How to Write a C Program to Calculate Generic Root of a Number with an example?. The mathematical formula to calculate the generic root is nothing but the calculating sum of all digits in a given number until we get a single-digit output (less than 10). For example, Generic Root of 98765 = 9 + 8 + 7 + 6 + 5 = 35 = 8
C Program to Calculate Generic Root of a Number
This C program allows the user to enter one positive integer. And then, it will calculate the Generic Root of a given number
/* C Program to Calculate Generic Root of a Number */ #include <stdio.h> int main() { int Number, Sum, Reminder; printf("\n Please Enter any number\n"); scanf("%d", &Number); while(Number >= 10) { for (Sum=0; Number > 0; Number= Number/10) { Reminder = Number % 10; Sum=Sum + Reminder; } if(Sum >= 10) { Number = Sum; } else { printf("\n The Generic Root of a Given Number = %d", Sum); return 0; } } }
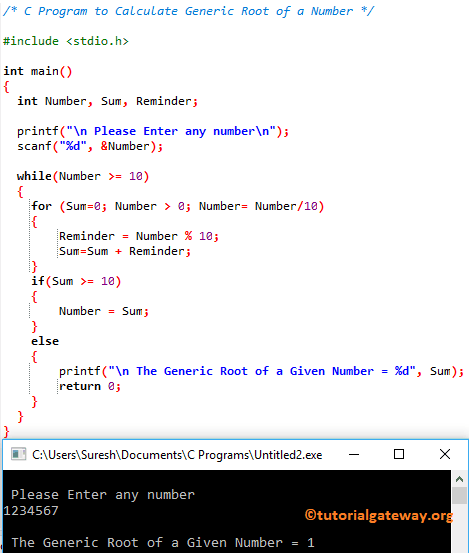
C Program to Calculate Generic Root of a Number using Functions
It is the same program that we used in the first example, but this time we separated the logic using Functions.
/* C Program to Calculate Generic Root of a Number */ #include <stdio.h> int Sum_Of_Digits (int); int main() { int Number, Sum, Root; printf("\n Please Enter any number\n"); scanf("%d", &Number); while(Number >= 10) { Sum = Sum_Of_Digits (Number); if(Sum >= 10) { Number = Sum; } else { printf("\n Generic Root of a Given Number = %d", Sum); return 0; } } } int Sum_Of_Digits (int Number) { int Reminder, Sum; for (Sum=0; Number > 0; Number = Number/10) { Reminder = Number % 10; Sum = Sum + Reminder; } return Sum; }
We used a function called Sum_of_Digits to find the Sum of all digits of a Number. I suggest you refer Sum of Digits in a Given Number article in C Programming to understand the function.
C Generic roots output
Please Enter any number
9875625
Generic Root of a Given Number = 6
Within this Program to Calculate Generic Root of a Number example,
- First, Condition inside the While loop will make sure that the given number is greater than or equal to 10. And if the condition is True, then it will call the Sum_of_Digits.
- Next, Condition inside the If Statement will make check whether the Sum_of_Digits Function Output (i.e., Sum) is greater than or equal to 10.
- If the condition is True, we are assigning Sum to Number. It means, it will again call the Sum_of_Digits with updated Number (i.e., Sum).
- If the condition is False, Print the Output.