Write a Java Program to Add Two Numbers and print the output. It is one of the fundamental concepts of calculations, and to achieve the same, we can use the arithmetic operator (+).
Java Program to Add Two Numbers Example
This simple Java program lets the user enter two integer values and then add those two numbers using the arithmetic operator (+) and assign them to a variable sum.
package SimpleNumberPrograms; import java.util.Scanner; public class addTwoNumbers { private static Scanner sc; public static void main(String[] args) { int Number1, Number2, Sum; sc = new Scanner(System.in); System.out.print("Please Enter the First integer Value: "); Number1 = sc.nextInt(); System.out.print("Please Enter the Second integer Value: "); Number2 = sc.nextInt(); Sum = Number1 + Number2; System.out.println("\nSum of the two integer values is = " + Sum); } }
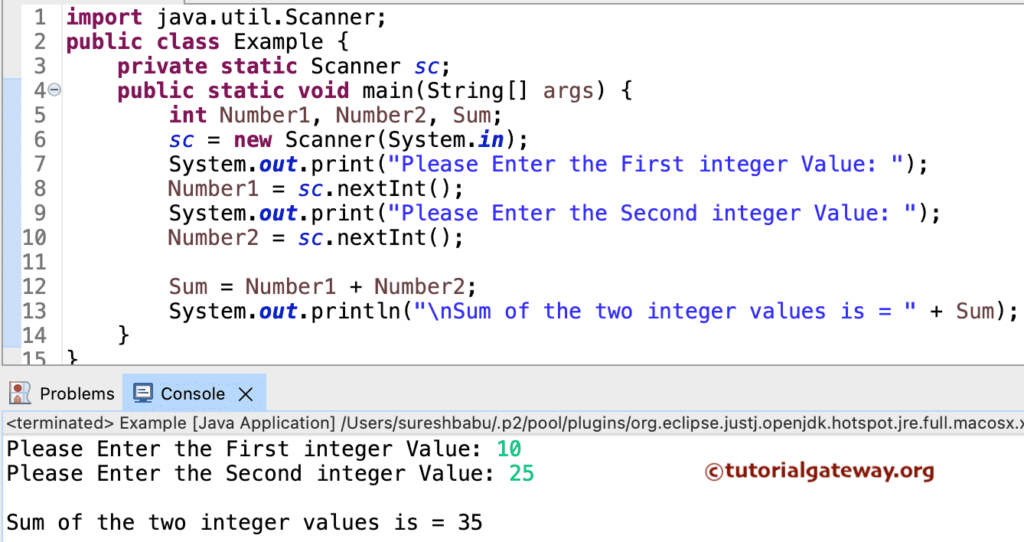
Within this Program, we used Arithmetic Operators + to add Number1 and Number2 and then assigned that total to Sum.
Sum = Number1 + Number2;
The following Java System.out.println statement will print the sum variable as output (10 + 25 = 35).
System.out.println("\nSum of the two integer values is = " + Sum);
Java Program to Add Two Numbers using Functions
In this Java program, we are using the same steps we followed in our first example. Still, we separated the Add Two Numbers logic and placed it in a separate method.
package SimpleNumberPrograms; import java.util.Scanner; public class addTwoNumbersMethod { private static Scanner sc; public static void main(String[] args) { int Number1, Number2, Sum; sc = new Scanner(System.in); System.out.println("Please Enter the First integer Value: "); Number1 = sc.nextInt(); //System.out.println("Please Enter the Second integer Value: "); Number2 = sc.nextInt(); Sum = add(Number1, Number2); System.out.println("\nSum of the two integer values is = " + Sum); } public static int add(int Number1,int Number2){ int Sum; Sum = Number1 + Number2; return Sum; } }
Output.
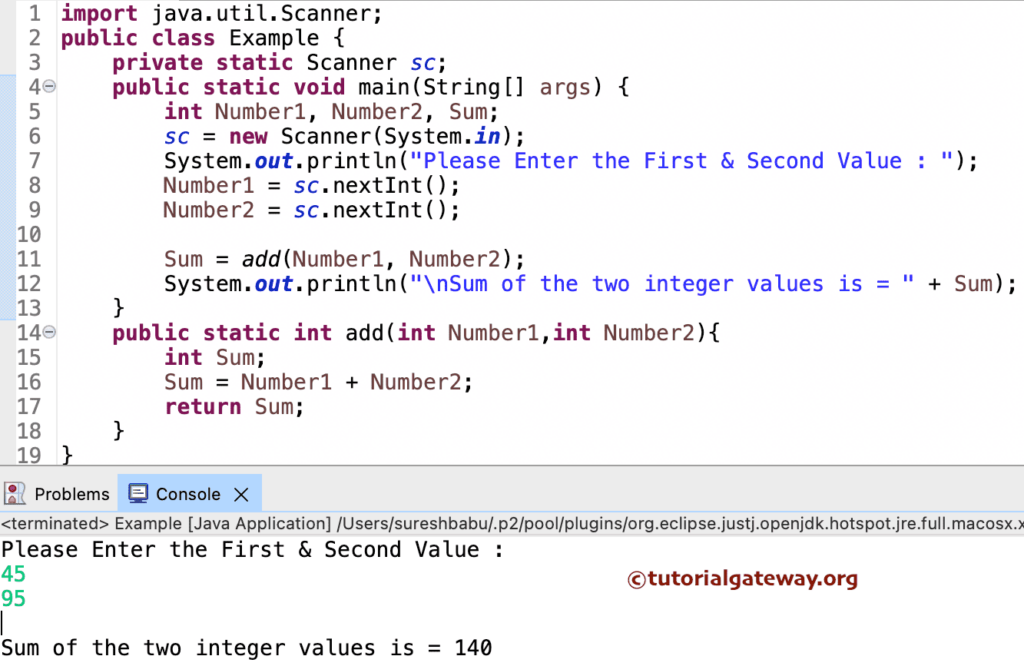
If you observe the following statement, we call the add method and assign the return value to the integer variable Sum.
Sum = add(Number1, Number2);
Let us see the code inside the add method.
public static int add(int Number1,int Number2){ int Sum; Sum = Number1 + Number2; return Sum; }
From the above code snippet, you can observe that this method accepts two integer type arguments.
- We declared an integer variable, Sum, to store the added value within the function.
- Next, we performed the arithmetic operation to add two integer numbers.
- Lastly, we are returning Sum.
Java Program to Add Two Numbers using OOPs
In this example, we are dividing the code further using Object Oriented Programming. First, we will create a class with two methods to do this.
TIP: In general, You don’t have to write the first method. We used this to show the available options.
package SimpleNumberPrograms; public class AddNumbers { int x, y, Sum; public void add(){ Sum = x + y; System.out.println("\n From Class: Sum of two integer values is = " + Sum); } public int addAgain(int Number1,int Number2){ Sum = Number1 + Number2; return Sum; } }
Within the Main Java Program to Add Two Numbers, we will create an instance of the above-specified class and call the methods.
// Calling AddNumbers Class package SimpleNumberPrograms; import java.util.Scanner; public class addTwoNumbersClass { private static Scanner sc; public static void main(String[] args) { int Number1, Number2, Sum; sc = new Scanner(System.in); System.out.println("\n Please Enter the First integer Value: "); Number1 = sc.nextInt(); System.out.println("\n Please Enter the Second integer Value: "); Number2 = sc.nextInt(); AddNumbers addNum = new AddNumbers(); addNum.x = Number1; addNum.y = Number2; addNum.add(); Sum = addNum.addAgain(Number1, Number2); System.out.println("\n Sum of the two integer values is = " + Sum); } }

Add Two Numbers in Java AddNumbers Class program Analysis:
- First, we declared a function add with zero arguments. We performed the arithmetic addition within the function and printed the Output using the System.out.println statement.
- Next, we declared an integer function addAgain with two arguments. We performed the arithmetic addition within the function and returned the integer value.
Main Class Analysis:
In the Java Program to Add Two Numbers main class, First, we created an instance of the AddNumbers Class
AddNumbers addNum = new AddNumbers();
Next, we assign the user-entered values to the AddNumbers Class variables.
addNum.x = Number1; addNum.y = Number2;
Next, we are calling the add method. Note that this method will add two numbers and print the output from the AddNumbers Class itself.
addNum.add();
Next, we are calling the addAgain method. Note that this is the second method with an integer data type.
Sum = addNum.addAgain(Number1, Number2);