How to Write C Program to Find NCR Factorial of a Number with an example. The mathematical formula behind this NCR factor is nCr = n! / (r! (n-r)!)
C Program to Find NCR Factorial of a Number
This program allows the user to enter two positive integers. And then, it will calculate the NCR Factorial of the given number
/* C Program to Find NCR Factorial of a Number */ #include <stdio.h> int Cal_Fact(int); int main() { int n, r, ncr; printf("\n Please Enter the Values for N and R: \n"); scanf("%d %d", &n, &r); ncr = Cal_Fact(n) / (Cal_Fact(r) * Cal_Fact(n-r)); printf("\n NCR Factorial of %d and %d = %d", n, r, ncr); return 0; } int Cal_Fact(int Number) { int i; int Factorial = 1; for (i = 1; i <= Number; i++) { Factorial = Factorial * i; } return Factorial; }
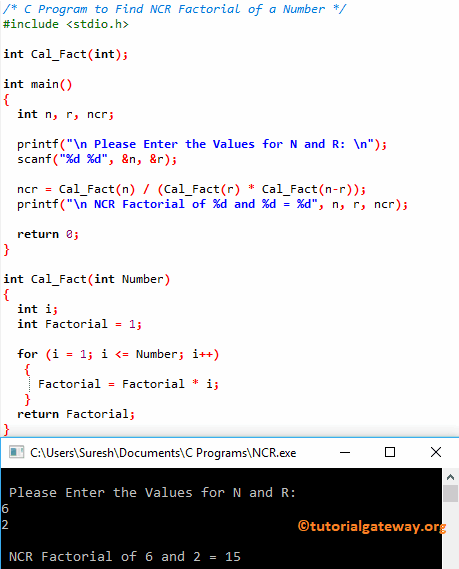
From the above program to Find NCR Factorial of a Number code snippet, we used a function called Cal_Fact to calculate the factorial of a number. Next, we are calling that function to calculate the factorial of n, r, and n-r. I suggest you to refer Factorial of Number article in C Programming to understand the factorial program
nCr = 6! / (2! (6-2)!) = 720/ (2 * 24) = 15