The Java Array Fill Method is one of the Array Methods, which is to assign a user-specified value to every element in the specified range (if specified) of an array.
In this Java article, we will show how to fill the Boolean, Byte, Integer, Char, Long, Double, Float, Short, and Object array. The basic syntax of the Array fill in Java Programming language is as shown below.
Java Array Fill Method syntax
Java Programming Language provides eighteen different methods to fill the Array. The following fill method will accept the Byte Array and byte value as the parameter and assigns the byte value to every element in a Byte. Alternatively, we can say the Java fill method will replace all the array elements with user-specified byte values.
public static void fill(byte[] anByteArray, byte Value); //In order to use in program Arrays.fill(byte[] anByteArray, byte Value);
It accepts the Byte Array as the first argument, starting index position (fromIndex). The filling will begin as the second parameter (integer value), the last index position (toIndex). Where the filling will end as the third argument and byte value (Java array element replaced by this value).
NOTE: This method will fill the array elements starting from the fromIndex up to toIndex but not included.
public static void fill(byte[] anByteArray, int fromIndex, int toIndex, byte Value); //In order to use in program Arrays.fill(byte[] anByteArray, int fromIndex, int toIndex, byte Value);
This Java Fill method will accept the Boolean Array and value as the parameter and assigns the Boolean value to every element.
public static void fill(boolean[] anBooleanArray, boolean Value); //In order to use in program Arrays.fill(boolean[] anBooleanArray, boolean Value);
The following method will accept the Boolean Array as the first argument, starting index position (fromIndex) as the second parameter (integer value), the last index position (toIndex) as the third argument, and the Boolean value.
It accepts the Java Double Array as the first argument, starting index position (fromIndex) where the filling will begin as the second parameter (integer value), last index position (toIndex) where the filling will end as the third argument, and Double value (array element replaced by this value).
public static void fill(boolean[] anBooleanArray, int fromIndex, int toIndex, boolean Value); //In order to use in program Arrays.fill(boolean[] anBooleanArray, int fromIndex, int toIndex, boolean Value);
This Java Fill method accepts the short Array and short value as the parameter. It assigns the short value to every element in a short array.
public static void fill(short[] anShortArray, short Value); //In order to use in program Arrays.fill(short[] anShortArray, short Value);
Java fill method accepts the Short Array as the first argument, starting index position (fromIndex, last index position (toIndex), and Short value (element replaced by this value).
public static void fill(short[] anShortArray, int fromIndex, int toIndex, short Value); Arrays.fill(short[] anShortArray, int fromIndex, int toIndex, short Value); public static void fill(char[] anCharArray, char Value); Arrays.fill(char[] anCharArray, char Value); public static void fill(char[] anCharArray, int fromIndex, int toIndex, char Value); Arrays.fill(char[] anCharArray, int fromIndex, int toIndex, char Value); public static void fill(int[] anIntArray, int Value); Arrays.fill(int[] anIntArray, int Value); public static void fill(int[] anIntArray, int fromIndex, int toIndex, int Value); Arrays.fill(int[] anIntArray, int fromIndex, int toIndex, int Value); public static void fill(long[] anLongArray, long Value); Arrays.fill(long[] anLongArray, long Value); public static void fill(long[] anLongArray, int fromIndex, int toIndex, long Value); Arrays.fill(long[] anLongArray, int fromIndex, int toIndex, long Value); public static void fill(double[] anDoubleArray, double Value); Arrays.fill(double[] anDoubleArray, double Value); public static void fill(double[] anDoubleArray, int fromIndex, int toIndex, double Value); Arrays.fill(double[] anDoubleArray, int fromIndex, int toIndex, double Value); public static void fill(float[] anFloatArray, float Value); Arrays.fill(float[] anFloatArray, float Value); public static void fill(float[] anFloatArray, int fromIndex, int toIndex, float Value); Arrays.fill(float[] anFloatArray, int fromIndex, int toIndex, float Value); public static void fill(Object[] Array, Object Val); Arrays.fill(Object[] Array, Object Val); public static void fill(Object[] Array, int fromIndex, int toIndex, Object Val); Arrays.fill(Object[] Array, int fromIndex, int toIndex, Object Val);
- fromIndex: Please specify the starting index position. It is the index position where the Filling will begin.
- toIndex: Please specify the end index position. Java Arrays.fill method will fill up to this index position. However, it will not include the element at this position (toIndex).
- Value: Please specify the value you want to assign for every element of the Java Array.
Java fill Byte Array
In this Java program, we declare the byte array with random array elements. Then we will fill the array elements with a new value.
package ArrayMethods; import java.util.Arrays; public class ByteFill { public static void main(String[] args) { byte[] byteArray = {20, 8, 18, 45}; byte[] bitArray = {10, 25, 8, 19, 16}; //Filling Array with Value 5 Arrays.fill(byteArray, (byte)5); System.out.println("Filling Byte Array:"); arrayPrint(byteArray); //Filling Array with Value 7 using Index Arrays.fill(bitArray, 1, 4, (byte)7); System.out.println("Filling Byte Array Using Index:"); arrayPrint(bitArray); } public static void arrayPrint(byte[] anByteArray) { for (byte Number: anByteArray) { System.out.println("Array Elelment = " + Number); } } }
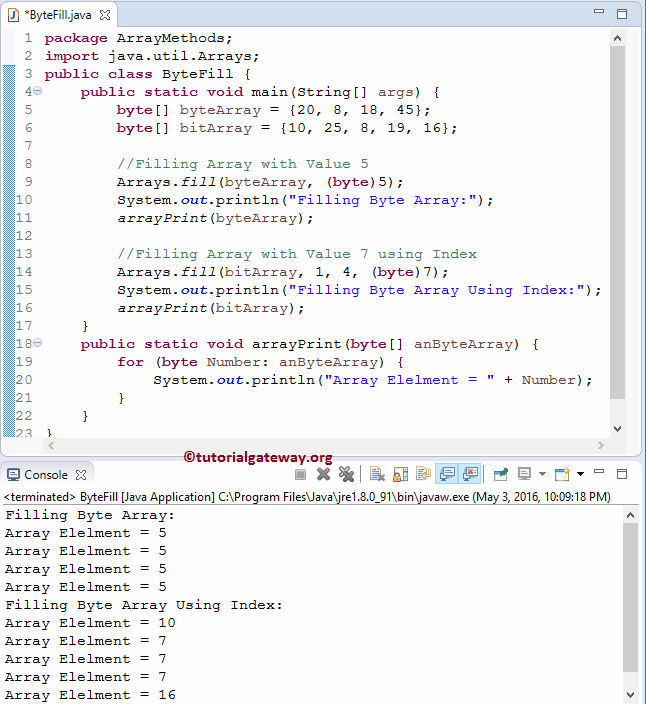
The following Java statement will call the public static void fill(byte[] anByteArray, byte Value) method to assign the Value to every element in a byte array.
Arrays.fill(byteArray, (byte)5);
The following Java statement is to print the Byte array elements to the output.
arrayPrint(byteArray);
When the compiler reaches the above statement, the compiler will jump to the following function. We used the Java Foreach Loop from the code snippet to iterate the Byte Array. Then we print every array element using the System.out.println statement.
public static void arrayPrint(byte[] anByteArray) { for (byte Number: anByteArray) { System.out.println("Array Elelment = " + Number); } }
The following Java statement calls public static void fill(byte[] anByteArray, int fromIndex, int toIndex, byte Value) to fill the byte array from index position 1 to position 3 with value 7.
Arrays.fill(bitArray, 1, 4, (byte)7);
Java fill Boolean Array
In this Java fill program, we declared the Boolean array with random elements. Then we will fill the array elements with a new value.
package ArrayMethods; import java.util.Arrays; public class BooleanFill { public static void main(String[] args) { boolean[] bool = {true, false, false, true}; boolean[] boolArray = {true, false, true, true, true}; Arrays.fill(bool, true); System.out.println("Boolean Array:"); arrayPrint(bool); Arrays.fill(boolArray, 1, 4, false); System.out.println("Filling Boolean Array Using Index:"); arrayPrint(boolArray); } public static void arrayPrint(boolean[] anBooleanArray) { for (boolean bool: anBooleanArray) { System.out.println("Array Elelment = " + bool); } } }
Boolean Array:
Array Elelment = true
Array Elelment = true
Array Elelment = true
Array Elelment = true
Filling Boolean Array Using Index:
Array Elelment = true
Array Elelment = false
Array Elelment = false
Array Elelment = false
Array Elelment = true
The following statement will call the public static void fill(boolean[] anBooleanArray, Boolean Value) method to assign the Value to every element in a Boolean array.
Arrays.fill(bool, true);
It calls the public static void fill(boolean[] anBooleanArray, int fromIndex, int toIndex, Boolean Value) method to fill the Boolean array from index position 1 to position 3 with Boolean False.
Arrays.fill(boolArray, 1, 4, false);
Java fill Short Array
In this Java fill program, we declared the Short array with random elements. Then we will Sort the short array elements in Ascending Order.
package ArrayMethods; import java.util.Arrays; public class ShortFill { public static void main(String[] args) { short[] shrArray = {12, 14, 2, 9}; short[] ShortArray = {25, 5, 19, 6, 50}; Arrays.fill(shrArray, (short)15); System.out.println("Filling Short Array:"); arrayPrint(shrArray); Arrays.fill(ShortArray, 1, 4,(short)75); System.out.println("Filling Short Array Using Index:"); arrayPrint(ShortArray); } public static void arrayPrint(short[] anShortArray) { for (short Number: anShortArray) { System.out.println("Array Elelment = " + Number); } } }
Filling Short Array:
Array Elelment = 15
Array Elelment = 15
Array Elelment = 15
Array Elelment = 15
Filling Short Array Using Index:
Array Elelment = 25
Array Elelment = 75
Array Elelment = 75
Array Elelment = 75
Array Elelment = 50
It calls the public static void fill(short[] anShortArray, short Value) method to assign the Value to every element in a Short array.
Arrays.fill(shrArray, (short)15);
It calls public static void fill(short[] anShortArray, int fromIndex, int toIndex, short Value) to fill the Short array from index position 1 to position 3 with 75.
Arrays.fill(ShortArray, 1, 4,(short)75);
Java fill Method on Integer Array
In this program, we declared the Integer array with random elements. Then we will fill the Int array elements with a new value.
package ArrayMethods; import java.util.Arrays; public class IntFill { public static void main(String[] args) { int[] IntArray = {98, 54, 75, 82}; int[] IntegerArray = {14, 25, 78, 19, 94}; Arrays.fill(IntArray, 30); System.out.println("Integer Array:"); arrayPrint(IntArray); Arrays.fill(IntegerArray, 1, 4, 50); System.out.println("Filling Integer Array Using Index:"); arrayPrint(IntegerArray); } public static void arrayPrint(int[] anIntArray) { for (int Number: anIntArray) { System.out.println("Array Element = " + Number); } } }
Integer Array:
Array Element = 30
Array Element = 30
Array Element = 30
Array Element = 30
Filling Integer Array Using Index:
Array Element = 14
Array Element = 50
Array Element = 50
Array Element = 50
Array Element = 94
It calls the public static void fill(int[] anIntegerArray, int Value) method to assign the Value to every element in an Integer array.
Arrays.fill(IntArray, 30);
It calls the public static void fill(int[] anIntegerArray, int fromIndex, int toIndex, int Value) method to fill the integer array from index position 1 to position 3 with 50.
Arrays.fill(IntegerArray, 1, 4, 50);
Long Array using Java fill Method
In this program, we declared the Long array with random elements. Then we will fill the Long array of elements with new values.
package ArrayMethods; import java.util.Arrays; public class LongFill { public static void main(String[] args) { long[] LngArray = {198, 146, 187, 153}; long[] LongArray = {1515, 250, 190, 80, 2525}; Arrays.fill(LngArray, 750); System.out.println("Filling Long Array:"); arrayPrint(LngArray); Arrays.fill(LongArray, 1, 4, 1500); System.out.println("Filling Long Array Using Index:"); arrayPrint(LongArray); } public static void arrayPrint(long[] anLongArray) { for (long Number: anLongArray) { System.out.println(Number); } } }
Filling Long Array:
750
750
750
750
Filling Long Array Using Index:
1515
1500
1500
1500
2525
In this example, the below statement calls the public static void fill(long[] anLongArray, long Value) to assign the Value to every Long array element.
Arrays.fill(LngArray, 750);
The following statement calls public static void fill(long[] anLongArray, int fromIndex, int toIndex, long Value) to fill the Long array from index position 1 to position 3 with a value of 1500.
Arrays.fill(LongArray, 1, 4, 1500);
Java fill Method on Double Array
In this Java program, we declared the Double array with random array elements. Then we will fill the Double array elements with a new value.
package ArrayMethods; import java.util.Arrays; public class DoubleFill { public static void main(String[] args) { double[] DoubArray = {64.542, 7.98, 78.45, 35.76}; double[] DoubleArray = {145.25, 98.86, 27.9865, 83.485, 209.356}; Arrays.fill(DoubArray, 1400.25); System.out.println("Double Array:"); arrayPrint(DoubArray); Arrays.fill(DoubleArray, 1, 4, 180.75); System.out.println("Filling Double Array Using Index:"); arrayPrint(DoubleArray); } public static void arrayPrint(double[] anDoubleArray) { for (double Number: anDoubleArray) { System.out.println(Number); } } }
Double Array:
1400.25
1400.25
1400.25
1400.25
Filling Double Array Using Index:
145.25
180.75
180.75
180.75
209.356
It calls the public static void fill(double[] anDoubleArray, double Value) method to assign the Value to every element in a double array.
Arrays.fill(DoubArray, 1400.25);
The following statement calls public static void fill(double[] anDoubleArray, int fromIndex, int toIndex, double Value) to sort the Double array from index position 1 to position 3 with 180.75.
Arrays.fill(DoubleArray, 1, 4, 180.75);
Java fill Method on Float Array
In this Java program, we declared the Float array with random elements. Next, we will fill the Float array elements with the new values.
package ArrayMethods; import java.util.Arrays; public class FloatFill { public static void main(String[] args) { float[] floArray = {8.68f, 1.98f, 5.54f, 12.45f}; float[] floatArray = {8.4f, 10.25f, 25.98f, 16.48f, 13.3f}; //Filling Array with Value 10.25 Arrays.fill(floArray, 10.25f); System.out.println("Float Array:"); arrayPrint(floArray); //Filling Array with Value 25.75 using Index Arrays.fill(floatArray, 1, 4, 25.75f); System.out.println("Filling Float Array Using Index:"); arrayPrint(floatArray); } public static void arrayPrint(float[] anfloatArray) { for (float Number: anfloatArray) { System.out.println(Number); } } }
Float Array:
10.25
10.25
10.25
10.25
Filling Float Array Using Index:
8.4
25.75
25.75
25.75
13.3
The following statement will call the public static void fill(float[] anFloatArray, float Value) method to assign the Value to every element in a floating-point array.
Arrays.fill(floArray, 10.25f);
It calls the public static void fill(float[] anFloatArray, int fromIndex, int toIndex, float Value) method to fill the Float array from index position 1 to position 3 with 25.75f.
Arrays.fill(floatArray, 1, 4, 25.75f);
Java fill Method on Char Array
In this program, we declared the Character array with random elements. Then we will fill the Char array elements with a new value.
package ArrayMethods; import java.util.Arrays; public class CharFill { public static void main(String[] args) { char[] CharArray = {'g', 'a', 't', 'e'}; char[] CharacterArray = {'g', 'a', 't', 'e', 'y'}; //Filling Array with Char T Arrays.fill(CharArray, 'T'); System.out.println("Character Array:"); arrayPrint(CharArray); //Filling Array with Character A using Index Arrays.fill(CharacterArray, 2, 4, 'A'); System.out.println("Filling Character Array Using Index:"); arrayPrint(CharacterArray); } public static void arrayPrint(char[] anCharacterArray) { for (char ch: anCharacterArray) { System.out.println(ch); } } }
Character Array:
T
T
T
T
Filling Character Array Using Index:
g
a
A
A
y
In this example, the following statement will call the public static void fill(char [] anCharArray, char Value) method to assign the Value to each and every element in a char array.
Arrays.fill(CharArray, 'T');
The below statement will call the (char[] anCharArray, int fromIndex, int toIndex, char Value) to fill the Char array from index position 2 to position 3 with the character ‘A’.
Arrays.fill(CharacterArray, 2, 4, 'A');
Java fill Method on Object Array
In this Java program, we declare the String array with random elements. Next, we will fill the string object array elements with new object values.
package ArrayMethods; import java.util.Arrays; public class ObjectFill { public static void main(String[] args) { String[] strArray = {"Apple", "Orange", "Kiwi"}; String[] stringArray = {"India", "UK", "Australia", "Japan","China"}; Arrays.fill(strArray, "Cherry"); System.out.println("Filling Object Array:"); arrayPrint(strArray); Arrays.fill(stringArray, 1, 4, "USA"); System.out.println("Filling Object Array Using Index:"); arrayPrint(stringArray); } public static void arrayPrint(Object[] anObjectArray) { for (Object Number: anObjectArray) { System.out.println(Number); } } }
Filling Object Array:
Cherry
Cherry
Cherry
Filling Object Array Using Index:
India
USA
USA
USA
China
Within this Java Method example, the following statement calls public static void fill(Object[] anObjectArray, Object Value) to assign the Value to every Object array element.
Arrays.fill(strArray, "Cherry");
It calls public static void fill(Object[] anObjectArray, int fromIndex, int toIndex, Object Value). And the method fills the Object array from index position 1 to position 3 with the string “USA”.
Arrays.fill(stringArray, 1, 4, "USA");