Write a C program to find the distance between two points. As per the Pythagoras theorem, the distance between two points, i.e., (x1, y1) and (x2, y2), is √(x2 – x1)2 + (y2 – y1)2.
This example accepts two coordinates and prints the distance between them. We used the C library Math functions sqrt and pow to calculate the square root and power.
#include <stdio.h> #include<math.h> int main() { int x1, x2, y1, y2, dtn; printf("Enter the First Point Coordinates = "); scanf("%d %d",&x1, &y1); printf("Enter the Second Point Coordinates = "); scanf("%d %d",&x2, &y2); int x = pow((x2- x1), 2); int y = pow((y2- y1), 2); dtn = sqrt(x + y); printf("\nThe Distance Between Two Points = %d\n", dtn); }
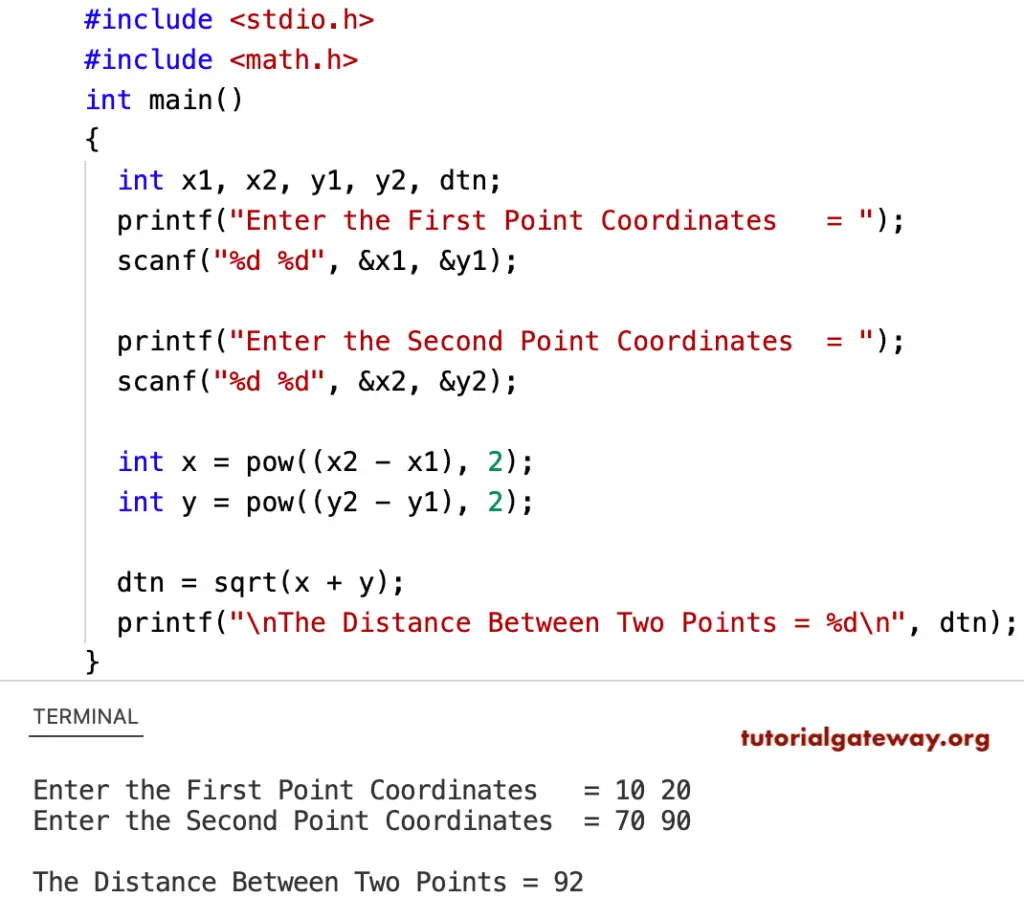
In this C program, the calcDis function accepts the coordinates of two points and returns the distance between those two points.
#include <stdio.h> #include<math.h> int calcDis(int x1, int x2, int y1, int y2) { return sqrt((pow((x2- x1), 2)) + (pow((y2- y1), 2))); } int main() { int x1, x2, y1, y2; printf("Enter the First Coordinates = "); scanf("%d %d",&x1, &y1); printf("Enter the Second Coordinates = "); scanf("%d %d",&x2, &y2); printf("\nThe Distance = %d\n", calcDis(x1, x2, y1, y2)); }
Enter the First Coordinates = 2 3
Enter the Second Coordinates = 9 11
The Distance = 10