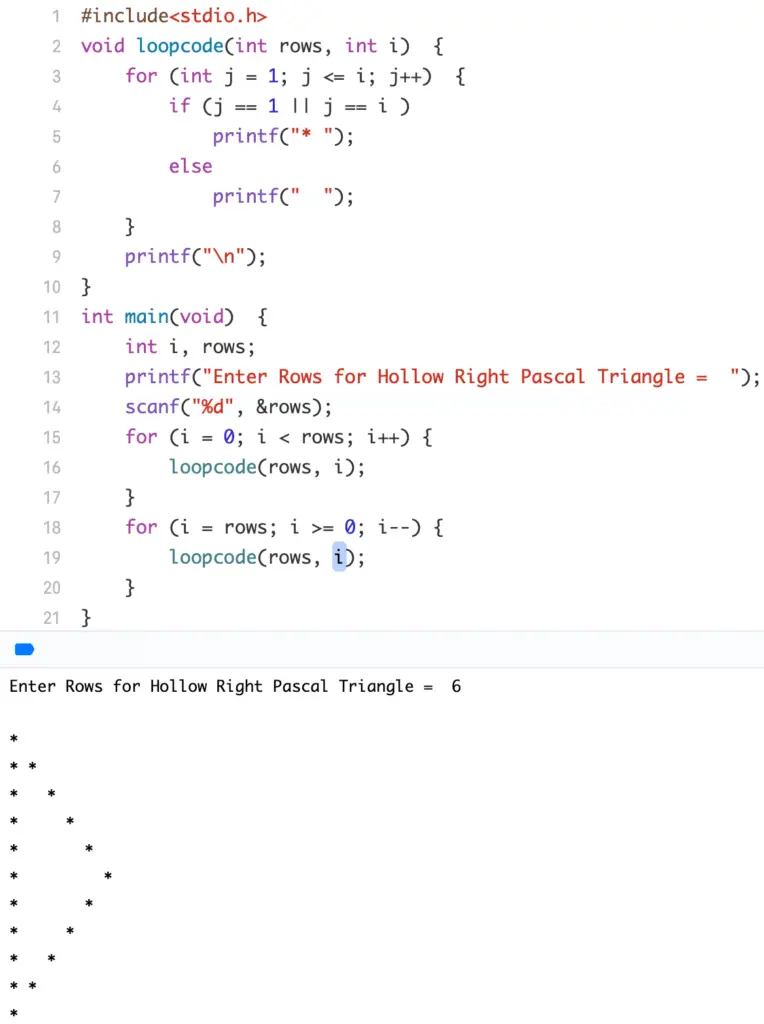
C Program to Print Hollow Right Pascal Triangle Star Pattern using while loop
We replaced the for loop with the while loop in this Hollow Right Pascal Triangle Stars Pattern program.
#include<stdio.h> void loopcode(int rows, int i) { int j = 1; while (j <= i ) { if (j == 1 || j == i ) printf("* "); else printf(" "); j++; } printf("\n"); } int main(void) { int i, rows; printf("Enter Rows for Hollow Right Pascal Triangle = "); scanf("%d", &rows); i = 0; while ( i < rows ) { loopcode(rows, i); i++; } i = rows; while ( i >= 0 ) { loopcode(rows, i); i--; } }
Output
Enter Rows for Hollow Right Pascal Triangle = 11
*
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
*
In the below Hollow Right Pascal Triangle Stars pattern program, the function accepts the rows and characters to print the pattern using the symbol.
#include<stdio.h> void loopcode(int rows, int i, char ch) { for (int j = 1; j <= i; j++) { if (j == 1 || j == i ) printf("%c ", ch); else printf(" "); } printf("\n"); } int main(void) { int i, rows; char ch; printf("Enter Character = "); scanf("%c", &ch); printf("Enter Rows for Hollow Right Pascal Triangle = "); scanf("%d", &rows); for (i = 0; i < rows; i++) { loopcode(rows, i, ch); } for (i = rows; i >= 0; i--) { loopcode(rows, i, ch); } }
Output
Enter Character = $
Enter Rows for Hollow Right Pascal Triangle = 15
$
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$