How to write a C Program to Print Exponentially Increasing Star Pattern with example?. And also show you how to print Exponentially Increasing Pattern with different symbols.
C Program to Print Exponentially Increasing Star Pattern
This C program allows the user to enter the maximum number of rows he/she want to return as exponentially increasing Star Pattern. Here, we are going to print the * symbols until it reaches the user-specified rows.
/* C Program to Print Exponentially Increasing Star Pattern */ #include <stdio.h> #include <math.h> int main() { int Rows, i, j; printf("Please Enter the Number of Rows: "); scanf("%d", &Rows); printf("\nPrinting Exponentially Increasing Star Pattern \n"); for ( i = 0 ; i <= Rows; i++ ) { for ( j = 1 ; j <= pow(2, i); j++ ) { printf("* "); } printf("\n"); } return 0; }
Please Enter the Number of Rows: 5
Printing Exponentially Increasing Star Pattern
*
* *
* * * *
* * * * * * * *
* * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
C Program to return Exponentially Increasing Star Pattern
This program allows the user to enter the Symbol and number of rows he/she want to print. It means, instead of printing pre-defined stars, this C Program will enable the user to enter their own character.
#include <stdio.h> #include <math.h> int main() { int Rows, i, j; char Ch; printf("Please Enter any Symbol: "); scanf("%c", &Ch); printf("Please Enter the Number of Rows: "); scanf("%d", &Rows); printf("\nPrinting Exponentially Increasing Star Pattern \n"); for ( i = 0 ; i <= Rows; i++ ) { for ( j = 1 ; j <= pow(2, i); j++ ) { printf("%c ", Ch); } printf("\n"); } return 0; }
Please Enter any Symbol: $
Please Enter the Number of Rows: 4
Printing Exponentially Increasing Star Pattern
$
$ $
$ $ $ $
$ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
C Program to display Exponentially Increasing Pattern using While Loop
In this C program, we just replaced the For Loop with the While Loop. I suggest you refer to While Loop article to understand the logic.
#include <stdio.h> #include <math.h> int main() { int Rows, i = 0, j; char Ch; printf("Please Enter any Symbol: "); scanf("%c", &Ch); printf("Please Enter the Number of Rows: "); scanf("%d", &Rows); printf("\n---- Printing Exponentially Increasing Star Pattern ---- \n"); while ( i <= Rows) { for ( j = 1 ; j <= pow(2, i); j++ ) { printf("%c ", Ch); } printf("\n"); i++; } return 0; }
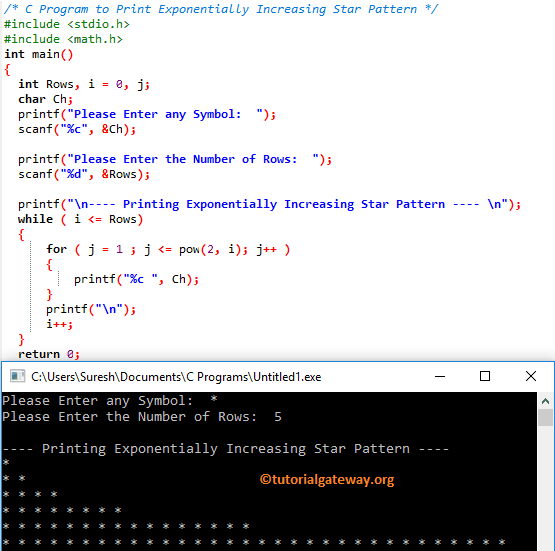