How to write a Java Program to Reverse a Number using While Loop, For Loop, Built-in reverse function, Functions, and Recursion. With no direct approach, you must use logic to extract the last digit from the number, add it to the first position, and repeat the process until all digits are completed.
Java Program to Reverse a Number Using While Loop
This Java program allows the user to enter any positive integer, and then it will reverse a number using While Loop.
package SimplerPrograms; import java.util.Scanner; public class ReverseNumberUsingWhile { private static Scanner sc; public static void main(String[] args) { int Number, Reminder, Reverse = 0; sc = new Scanner(System.in); System.out.println("\n Please Enter any Number you want to Reverse : "); Number = sc.nextInt(); while(Number > 0) { Reminder = Number %10; Reverse = Reverse * 10 + Reminder; Number = Number /10; } System.out.format("\n Reverse of entered number is = %d\n", Reverse); } }
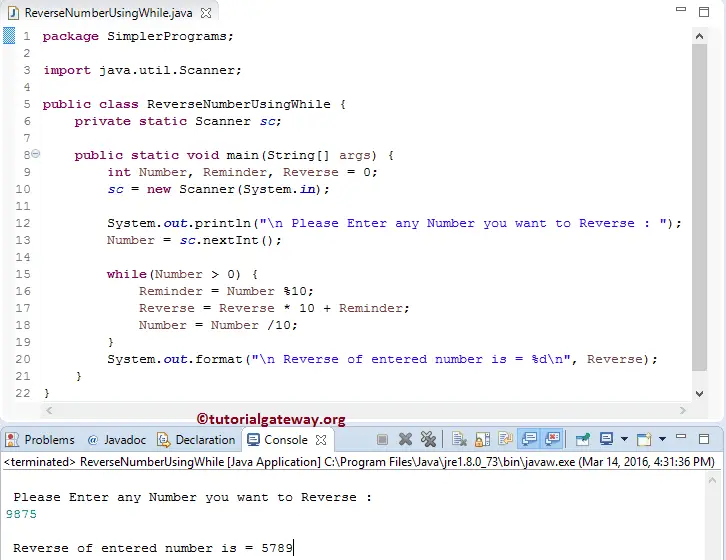
The first System.out.println statement of the Java reverse a number will be printed inside the double Quotes. And next, we assign the user entered value to an integer variable (Number)
Next line, we used the Java While Loop, and the Condition inside the While loop will make sure that the given number is greater than 0 (Which means a Positive integer)
From the above Java screenshot, the User Entered value: Number= 9875 and Reverse= 0.
Java Reverse a Number First Iteration
Reminder = Number % 10
Reminder = 9875 % 10 = 5
Reverse= Reverse* 10 + Reminder
Reverse= 0 * 10 + 5 = 5
Number= Number/ 10
Number= 9875 /10 = 987
2nd Iteration: From the first iteration of Java, the values of both Number and Reverse have changed as Number= 987 and Reverse= 5.
- Reminder = 987 % 10 = 7
- Reverse= 5 * 10 + 7 = 50 + 7 = 57
- Number= 987 /10 = 98
3rd Iteration: From the Second Iteration, Number= 98 and Reverse= 57
- Reminder = 98 % 10 = 8
- Reverse= 57 * 10 + 8 = 570 + 8 = 578
- Number = 98 / 10 = 9
4th Iteration: From the 3rd Iteration, Number= 9 and Reverse= 578
- Reminder = 9 %10 = 9
- Reverse= 578 * 10 + 9 = 5780 + 9 = 5789
- Number = 9 / 10 = 0
Here, For the next iteration, Number = 0. So, the while loop condition will fail.
Java Program to Reverse a Number Using For Loop
This Java program allows the user to enter any positive integer, and then it will reverse a number using For Loop.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int num, Reminder, rv; sc = new Scanner(System.in); System.out.println("\n Please Enter any Number you want : "); num = sc.nextInt(); for(rv = 0; num > 0; num = num/10) { Reminder = num %10; rv = rv * 10 + Reminder; } System.out.format("\n Reverse of entered is = %d\n", rv); } }
Using the for loop output.
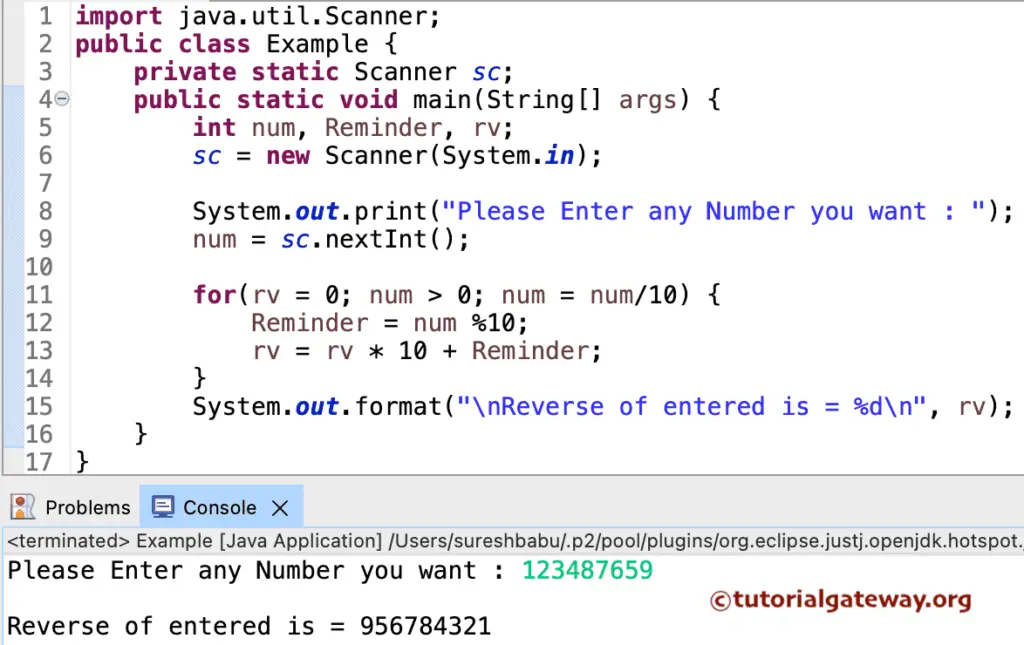
We just replaced the While loop in the above example with the Java For Loop.
Using string buffer
This Java program allows the user to enter any positive integer, and then it will reverse a number using the built-in string buffer reverse function.
package SimplerPrograms; import java.util.Scanner; public class ReverseNumberUsingSTring { private static Scanner sc; public static void main(String[] args) { int num; sc = new Scanner(System.in); System.out.println("\n Please Enter any Integer : "); num = sc.nextInt(); String str = Integer.toString(num); String rev = new StringBuffer(str).reverse().toString(); System.out.format("\n Result = %s", rev); } }
Please Enter any Integer :
6597213
Result = 3127956
The following statement will convert the integer value to a string value and assign the converted value to the Str variable.
String str = Integer.toString(num);
Next, we used the Java String Buffer reverse function to reverse the number string.
String rev = new StringBuffer(str).reverse().toString();
lastly, we are displaying the reversed string value
System.out.format("\n Result = %s", rev);
Java Program to Reverse a Number Using Functions
In this Java program, we use the same steps we followed in our first example, but we separate the logic and place it in a separate method.
import java.util.Scanner; public class Example { private static Scanner sc; private static int rv = 0; public static void main(String[] args) { int num; sc = new Scanner(System.in); System.out.println("\n Please Enter any Number you want : "); num = sc.nextInt(); rv = rvnum(num); System.out.format("\n Reverse of entered is = %d\n", rv); } public static int rvnum(int num) { int Reminder; while(num > 0) { Reminder = num %10; rv = rv * 10 + Reminder; num = num /10; } return rv; } }
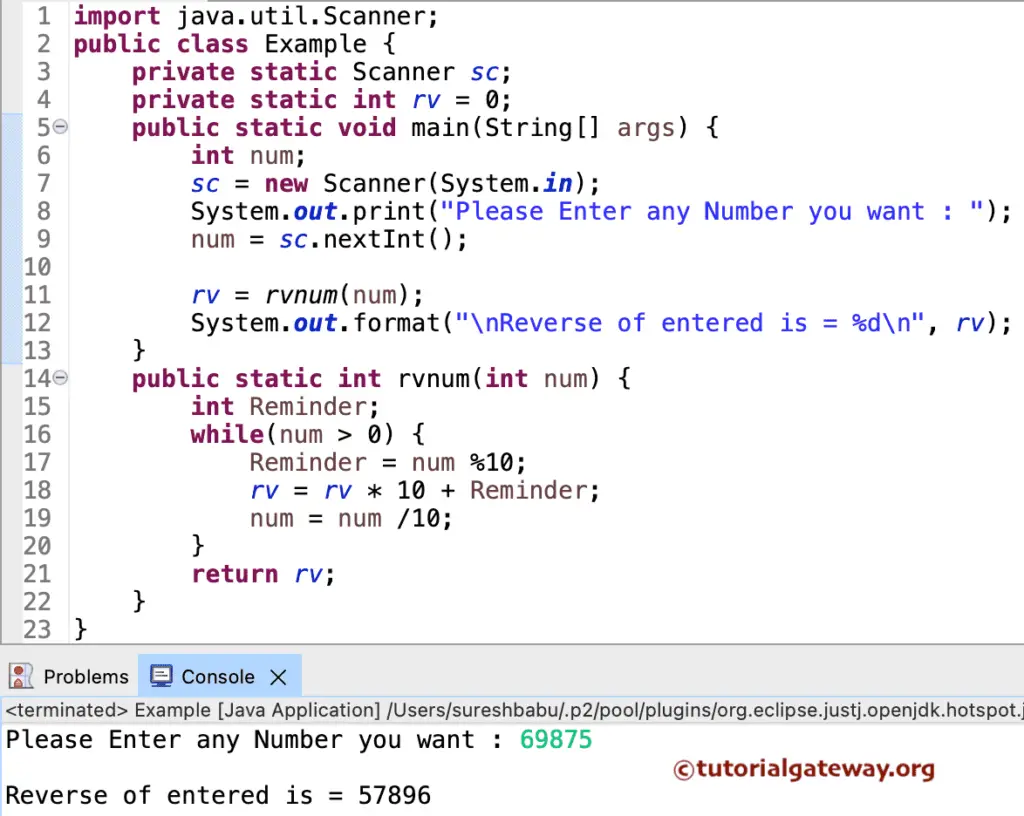
If you observe the following statement, we are calling the rvnum(num) method and assigning the return value to the integer variable Reverse.
rv = rvnum(num);
When the compiler reaches the rvnum(int num) line in the main() program, then the compiler will immediately jump to the below function:
public static int rvnum(int num) {
Java Program to Reverse a Number using Recursion
This Java program allows the user to enter any positive integer, and then it will reverse the given number using the Recursion concept. In this example, we are dividing the code using Object Oriented Programming. To do this, we will first create a class with a method to reverse an integer recursively.
package SimplerPrograms; public class ReverseNumber { int Reverse = 0, Reminder; public int NumberReverse(int Number) { if(Number > 0) { Reminder = Number %10; Reverse = Reverse * 10+ Reminder; NumberReverse(Number /10); } return Reverse; } }
Within the Main program, we will create an instance of the above-specified class and call the methods.
package SimplerPrograms; import java.util.Scanner; public class RevNumbUsingClass { private static Scanner sc; public static void main(String[] args) { int Number, Rev = 0; sc = new Scanner(System.in); System.out.println("\n Please Enter any Number you want : "); Number = sc.nextInt(); ReverseNumber rn = new ReverseNumber(); Rev = rn.NumberReverse(Number); System.out.format("\n Reverse of entered number is = %d\n", Rev); } }
Java reverse number using Oops output.
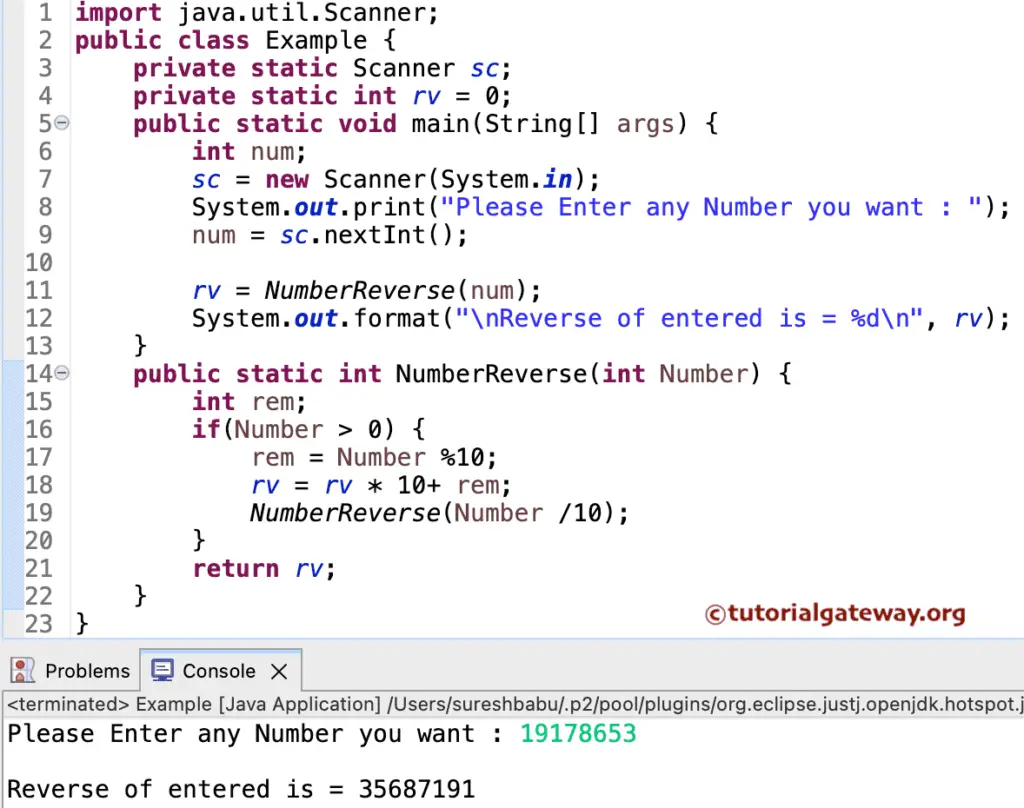
ReverseNumber Class Analysis:
First, we declared an integer function NumberReverse with one argument. Within the function, we used the If statement to check whether the given number is greater than Zero or Not, and if it is True, then statements inside the If block will be executed. We already explained the Logic in the above example.
NOTE: NumberReverse (Number / 10) statement will help to call the function Recursively with an updated value. If you miss this statement, it will terminate after completing the first line.
Main Class Analysis: First, we created an instance / created an Object of the ReverseNumber Class
ReverseNumber rn = new ReverseNumber();
Next, we are calling the NumberReverse method.
Reverse = rn.NumberReverse(Number);
Lastly, the System.out.println statement will print the output.