Write a C Program to find the Sum of the Arithmetic Progression Series (A.P. Series) using Mathematical formulas and without using Math formulas.
C Arithmetic Progression Series
Arithmetic Series is a sequence of terms in which the next element is obtained by adding a common difference to the prior item. Or A.P. series is a series of numbers in which the difference between any two consecutive numbers is always the same. It is called a common difference.
In Mathematical behind calculating Arithmetic Progression Series
Sum of A.P. Series : Sn = n/2(2a + (n – 1) d)
In term of A.P. Series: Tn = a + (n – 1) d
C Program to find Sum of Arithmetic Progression Series Example
This program allows the user to enter the first value, the total number of elements in a series, and the common difference. Next, it will find the sum of the Arithmetic Progression Series. Here, we used For Loop to display the A.P series, which is optional.
#include <stdio.h> int main() { int a, n, d, tn, i; int sum = 0; printf(" Please Enter First Number of an A.P Series: "); scanf("%d", &a); printf(" Please Enter the Total Numbers in this A.P Series: "); scanf("%d", &n); printf(" Please Enter the Common Difference: "); scanf("%d", &d); sum = (n * (2 * a + (n - 1) * d)) / 2; tn = a + (n - 1) * d; printf("\n The Sum of Series A.P. : "); for(i = a; i <= tn; i = i + d) { if(i != tn) printf("%d + ", i); else printf("%d = %d", i, sum); } printf("\n"); return 0; }
Please Enter First Number of an A.P Series: 1
Please Enter the Total Numbers in this A.P Series: 4
Please Enter the Common Difference: 5
The Sum of Series A.P. : 1 + 6 + 11 + 16 = 34
C Program to calculate the Sum of Arithmetic Progression Series using While Loop
This C Program is the same as above. However, we used While Loop to display the optional A.P Series
#include <stdio.h> int main() { int a, n, d, tn, i; int sum = 0; printf(" Please Enter First Number of an A.P Series: "); scanf("%d", &a); printf(" Please Enter the Total Numbers in this A.P Series: "); scanf("%d", &n); printf(" Please Enter the Common Difference: "); scanf("%d", &d); sum = (n * (2 * a + (n - 1) * d)) / 2; tn = a + (n - 1) * d; i = a; printf("\n The Sum of Series A.P. : "); while(i <= tn) { if(i != tn) printf("%d + ", i); else printf("%d = %d", i, sum); i = i + d; } printf("\n"); return 0; }
Please Enter First Number of an A.P Series: 2
Please Enter the Total Numbers in this A.P Series: 6
Please Enter the Common Difference: 3
The Sum of Series A.P. : 2 + 5 + 8 + 11 + 14 + 17 = 57
Sum of Arithmetic Progression Series without Math Formula
In this Program, we are not using any C Programming Mathematical formula.
#include <stdio.h> int main() { int a, n, d, value, i; int sum = 0; printf(" Please Enter First Number of an A.P Series: "); scanf("%d", &a); printf(" Please Enter the Total Numbers in this A.P Series: "); scanf("%d", &n); printf(" Please Enter the Common Difference: "); scanf("%d", &d); value = a; printf("\n A.P. Series : "); for(i = 0; i < n; i++) { printf("%d + ", value); sum = sum + value; value = value + d; } printf("\n The Sum of A.P Series until %d = %d\n", n, sum); return 0; }
Please Enter First Number of an A.P Series: 2
Please Enter the Total Numbers in this A.P Series: 6
Please Enter the Common Difference: 7
A.P. Series : 2 + 9 + 16 + 23 + 30 + 37 +
The Sum of A.P Series until 6 = 117
C Program to return Sum of Arithmetic Progression Series using Functions
This Program is the same as above. However, we separated the logic using Functions.
#include <stdio.h> int sumofAP(int a, int n, int d) { int sum = (n * (2 * a + (n - 1) * d)) / 2; return sum; } int main() { int a, n, d; int sum = 0; printf(" Please Enter First Number of an A.P Series: "); scanf("%d", &a); printf(" Please Enter the Total Numbers in this A.P Series: "); scanf("%d", &n); printf(" Please Enter the Common Difference: "); scanf("%d", &d); sum = sumofAP(a, n, d); printf("\n The Sum of Arithmetic Progression Series is = %d\n", sum); return 0; }
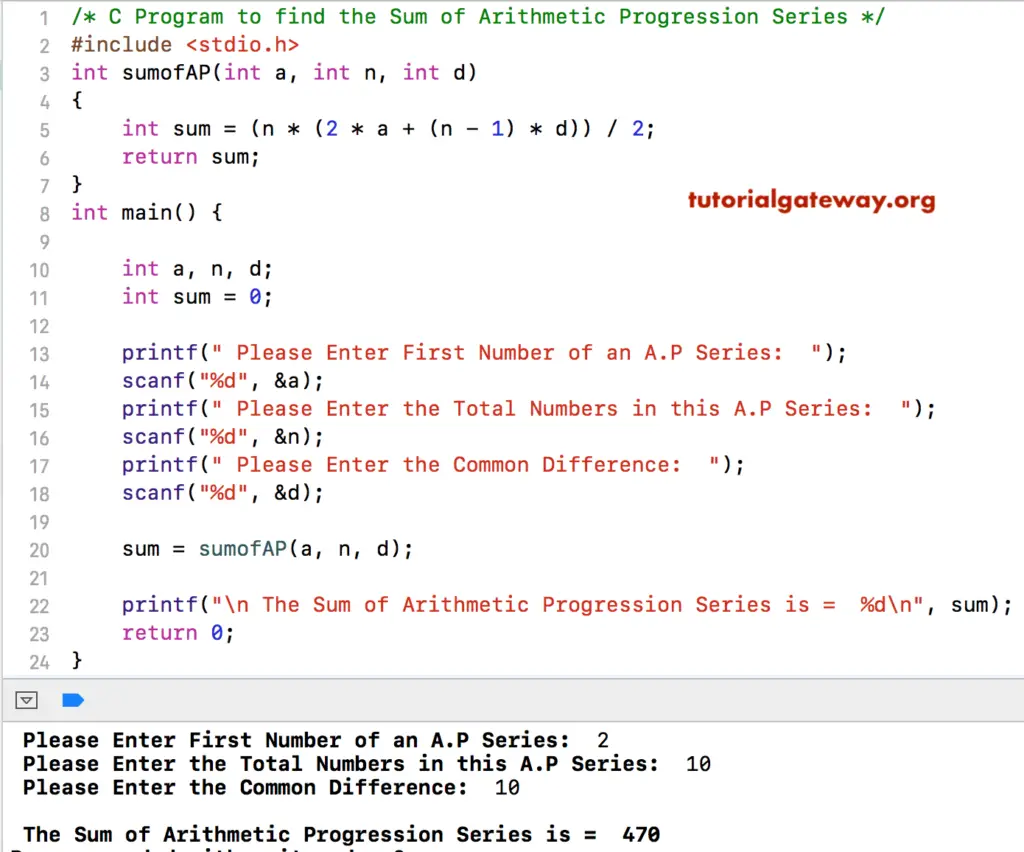