The Java endsWith method is one of the String Methods to check whether the string is ending with a user-specified substring or not. The endsWith method will return Boolean True or False based on the result. In this article, we will show how to write string endsWith in Java Programming language with an example.
Java endsWith Syntax
The following Java endsWith method will accept the substring as the argument and check whether the string is ending with this substring or not.
public boolean endsWIth(String suffix); // It will return boolean True or False //In order to use in program String_Object.endsWIth(String suffix)
If we specify the Suffix as an empty string, the Java endsWith String function returns Boolean True as output.
Java endsWith Example
In this program, we will use the endsWith method to check whether the string ends with a user-specified substring.
The first statement will call the Java public boolean endsWith (String suffix) method to check whether the string str is ending with ing. If it is TRUE, it will return TRUE; otherwise, False.
The following statement will check whether the str1 is ending with the company. If it is TRUE, it will return TRUE; otherwise, False.
Let us try the wrong value for d. The following statement will call the public boolean endsWith (String suffix) method to check whether the str1 is ending with abc. We all know that it is False.
The following System.out.println statements will print the output.
package StringFunctions; public class EndsWithMethod { public static void main(String[] args) { String str = "Tutorials On Java Programming"; String str1 = "We are abc working at abc company"; boolean a = str.endsWith("ing"); boolean b = str.endsWith("Programming"); boolean c = str1.endsWith("company"); boolean d = str1.endsWith("abc"); System.out.println("Does the String str ends with ing? = " + a); System.out.println("Does the String str ends with Programming? = " + b); System.out.println("Does the String str1 ends with company? = " + c); System.out.println("Does the String str1 ends with abc? = " + d); } }
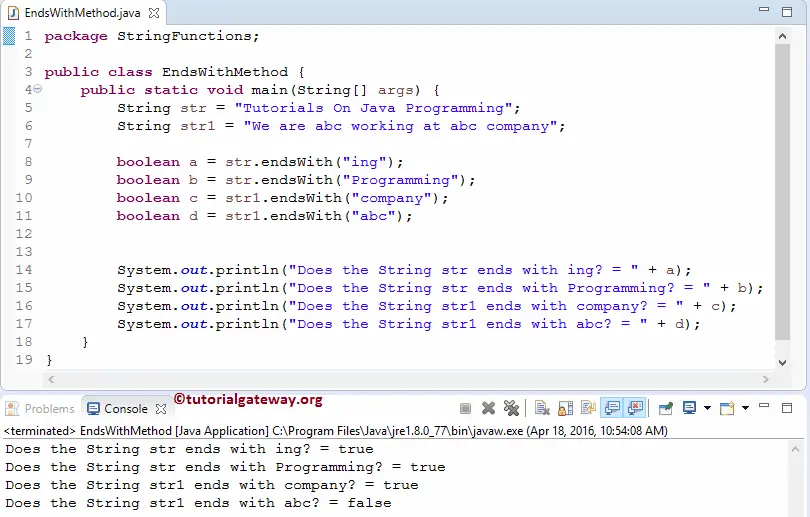
Java endsWith Example
In this Java program, we will ask the user to enter any word. Based on the user entered string value, the endswith program will display the message.
Within this String Method example, the first statement will ask the user to enter any word. Then we will assign the user entered value to the string variable str.
Next, we used the If Else Statement to check whether the user entered a string ending with “java” or not.
- If the condition is True, the Java System.out.println(“Hey!! Welcome to Tutorials”); statement will be printed.
- Else System.out.println(“Goodbye to Programming”); statement will be printed.
package StringFunctions; import java.util.Scanner; public class EndsWithMethod2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.println("Please Enter any word: "); String str = sc.nextLine(); if (str.endsWith("java")) { System.out.println("Hey!! Welcome to Tutorials"); } else { System.out.println("Goodbye to Programming"); } } }
Please Enter any word:
hello java
Hey!! Welcome to Tutorials
Let us enter a different word.
Please Enter any word:
hi friends
Goodbye to Programming