How to write a C Program to Calculate Compound Interest with an example. Before we get into the example, let me show you the formula behind the calculation:
Future CI = Principal Amount * ( 1 + Rate of Interest) power Number of years
The above Code is called Future because it contains both the Principal Amount and Compound Interest. To calculate the CI, use the below formula:
CI = Future CI – Principal Amount
C Program to Calculate Compound Interest
This program allows the user to enter the Principal Amount, Rate of Interest, and the number of years. By using those values, this Program finds Compound interest and FCI using the above-specified formula.
#include<stdio.h> #include <math.h> int main() { float PAmount, ROI, Time_Period, CIFuture, CI; printf("\nPlease enter the Principal Amount : \n"); scanf("%f", &PAmount); printf("Please Enter Rate Of Interest : \n"); scanf("%f", &ROI); printf("Please Enter the Time Period in Years : \n"); scanf("%f", &Time_Period); CIFuture = PAmount * (pow(( 1 + ROI/100), Time_Period)); CI = CIFuture - PAmount; printf("\nFuture Compound Interest for Principal Amount %.2f is = %.2f", PAmount, CIFuture); printf("\nCompound Interest for Principal Amount %.2f is = %.2f", PAmount, CI); return 0; }
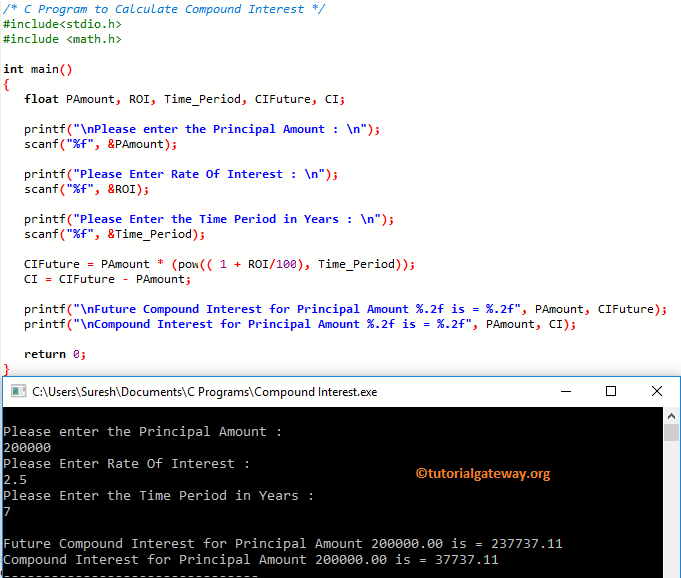