How to write a C Program to find ASCII Value of Total Characters in a String using For loop and while loop with a practical example.
C Program to find ASCII Value of Total Characters in a String Example
This program uses For Loop to iterate each character inside a string. Inside this, we used the printf statement to print characters and their ASCII values.
/* C Program to find ASCII Value of Total Characters in a String */ #include <stdio.h> int main() { char str[100]; printf("\n Please Enter any String : "); scanf("%s", str); for( int i = 0; str[i] != ‘\0’; i++) { printf(" The ASCII Value of Character %c = %d \n", str[i], str[i]); } return 0; }
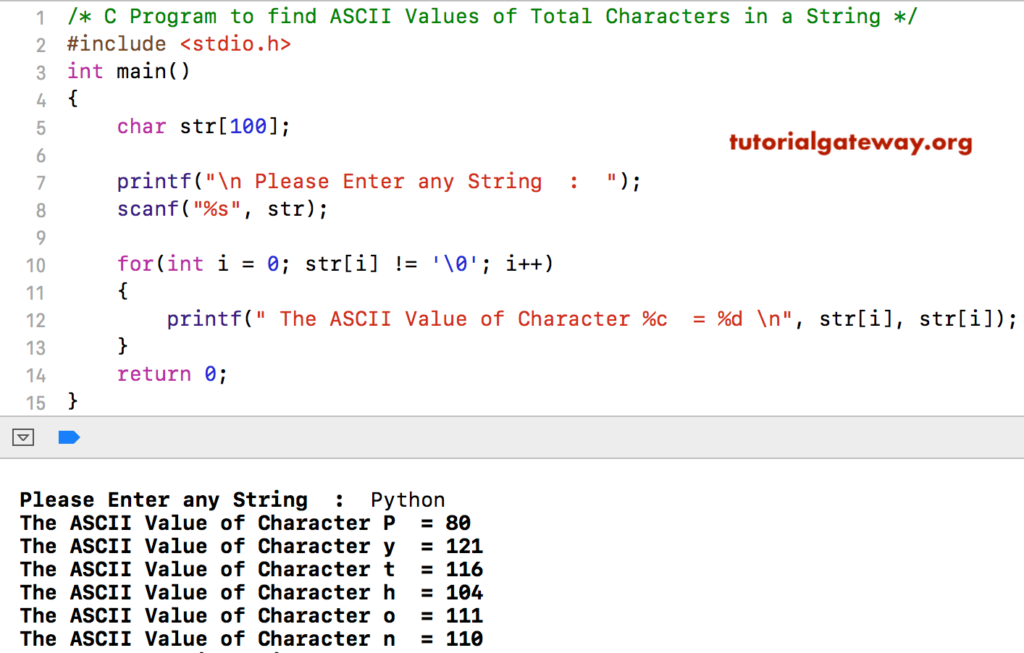
str[] = python
For Loop First Iteration: for( int i = 0; str[i] != ‘\0’; i++)
The condition is True because str[0] = p. So, the compiler will execute the printf statement.
Do the same for the remaining For Loop iterations. Please refer to the ASCII table to understand ASCII values in C Programming.
Program to find ASCII Value of Total Characters in a String using While loop
In this ASCII values C program, We replaced the For Loop with While Loop.
/* C Program to find ASCII Values of Total Characters in a String */ #include <stdio.h> int main() { char str[100]; int i = 0; printf("\n Please Enter any String : "); scanf("%s", str); while( str[i] != '\0') { printf(" The ASCII Value of Character %c = %d \n", str[i], str[i]); i++; } return 0; }
Please Enter any String : TutorialGateway
The ASCII Value of Character T = 84
The ASCII Value of Character u = 117
The ASCII Value of Character t = 116
The ASCII Value of Character o = 111
The ASCII Value of Character r = 114
The ASCII Value of Character i = 105
The ASCII Value of Character a = 97
The ASCII Value of Character l = 108
The ASCII Value of Character G = 71
The ASCII Value of Character a = 97
The ASCII Value of Character t = 116
The ASCII Value of Character e = 101
The ASCII Value of Character w = 119
The ASCII Value of Character a = 97
The ASCII Value of Character y = 121