The Java toDegrees Function is one of the Math Library functions which is used to convert an angle measured in radians to an approximately equivalent angle measured in degrees.
In this article, we will show how to use the toDegrees function with an example. The basic syntax of the Math.toDegrees in Java Programming language is as shown below.
static double toDegrees (double number); //Return Type is Double // In order to use in program: Math.toDegrees (double number);
Number: It can be an angle in radians that you want to convert as a degree.
Java toDegrees Function Example
The Java Math.toDegrees Function converts radians to an approximately equivalent angle measured in degrees. In this Java program, We are going to find the degrees of both positive and negative radian values and display the output.
package TrigonometricFunctions; public class ToDegrees { public static void main(String[] args) { double x = Math.toDegrees(4.96 + 2.65 - 4.98); System.out.println("toDegrees Function result = " + x); System.out.println("\ntoDegrees Function result of Zero = " + Math.toDegrees(0)); System.out.println("\ntoDegrees Function result of Positive Number = " + Math.toDegrees(1.95)); System.out.println("toDegrees Function result of Positive Number= " + Math.toDegrees(0.66)); System.out.println("\ntoDegrees Function result of Negative Number = " + Math.toDegrees(-1.85)); System.out.println("toDegrees Function result of Negative Number = " + Math.toDegrees(-2.35)); } }
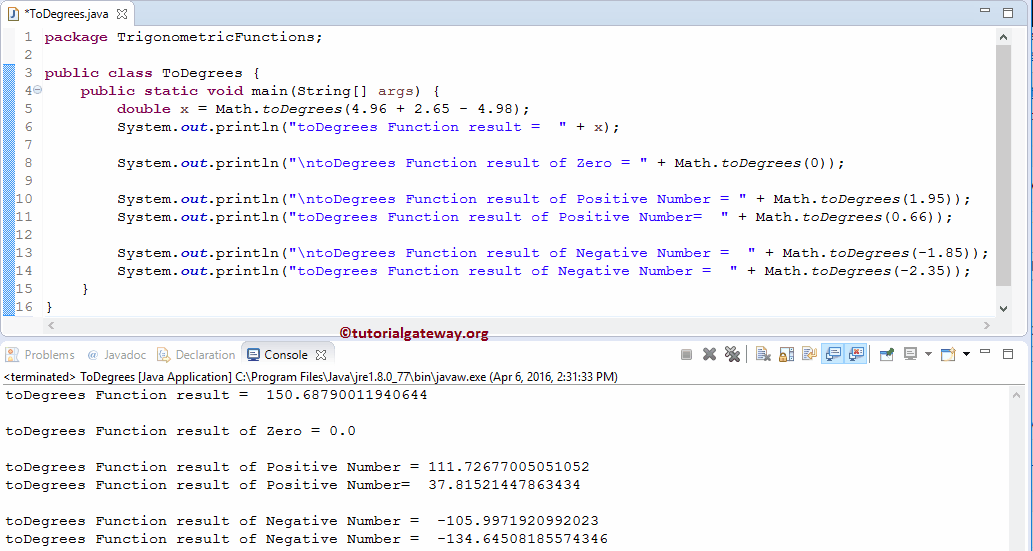
First, We declared a variable x of type Double and used the Java Math.toDegrees function directly on expression. Here, we used System.out.println statement to print the degrees as the output.
double x = Math.toDegrees(4.96 + 2.65 - 4.98); System.out.println("toDegrees Function result = " + x);
Next, We used this Function directly on the Positive double values.
System.out.println("\ntoDegrees Function result of Positive Number = " + Math.toDegrees(1.95)); System.out.println("toDegrees Function result of Positive Number= " + Math.toDegrees(0.66));
Next, We used this Math function directly on the Negative double values.
System.out.println("\ntoDegrees Function result of Negative Number = " + Math.toDegrees(-1.85)); System.out.println("toDegrees Function result of Negative Number = " + Math.toDegrees(-2.35));
Java toDegrees on Array example
In this Java program, we convert the bulk data to degrees. Here, we are going to declare an Array of double type and convert all the array elements into degrees.
package TrigonometricFunctions; public class ToDegreesOnArrays { public static void main(String[] args) { double [] myArray = {0.0175, 0.5236, 0.7854, 1.0472, 1.3090, 1.5708, 2.0944, 3.1416, 4.1888, 6.2832}; for (int i = 0; i < myArray.length; i++) { System.out.format("Degree of an Array Element %.4f is = %.2f\n", myArray[i], Math.toDegrees(myArray[i])); } } }
Degree of an Array Element 0.0175 is = 1.00
Degree of an Array Element 0.5236 is = 30.00
Degree of an Array Element 0.7854 is = 45.00
Degree of an Array Element 1.0472 is = 60.00
Degree of an Array Element 1.3090 is = 75.00
Degree of an Array Element 1.5708 is = 90.00
Degree of an Array Element 2.0944 is = 120.00
Degree of an Array Element 3.1416 is = 180.00
Degree of an Array Element 4.1888 is = 240.00
Degree of an Array Element 6.2832 is = 360.00
First, We declared a double Array and assigned some random radiant values.
Next, We used Java For Loop to iterate the Array. Within the For Loop, we initialized the i value as 0. Next, the compiler will check the condition (i < myArray.length). The statement inside the for loop will execute until the condition is False.
TIP: myArray.length finds the length of a given array.
for (int i = 0; i < myArray.length; i++) {
We used the Math Function directly inside the System.out.format statement. The Java compiler will call the Math method ( static double toDegrees(double number) ) to find the corresponding degree and print the same as an output.
System.out.format("Degree of an Array Element %.4f is = %.2f\n", myArray[i], Math.toDegrees(myArray[i]));
NOTE: To find the degree of a single item, then use the Math.toDegrees(myArray[index_position])
Java toDegrees Function on Arraylist example
In this toDegrees program, we are going to declare an ArrayList of double type and find the degrees of list elements.
package TrigonometricFunctions; import java.util.ArrayList; public class ToDegreesOnArrayList { public static void main(String[] args) { ArrayList<Double> myList = new ArrayList<Double>(5); myList.add(Math.PI); myList.add(Math.PI / 2); myList.add(Math.PI / 3); myList.add(Math.PI / 4); myList.add(Math.PI / 6); myList.add(Math.PI / 9); for (double x : myList) { System.out.format("result of ArrayList Item %.2f = %.4f \n", x, Math.toDegrees(x)); } } }
result of ArrayList Item 3.14 = 180.0000
result of ArrayList Item 1.57 = 90.0000
result of ArrayList Item 1.05 = 60.0000
result of ArrayList Item 0.79 = 45.0000
result of ArrayList Item 0.52 = 30.0000
result of ArrayList Item 0.35 = 20.0000
Within this example, We declared an ArrayList of double type and assigned random values using Math.PI constant (its value is approximately 3.14)
Next, We used Java For Loop to iterate the double values in ArrayList.
for (double x : myList) {
Here, the compiler will call the method ( static double toDegrees(double x) ) to find the corresponding degree and print the output.
System.out.format("toDegrees Function result of ArrayList Item %.2f = %.4f \n", x, Math.toDegrees(x));