Write a C program to convert octal to decimal using a while loop. In this c example, the while loop iterate and divides the value into digits, and the decimal = decimal + (octal % 10) * pow(8, i++) statement convert octal to decimal number.
#include <stdio.h> #include <math.h> int main() { int octal, decimal = 0; int i = 0; printf("Enter the Octal Number = "); scanf("%d",&octal); while(octal != 0) { decimal = decimal + (octal % 10) * pow(8, i++); octal = octal / 10; } printf("The Decimal Value = %d\n", decimal); return 0; }
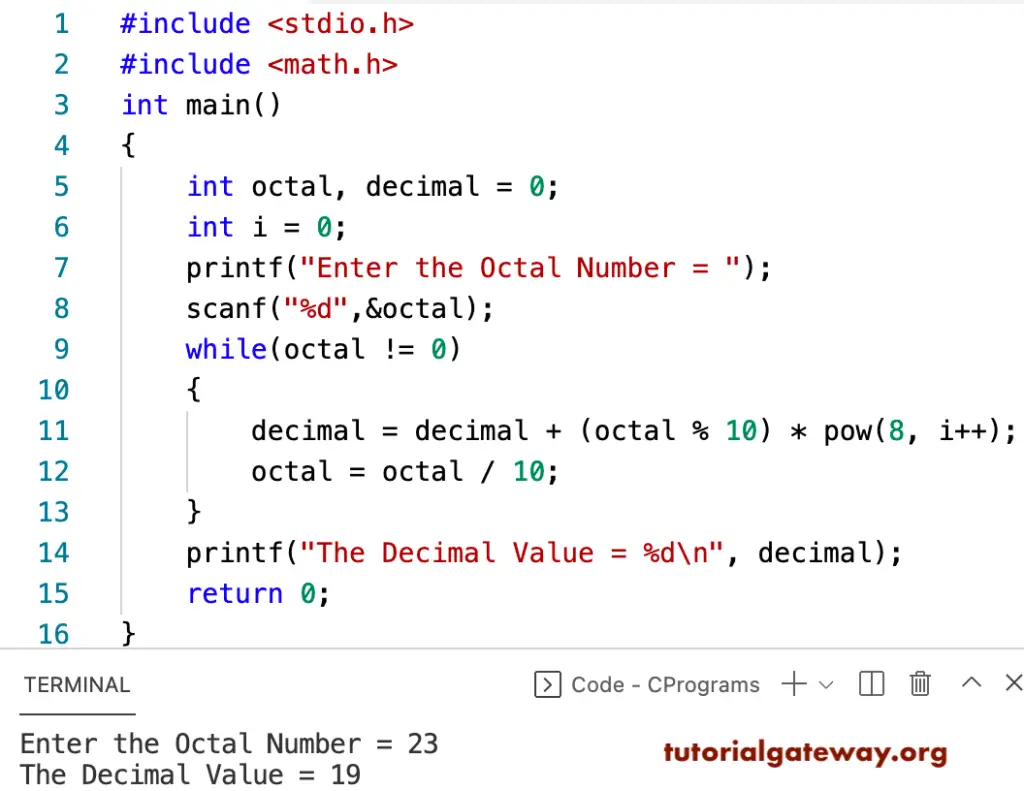
In this c program, the decimalToOctal function accepts the octal number and converts it to a decimal using the for loop.
#include <stdio.h> #include <math.h> long decimalToOctal(int octal) { long decimal = 0, i = 0; while(octal != 0) { decimal = decimal + (octal % 10) * pow(8, i++); octal = octal / 10; } return decimal; } int main() { int octal; int i = 0; printf("Enter the Number = "); scanf("%d",&octal); long deci = decimalToOctal(octal); printf("Result = %ld\n", deci); return 0; }
Enter the Number = 987
Result = 647
Enter the Number = 15
Result = 13