Write a Program to Find the Sum of Digits in Java using For Loop, While Loop, Functions, and Recursion.
Java program to find the Sum of Digits using While Loop
This program allows the user to enter any positive integer. Then, it will divide the given number into individuals and add those individual (Sum) digits using Java While Loop.
package SimplerPrograms; import java.util.Scanner; public class SumofDigitsUsingWhile { private static Scanner sc; public static void main(String[] args) { int Number, Reminder, Sum = 0; sc = new Scanner(System.in); System.out.println("Please Enter any Number: "); Number = sc.nextInt(); while(Number > 0) { Reminder = Number % 10; Sum = Sum+ Reminder; Number = Number / 10; } System.out.format("Sum of the digits of Given Number = %d", Sum); } }
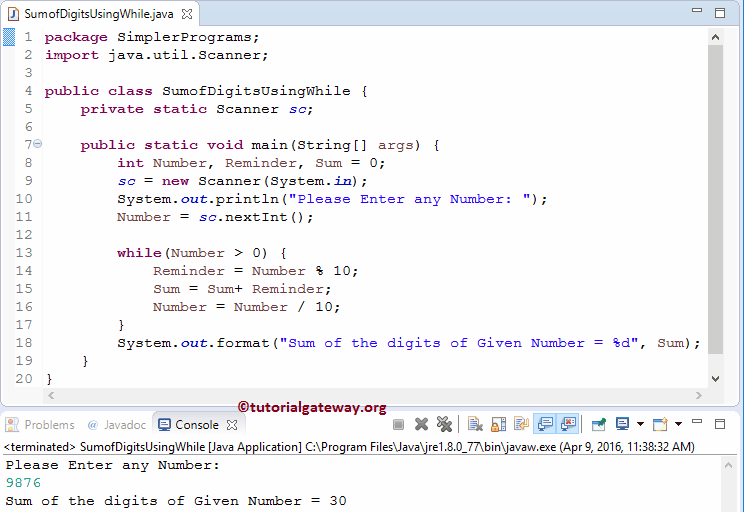
In this program, first, We declared three integer variables: Number, Remainder, and Sum = 0. The first two statements will ask the user to enter any positive integer. Then, that number is assigned to the variable Number.
Next, the Condition inside the While Loop will make sure that the given number is greater than 0 (Means Positive integer and greater than 0)
Let us see the working principle of this while loop iteration wise.
From the above Java sum of digits of a number screenshot, the user entered the value: Number= 9876.
First Iteration: For the first Iteration, Number = 9876 and Sum = 0. It means, While (9876 > 0) is TRUE. So, the program will start executing statements inside the while loop.
Reminder = Number% 10
Reminder = 9876 % 10 = 6
Next, we have to find the Sum
Sum= Sum+ Reminder
Sum= 0 + 6 = 6
Lastly, we have to remove the last digit from the user-specified number
Number = Number/ 10
Number= 9876 / 10 = 987
Second Iteration of the Sum of Digits in Java program:
From the first iteration, the values of both Number and Sum changed as Number = 987 and Sum = 6. It means, While (987 > 0) is TRUE. So, the program will start executing statements inside the while loop.
Reminder = 987 % 10 = 7
Sum= 6 + 7 = 13
Number= 987 / 10 = 98
Third Iteration
From the Second Iteration of Java sum of digits of a number program, the values of Number= 98 and Sum= 13. It means, While (98 > 0) is TRUE
Reminder = 98 % 10 = 8
Sum= 13 + 8 = 21
Number= 98 / 10 = 9
Fourth Iteration
From the third Iteration, Number = 9 and Sum = 21. It means that while (9 > 0) is TRUE.
Reminder = 9 % 10 = 9
Sum= 21 + 9 = 30
Number= 9 / 10 = 0
Here Number= 0. So, the while loop condition will fail.
The last System.out.format statement will print the Sum variable as the output. So, the output of the given variable is 9876 = 30.
Java Program to Find Sum of Digits using For Loop
This program allows the user to enter any positive integer. Then, it will divide the given number into individual digits and find the sum of those individuals using Java For Loop.
package SimplerPrograms; import java.util.Scanner; public class UsingFor { private static Scanner sc; public static void main(String[] args) { int Number, Reminder, total = 0; sc = new Scanner(System.in); System.out.println("Please Enter any Number: "); Number = sc.nextInt(); for (total = 0; Number > 0; Number = Number/10){ Reminder = Number % 10; total = total + Reminder; } System.out.format("Sum of the digits = %d", total); } }
We just replaced the While loop in the above example with the For loop. Please refer to Java For Loop.
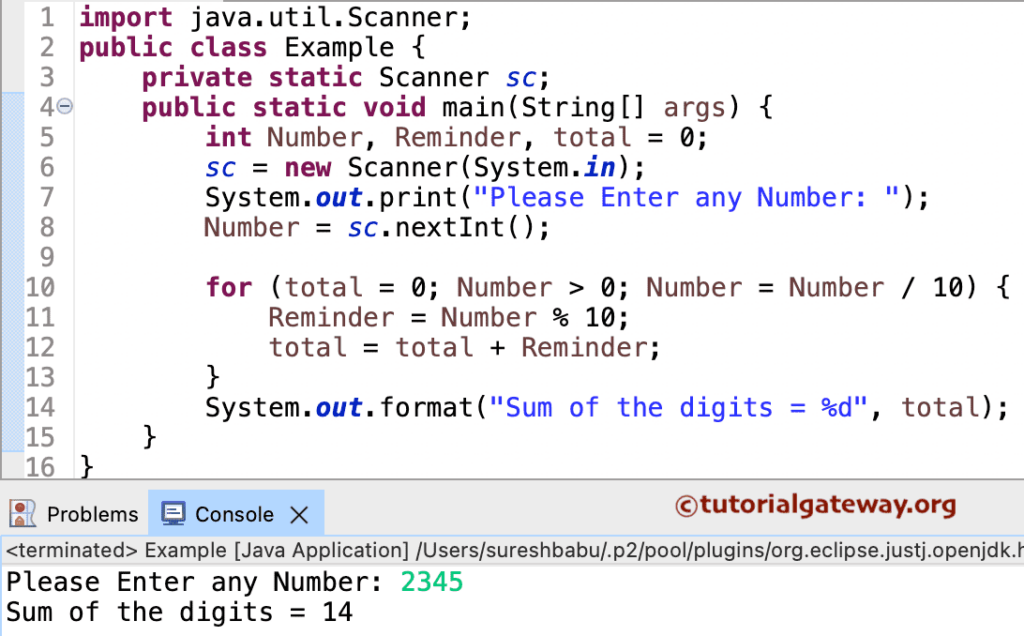
Java Program to Find Sum of Digits using Functions
This Java program allows the user to enter any positive integer value. Next, we will pass the User entered value to the Method we created.
Within this User defined function, this sum of digits program will divide the given number into individual items and add those individuals using the For Loop
package SimplerPrograms; import java.util.Scanner; public class UsingMethods { private static Scanner sc; private static int total = 0; public static void main(String[] args) { int Num; sc = new Scanner(System.in); System.out.println("Please Enter any Value: "); Num = sc.nextInt(); total = SOD(Num); System.out.format("Sum of the digits = %d", total); } public static int SOD(int Num) { int Reminder; for (total = 0; Num > 0;Num = Num/10) { Reminder = Num % 10; total = total+ Reminder; } return total; } }
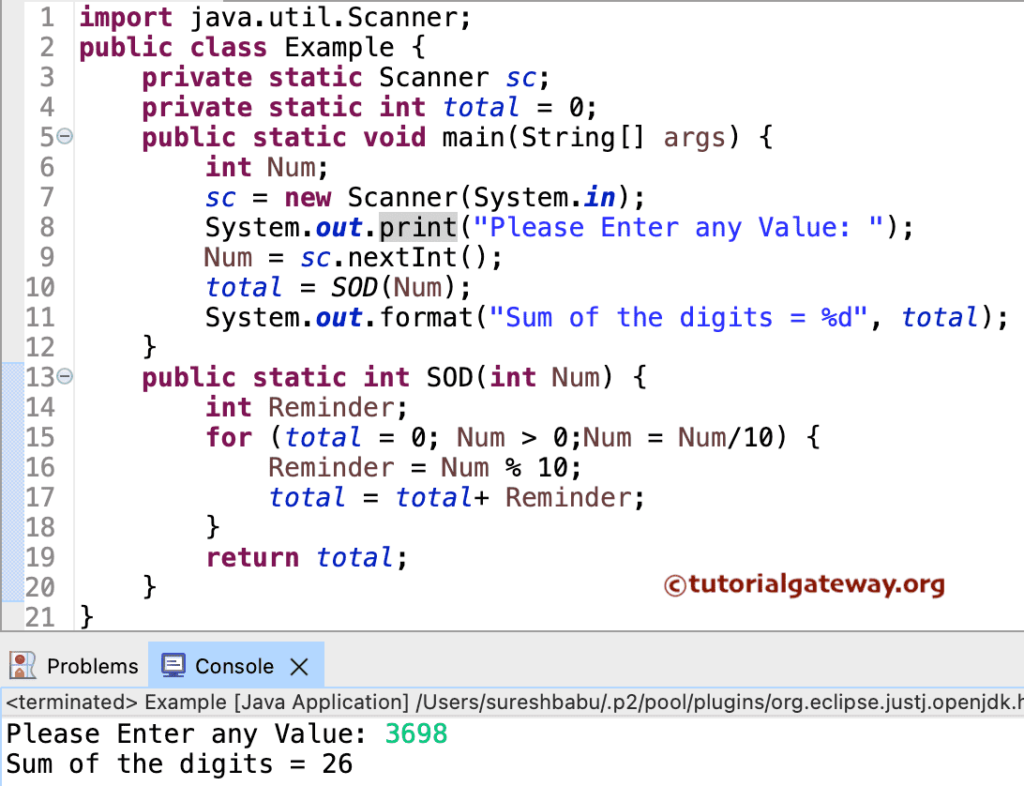
In this Java Sum of Digits program, we are calling the SOD(Number) method. As we all know, the method will return an integer value, so we are assigning that return value to Sum
total = SOD(Num);
When the compiler reaches to SOD(Num) line, the compiler will immediately jump to the below function:
public static int SOD(int Num) {
We already explained the LOGIC in the above example.
Java Program to Find Sum of Digits using Recursion
This example allows the user to enter any positive integer. Then, the Java program will divide the given number into individual digits and add those individuals as Sum by calling the function recursively.
In this program, we are dividing the code using Object-Oriented Programming. To do this, First, we will create a class that holds a method to reverse an integer recursively.
package SimplerPrograms; public class SoDigi { int t = 0, r; public int SDCalc(int n) { if(n > 0) { r = n % 10; t = t + r; SDCalc(n / 10); return t; } else { return 0; } } }
Within the Main program, we will create an instance of the above-specified class and call the methods.
package SimplerPrograms; import java.util.Scanner; public class UsingRecursion { private static Scanner sc; public static void main(String[] args) { int n, t = 0; sc = new Scanner(System.in); System.out.println("\n Please Enter any : "); n = sc.nextInt(); SoDigi sd = new SoDigi(); t = sd.SDCalc(n); System.out.format("\n Sum of the digits = %d", t); } }
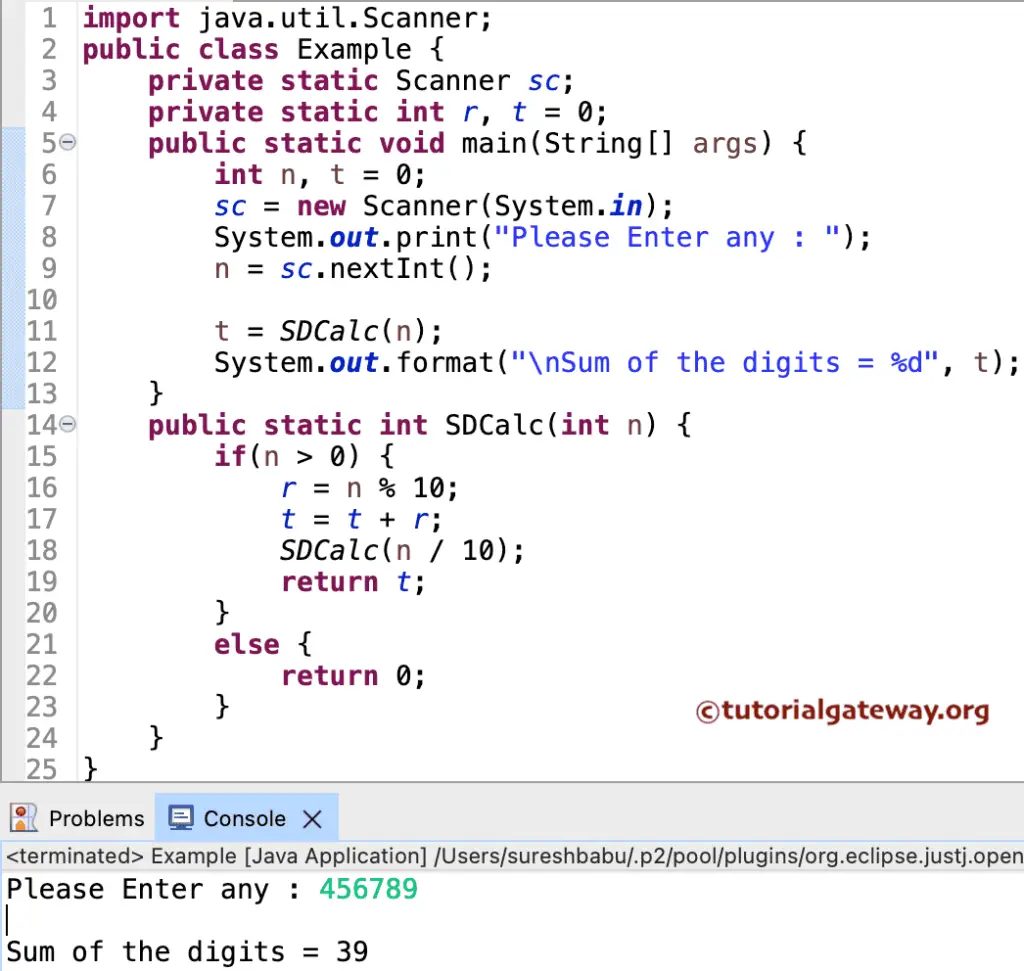
SoDigi Class Analysis:
Within this Java sum of digits program example, we defined a function in this class. The following function will accept an integer value as a parameter value and return an integer value.
public int SDCalc(int n) {
Let us see the If Else statement inside the above-specified function. If (Number > 0), it will check whether the given number is greater than zero or not.
- If the condition is True, the Java compiler will return the Sum of a given number. Here, we are using the SDCalc(Number / 10) statement inside the If block because it will help to call the function Recursively with the updated value. When you miss this statement, then after completing the first line, it will terminate. For example, Number = 4567 will return the output as 7
- If the condition is False, the compiler will return the Sum as Zero.
Main Class Analysis:
In the main class of the Java Sum of Digits program, we created an instance / created an Object of the SoDigi Class.
SoDigi sd = new SoDigi();
Next, we are calling the SDCalc(Number) method. We all know that the method will return an integer value. So, we are assigning that return value to Sum.
t = sd.SDCalc(n);
Comments are closed.