Write a C Program to print a hollow diamond inside a square star pattern using for loop, while loop, and functions with custom symbols.
C Program to Print Hollow Diamond Pattern inside a Square
In this example, we used multiple nested for loops to iterate the rows and print the hollow diamond pattern inside a square pattern.
#include<stdio.h> int main(void) { int i, j, k, rows; printf("Enter Hollow Diamond inside Square Rows = "); scanf("%d", &rows); for (i = 1 ; i <= rows; i++ ) { for (j = i ; j <= rows; j++ ) { printf("*"); } for (j = 1 ; j <= 2 * i - 2; j++ ) { printf(" "); } for (k = i ; k <= rows; k++ ) { printf("*"); } printf("\n"); } for (i = 1 ; i <= rows; i++ ) { for (j = 1 ; j <= i; j++ ) { printf("*"); } for (k = 2 * i - 2 ; k < 2 * rows - 2; k++ ) { printf(" "); } for (k = 1 ; k <= i; k++ ) { printf("*"); } printf("\n"); } }
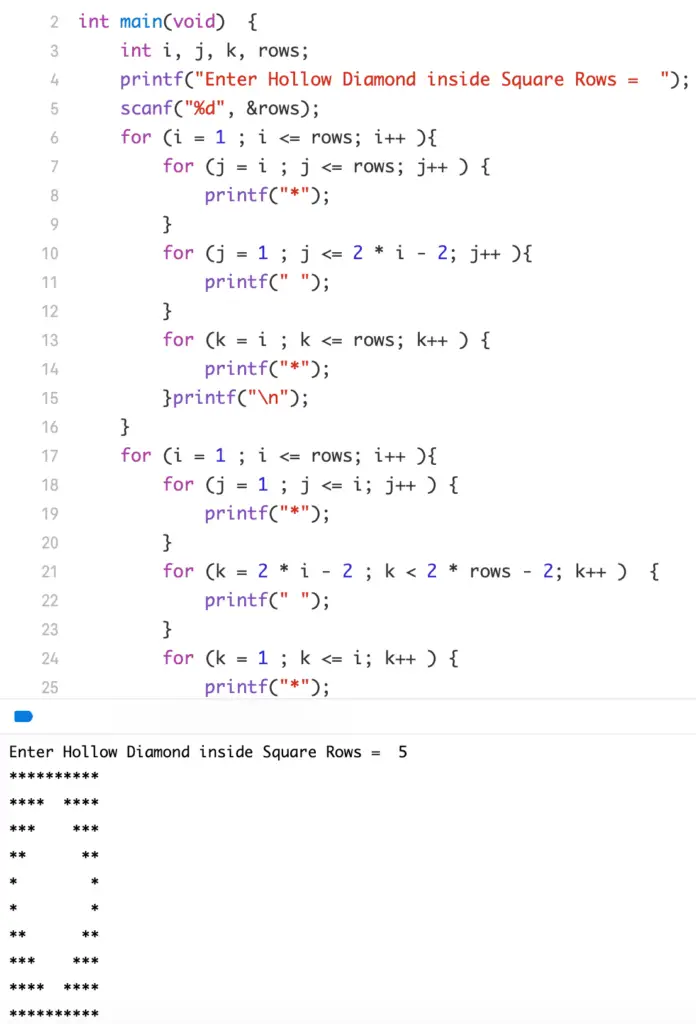
In this C hollow diamond star pattern inside a square program, we replace the above for loop code with the while loop and experiment with the if else block to get the result.
#include<stdio.h> int main(void) { int i, j, k, rows; printf("Enter Hollow Diamond inside Square Rows = "); scanf("%d", &rows); i = 0 ; while( i < rows) { j = 0 ; while ( j < rows ) { if(j < rows - i) { printf("*"); } else { printf(" "); } j++; } k = 0 ; while ( k < rows) { if (k < i ) { printf(" "); } else { printf("*"); } k++ ; } printf("\n"); i++; } i = 1 ; while ( i <= rows ) { j = 0 ; while ( j < rows ) { if(j < i) { printf("*"); } else { printf(" "); } j++; } k = 0 ; while ( k < rows) { if (k < rows - i ) { printf(" "); } else { printf("*"); } k++ ; } printf("\n"); i++; } }
Enter Hollow Diamond inside Square Rows = 9
******************
******** ********
******* *******
****** ******
***** *****
**** ****
*** ***
** **
* *
* *
** **
*** ***
**** ****
***** *****
****** ******
******* *******
******** ********
******************
This C hollow diamond Pattern inside a square program is the same as the second example. However, we replaced the static * symbol with the user-entered special character and replaced the while loop with for loop.
#include<stdio.h> int main(void) { int i, j, k, rows; char ch; printf("Enter Symbol = "); scanf("%c", &ch); printf("Enter Hollow Diamond inside Square Rows = "); scanf("%d", &rows); for (i = 0 ; i < rows; i++ ) { for (j = 0 ; j < rows; j++ ) { if(j < rows - i) { printf("%c", ch); } else { printf(" "); } } for (k = 0 ; k < rows; k++ ) { if (k < i ) { printf(" "); } else { printf("%c", ch); } } printf("\n"); } for (i = 1 ; i <= rows; i++ ) { for (j = 0 ; j < rows; j++ ) { if(j < i) { printf("%c", ch); } else { printf(" "); } } for (k = 0 ; k < rows; k++ ) { if (k < rows - i ) { printf(" "); } else { printf("%c", ch); } } printf("\n"); } }
Enter Symbol = @
Enter Hollow Diamond inside Square Rows = 9
@@@@@@@@@@@@@@@@@@
@@@@@@@@ @@@@@@@@
@@@@@@@ @@@@@@@
@@@@@@ @@@@@@
@@@@@ @@@@@
@@@@ @@@@
@@@ @@@
@@ @@
@ @
@ @
@@ @@
@@@ @@@
@@@@ @@@@
@@@@@ @@@@@
@@@@@@ @@@@@@
@@@@@@@ @@@@@@@
@@@@@@@@ @@@@@@@@
@@@@@@@@@@@@@@@@@@