How to write a C Program to Print Alphabets between A and Z using For Loop and While Loop?.
C Program to Print Alphabets between A and Z using For Loop
This C program will display alphabets between A and Z using For Loop. In this example, For Loop will make sure that the character (ch) is between A and Z.
#include <stdio.h> int main() { char ch; for(ch = 'A'; ch <= 'Z'; ch++) { printf(" %c\t", ch); } return 0; }
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
C Program to Print Alphabets between A and Z using ASCII Codes
Instead of hard-coding A and Z, in this program, we are using the ASCII codes to print alphabets. I suggest you refer to the ASCII Table to understand the ASCII values of each character in C Programming.
From the below code snippet you can see, A = 65 and Z = 90
#include <stdio.h> int main() { int i; for(i = 65; i <= 90; i++) { printf(" %c\t", i); } return 0; }
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
This Program to Print Alphabets between A and Z example is the same as above. We just replaced the For Loop with While Loop.
#include <stdio.h> int main() { char ch = 'A'; while(ch <= 'Z') { printf(" %c\t", ch); ch++; } return 0; }
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
This C program allows the user to enter the starting alphabet (A to Z). Next, the program will print the list of all alphabets between Starting_Char and Z.
#include <stdio.h> int main() { char ch, Starting_Char; printf("\n Please Enter the Starting Alphabet : "); scanf("%c", &Starting_Char); printf("\n List of Alphabets from %c to Z are : \n", Starting_Char); for(ch = Starting_Char; ch <= 'Z'; ch++) { printf(" %c\t", ch); } return 0; }
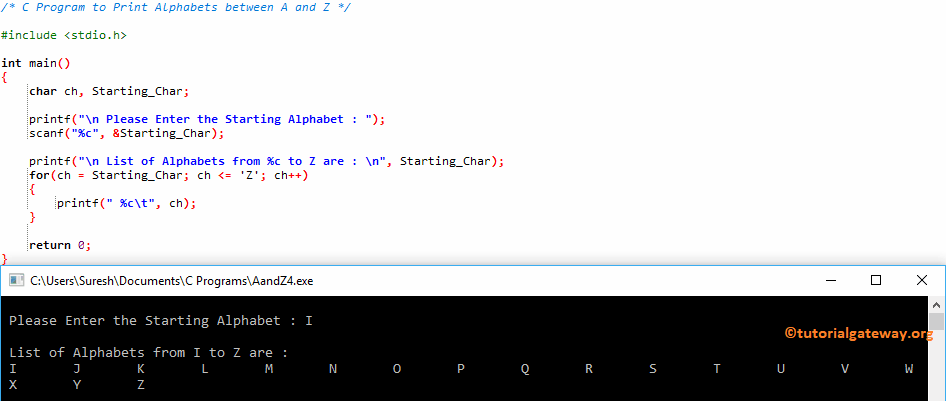