Write Java Program to find Volume and Surface Area of Cone with example. Before we step into the Java Program to find Volume and Surface Area of Cone, Let see the definitions and formulas behind the Surface area of a Cone and Volume of a Cone
Java Surface Area of a Cone
If we know the radius and Slant of a Cone, then we calculate the Surface Area of Cone using the below formula:
- Surface Area = Area of the Cone + Area of Circle
- Surface Area = πrl + πr² Where r= radius and l = Slant (Length of an edge from the top of the cone to edge of a cone)
If we know the radius and height of a Cone, then we calculate the Surface Area of Cone using the below formula:
- Surface Area = πr² +πr √h² + r²
- We can also write it as Surface Area = πr (r+√h² + r²)
Because radius, height, and Slant make the shape as right-angled Triangle. So, Using the Pythagoras theorem:
- l² = h² + r²
- l = √h² + r²
The Volume of a Cone
The amount of space inside the Cone is called Volume. If we know the radius and height of the Cone, then we can calculate the Volume using the formula:
- Volume = 1/3 πr²h (where h= height of a Cone)
- The Lateral Surface Area of a Cone = πrl
Java Program to find Volume and Surface Area of Cone
This Java program allows user to enter the value of a radius and height of a Cone. This Java program will calculate the Surface Area, Volume, length of a side (Slant), and Lateral Surface Area of a Cone as per the formulas.
// Java Program to find Volume and Surface Area of Cone package SurfaceAreaPrograms; import java.util.Scanner; public class VolumeOfCone { private static Scanner sc; public static void main(String[] args) { double radius, height; // LSA = Lateral Surface Area of a Cone, SA = Surface Area double length, SA, Volume, LSA; sc = new Scanner(System.in); System.out.println("\n Please Enter the Radius of a Cone : "); radius = sc.nextDouble(); System.out.println("\n Please Enter the Height of a Cone : "); height = sc.nextDouble(); length = Math.sqrt(radius * radius + height * height); SA = Math.PI * radius * (radius + length); Volume = (1.0/3) * Math.PI * radius * radius * height; LSA = Math.PI * radius * length; System.out.format("\n The Length of a Side (Slant)of a Cone = %.2f", length); System.out.format("\n The Surface area of a Cone = %.2f", SA); System.out.format("\n The Volume of a Cone = %.2f", Volume); System.out.format("\n The Lateral Surface area of a Cone = %.2f", LSA); } }
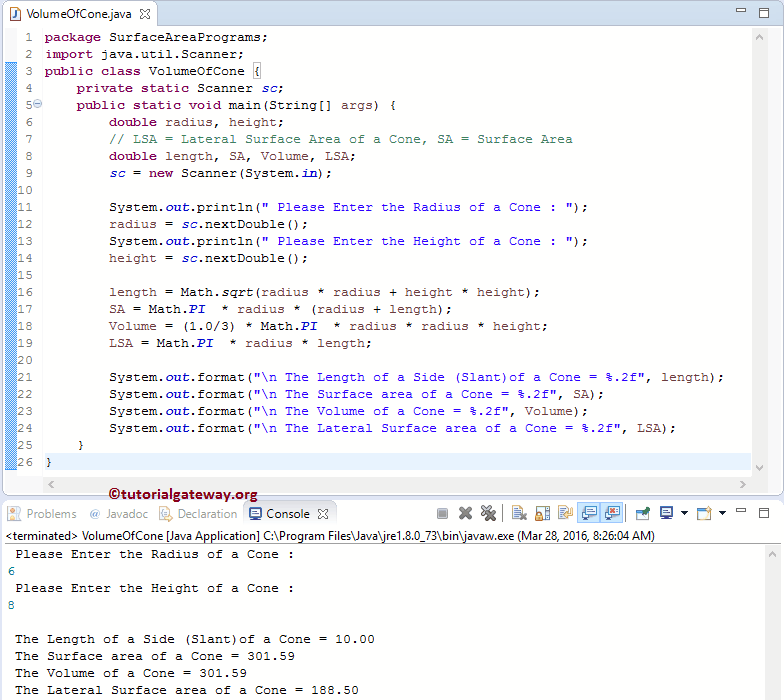
In this Java Program to find Volume and Surface Area of Cone, we are using the Mathematical Formula to calculate the Length of a Side (Slant) of a Cone
length = Math.sqrt(radius * radius + height * height);
Next, we are using the Mathematical Formula to calculate the surface area of a Cone
SA = Math.PI * radius * (radius + length);
In the next line, We are calculating the volume of a Cone
Volume = (1.0/3) * Math.PI * radius * radius * height;
In the next Java line, We are calculating the Lateral Surface Area of a Cone
LSA = Math.PI * radius * length;
The following System.out.format statements will help us to print the Volume of a Cone, Lateral Surface Area of a Cone, Length of a Side (Slant), and Surface Area of a Cone
System.out.format("\n The Length of a Side (Slant)of a Cone = %.2f", length); System.out.format("\n The Surface area of a Cone = %.2f", SA); System.out.format("\n The Volume of a Cone = %.2f", Volume); System.out.format("\n The Lateral Surface area of a Cone = %.2f", LSA);
From the above Java screenshot, you can observe that we have entered the Radius of a Cone = 6 and Height = 8
As per the Pythagoras theorem, We can calculate the Slant (Length of a side):
l² = h² + r²
l = √h² + r² ==> √8² + 6²
= √64 + 36 ==> √100
l = 10
The Surface Area of a Cone is
Surface Area of a Cone = πr² +πrl ==> πr (r + l)
Surface Area of a Cone = Math.PI * radius * (radius + l)
The Surface Area of a Cone = 3.14 * 6 * (6 +10)
Surface Area of a Cone = 301.44
The Volume of a Cone is
Volume of a Cone = 1/3 πr²h
Volume of a Cone = (1.0/3) * Math.PI * radius * radius * height
The Volume of a Cone = (1.0/3) * 3.14 * 6 * 6 * 8
Volume of a Cone = 301.44
The Lateral Surface Area of a Cone is
Lateral Surface Area = πrl
Lateral Surface Area = Math.PI * radius * l
The Lateral Surface Area = 3.14 * 6 * 10
Lateral Surface Area = 188.4
Let us calculate the Surface Area of a Cone using radius without using the Slant (Standard Formula):
Surface Area of a Cone = πr² +πr √h² + r² ==> πr (r + √h² + r²)
Surface Area = Math.PI * radius * (radius + sqrt ( (height * height) + (radius * radius)))
The Surface Area of a Cone = 3.14 * 6 * ( 6 + √8² + 6²)
Surface Area of a Cone = 3.14 * 6 * ( 6 + √100) ==> 3.14 * 6 * (16)
Surface Area of a Cone = 301.44
Java Program to find Volume and Surface Area of Cone using Functions
This Java program allows user to enter the value of a radius and height of a cone. By using these values, this Java program will calculate the Surface Area, Volume, length of a side (Slant), and Lateral Surface Area of a Cone as per the formulas. In this example, we are going to use the logic that we specified in the first example. However, we will separate the logic and place it in a method.
package SurfaceAreaPrograms; import java.util.Scanner; public class VolumeOfConeUsingMethods { private static Scanner sc; public static void main(String[] args) { double radius, height; sc = new Scanner(System.in); System.out.println(" Please Enter the Radius and Height of a Cone : "); radius = sc.nextDouble(); height = sc.nextDouble(); VolumeOfCone(radius, height); } public static void VolumeOfCone(double radius, double height) { double length, SA, Volume, LSA; length = Math.sqrt(radius * radius + height * height); SA = Math.PI * radius * (radius + length); Volume = (1.0/3) * Math.PI * radius * radius * height; LSA = Math.PI * radius * length; System.out.format(" The Length of a Side (Slant)of a Cone = %.2f", length); System.out.format("\n The Surface area of a Cone = %.2f", SA); System.out.format("\n The Volume of a Cone = %.2f", Volume); System.out.format("\n The Lateral Surface area of a Cone = %.2f", LSA); } }
Please Enter the Radius and Height of a Cone :
5
12
The Length of a Side (Slant)of a Cone = 13.00
The Surface area of a Cone = 282.74
The Volume of a Cone = 314.16
The Lateral Surface area of a Cone = 204.20
Java Program to find Volume and Surface Area of Cone using Oops
In this Java program, we are dividing the volumes and surface area of a cone code using the Object-Oriented Programming. To do this, we will create a class that holds methods.
package SurfaceAreaPrograms; public class VolumeOfaCone { double length, SA, Volume, LSA; public double LengthOfCone (double radius, double height) { length = Math.sqrt(radius * radius + height * height); return length; } public double VolumeOfCone (double radius, double height) { Volume = (1.0/3) * Math.PI * radius * radius * height; return Volume; } public double SurfaceAreaOfCone (double radius, double length) { SA = Math.PI * radius * (radius + length); return SA; } public double LateralSurfaceAreaOfCone(double radius, double length) { LSA = Math.PI * radius * length; return LSA; } }
Within the Main Java Program to find Volume and Surface Area of Cone program, we will create an instance of the above specified class and call the methods.
package SurfaceAreaPrograms; import java.util.Scanner; public class VolumeOfConeUsingClass { private static Scanner sc; public static void main(String[] args) { double radius, height; // LSA = Lateral Surface Area of a Cone, SA = Surface Area double length, SA, Volume, LSA; sc = new Scanner(System.in); System.out.println(" Please Enter the Radius and Height of a Cone : "); radius = sc.nextDouble(); height = sc.nextDouble(); VolumeOfaCone vc = new VolumeOfaCone(); length = vc.LengthOfCone(radius, height); SA = vc.SurfaceAreaOfCone(radius, length); Volume = vc.VolumeOfCone(radius, height); LSA = vc.LateralSurfaceAreaOfCone(radius, length); System.out.format(" The Length of a Side (Slant)of a Cone = %.2f", length); System.out.format(" The Surface area of a Cone = %.2f", SA); System.out.format(" The Volume of a Cone = %.2f", Volume); System.out.format(" The Lateral Surface area of a Cone = %.2f", LSA); } }
Please Enter the Radius and Height of a Cone :
6
11
The Length of a Side (Slant)of a Cone = 12.53
The Surface area of a Cone = 349.28
The Volume of a Cone = 414.69
The Lateral Surface area of a Cone = 236.18
VolumeofaCone Class Analysis:
- First, we declared a function LengthofCone with two arguments. Within the Java Program to find Volume and Surface Area of Cone function, we are finding the length of a side or Slant of a Cone and returning the value.
- Next, we declared a function VolumeofCone with two arguments. Within the function, we are calculating the Volume of a Cone and returning the value.
- Next, we declared a function SurfaceAreaofCone, with two arguments. Within the function, we are calculating the Surface Area of a Cone and returning the value.
- Then we declared a function LateralSurfaceAreaofCone with two arguments. Within the function, we are calculating the Lateral Surface Area of a Cone and returning the value.
Main Class Analysis:
In this Java Program to find Volume and Surface Area of Cone, First, we created an instance / created an Object of the VolumeofaCone Class
VolumeOfaCone vc = new VolumeOfaCone();
Next, we are calling the LengthofCone method by passing two arguments. It is the first method that we created with the double data type, and this method will calculate the length of a side and return a value. So, we are assigning the return value to the length variable.
length = vc.LengthOfCone(radius, height);
Next, we are calling the VolumeofCone method by passing two arguments. It is the second method that we created with the double data type, and this method will calculate the Volume of a Cone and return a value. So, we are assigning the return value to the volume variable.
Volume = vc.VolumeOfCone(radius, height);
Next, we are calling the SurfaceAreaofCone method. It is the third method that we created with the double data type, and this method will calculate the Surface Area of a Cone and return a value. So, we are assigning the return value to the SA variable.
SA = vcd.SurfaceAreaOfCuboid(length, width, height);
Next, we are calling the LateralSurfaceAreaofCone method. It is the fourth method that we created with the double data type, and this method will calculate the Lateral Surface Area of a Cone and return a value. So, we are assigning the return value to the LSA variable.
LSA = vc.LateralSurfaceAreaOfCone(radius, length);
Lastly, we used the following System.out.format statement to print the Length of a Side or Slant, Volume of a Cone, Lateral Surface Area of a Cone, and Surface Area of a Cone.
System.out.format(" The Length of a Side (Slant)of a Cone = %.2f", length); System.out.format("\n The Surface area of a Cone = %.2f", SA); System.out.format("\n The Volume of a Cone = %.2f", Volume); System.out.format("\n The Lateral Surface area of a Cone = %.2f", LSA);