Write a C Program to Check the Number is Divisible by 5 and 11 with an example using If Else Statement, and the Conditional Operator.
C Program to Check the Number is Divisible by 5 and 11 Example
This program helps the user to enter any number. Next, this program checks whether the number is divisible by both 5 and 11 using If Else.
/* C Program to Check the Number is Divisible by 5 and 11 */ #include<stdio.h> int main() { int number; printf("\n Please Enter any Number to Check whether it is Divisible by 5 and 11 : "); scanf("%d", &number); if (( number % 5 == 0 ) && ( number % 11 == 0 )) printf("\n Given number %d is Divisible by 5 and 11", number); else printf("\n Given number %d is Not Divisible by 5 and 11", number); return 0; }
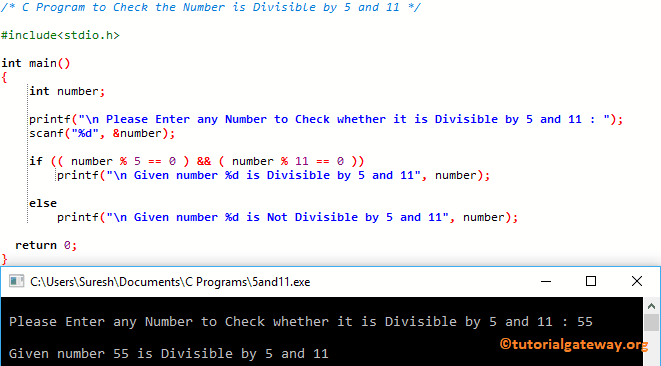
Program to Check the Number is Divisible by 5 and 11 using Conditional Operator
This program will use the Conditional Operator in C Programming to check whether it is divisible by both 5 and 11 in C.
#include<stdio.h> int main() { int num; printf("\n Please Enter any Value : "); scanf("%d",&num); ((num % 5 == 0 ) && ( num % 11 == 0 )) ? printf("\n %d is Divisible by 5 and 11", num) : printf("\n %d is Not Divisible by 5 and 11", num); return 0; }
Please Enter any Value : 110
110 is Divisible by 5 and 11
Let me try another value
Please Enter any Value : 205
205 is Not Divisible by 5 and 11