The Java Arrays.copyOfRange Method is one of the Java Array Methods, which is to copy the array items within the specified user range into a new array.
In this article, we will show how to use the Java copyOfRange Method to copy the range of Array elements to a new array with an example. The syntax of the Arrays.copyOfRange in Java Programming language is as shown below.
Java Arrays.copyOfRange Method syntax
The Java Programming Language provides ten different Arrays.copyOfRange methods to copy the specified Arrays to a New one. While coping:
- If the specified user length is greater than the Original Array, then the remaining elements are filled with default values of the data type. For example, array a[5] of integer type holds five elements (1,2 3, 4, 5), but we specified the new length as 7 (Arrays.copyOfRange(b, 0, 7), then b array will become b[7] holding elements 1, 2, 3, 4, 5, 0, 0 (Here, 0 is the default value for int type).
- If the user-specified to (third argument) is less than the Original Array, then the remaining elements are truncated. For example, array a[7] of integer type holds five elements (15, 22, 10, 45, 3, 65, 54), but we specified the new length as 5 (Arrays.copyOfRange(b, 1, 4), then b array become b[3] holding elements 22, 10, 45.
The Following Java Arrays.copyOfRange method accepts the Boolean Array, starting index position (from) where the elements will start coping as the second parameter (integer value). And the last index position (to) where the coping will end as the third argument.
NOTE: This method will copy the array elements starting from the from up to but not included.
public static boolean copyOfRange(Boolean[] anBooleanArray, int from, int to); // It will return Boolean Array //In order to use in program Arrays.copyOfRange(Boolean[] anBooleanArray, int from, int to);
The remaining Java arrays.copyOfRange methods.
public static byte copyOfRange(byte[] anByteArray, int from, int to); // It will return Byte Array Arrays.copyOfRange(byte[] anByteArray, int from, int to); public static short copyOfRange(short[] anShortArray, int from, int to); // It will return short Array Arrays.copyOfRange(short[] anShortArray, int from, int to); public static char copyOfRange(char[] anCharArray, int from, int to); // It will return char Array Arrays.copyOfRange(char[] anCharArray, int from, int to); public static short copyOfRange(int[] anIntArray, int from, int to); // It will return Integer Array Arrays.copyOfRange(int[] anIntArray, int from, int to); public static long copyOfRange(long[] anLongArray, int from, int to); // It will return Long Array Arrays.copyOfRange(long[] anLongArray, int from, int to); public static double copyOfRange(double[] anDoubleArray, int from, int to); // It will return Double Array Arrays.copyOfRange(double[] anDoubleArray, int from, int to); public static float copyOfRange(float[] anFloatArray, int from, int to); // It will return float Array Arrays.copyOfRange(float[] anFloatArray, int from, int to); public static <T> T[] copyOfRange(T[] Array, int from, int to); Arrays.copyOfRange(T[] Array, int from, int to); public static <T,U> T[] copyOfRange(U[] Array, int from, int to, Class<? extends T[]> newType); Arrays.copyOfRange(U[] Array, int from, int to, Class<? extends T[]> newType);
- Array: This is the Original Array. We are going to use the Arrays.copyOfRange method to copy this array to a new array.
- from: Please specify the starting position from where the elements will start copy into a new array.
- to: Please specify the end position. The copyOfRange method will copy array elements up to this index position but not be included them in a new one. Based on the to, array elements truncated or extended (with default values)
- newType: Please specify the class type to apply for a new array.
Java Arrays.copyOfRange Byte Array
This Java program declares the byte array with random array elements. Next, we will call the public static byte[] copyOfRange (byte[] anByteArray, int from, int to) method to copy the Byte array to a new one of the specified range (from-to).
// Java Example to Copy Byte Array to New package ArrayMethods; import java.util.Arrays; public class CopyOfRangeBytes { public static void main(String[] args) { byte[] byteArray = {10, 25, 8, 19, 16, 5}; //Copying Array byte[] bitArray = Arrays.copyOfRange(byteArray, 1, 5); System.out.println("New Integer Array after Copying:"); arrayPrint(bitArray); //Copying Array byte[] biteArray = Arrays.copyOfRange(byteArray, 3, 9); System.out.println("New Integer Array after Copying:"); arrayPrint(biteArray); } public static void arrayPrint(byte[] anByteArray) { for (byte Number: anByteArray) { System.out.println("Array Elelment = " + Number); } } }
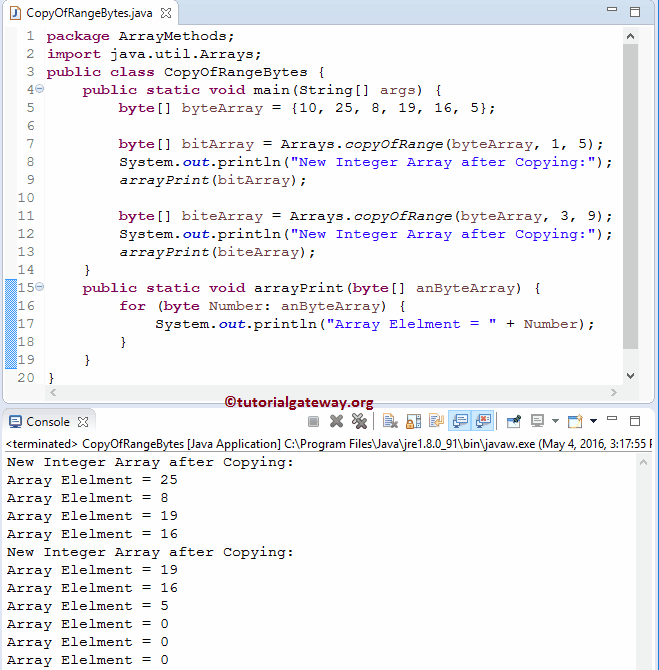
Within this example, we declared a byte Array and assigned some random values as the array elements using the following statement.
byte[] byteArray = {10, 25, 8, 19, 16, 5};
Next, we used the Java Arrays.copyOfRange method to copy the bitArray elements from index position 1 to 4.
byte[] bitArray = Arrays.copyOfRange(byteArray, 1, 5);
The following statement is to print the Byte array elements to the output.
arrayPrint(bitArray);
Next line, we used the Arrays.copyOfRange method to copy the byteArray elements from index position 3 to 5. It means the remaining values will fill with default values (zeros).
byte[] biteArray = Arrays.copyOfRange(byteArray, 3, 9);
The following statement is to print the Byte array elements to the output
arrayPrint(byteArray);
When the compiler reaches the above statement, the Jcompiler will jump to the following function.
From the below code snippet, observe that we used the Java Foreach Loop to iterate the Byte Array. Then we are printing every array element using the System.out.println statement.
public static void arrayPrint(byte[] anByteArray) { for (byte Number: anByteArray) { System.out.println("Array Elelment = " + Number); } }
Java Arrays.copyOfRange to copy Boolean Array
In this Java program, we declare the Boolean array with random elements. Then we will call the public static Boolean[] copyOfRange (boolean[] anBooleanArray, int from, int to) method to copy the Boolean array to a new one of the specified range.
package ArrayMethods; import java.util.Arrays; public class CopyOfRangeBoolean { public static void main(String[] args) { boolean[] bool = {true, false, false, true, true}; boolean[] boolArray = Arrays.copyOfRange(bool, 1, 4); System.out.println("New Boolean Array after Copying:"); arrayPrint(boolArray); boolean[] bolArray = Arrays.copyOfRange(bool, 2, 7); System.out.println("New Boolean Array with Vlaues:"); arrayPrint(bolArray); } public static void arrayPrint(boolean[] anBooleanArray) { for (boolean bool: anBooleanArray) { System.out.println("Array Elelment = " + bool); } } }
New Boolean Array after Copying:
Array Elelment = false
Array Elelment = false
Array Elelment = true
New Boolean Array with Vlaues:
Array Elelment = false
Array Elelment = true
Array Elelment = true
Array Elelment = false
Array Elelment = false
Java Arrays.copyOfRange to copy Short Array
In this program, we declared the short array with random array elements. Then we call the public static Short[] copyOfRange (short[] anShortArray, int from, int to) method to copy the short array to a new one of the specified range.
package ArrayMethods; import java.util.Arrays; public class ShortCopyOfRange { public static void main(String[] args) { short[] srtArray = {2, 6, 4, 8, 9, 7, 3}; short[] ShortArray = Arrays.copyOfRange(srtArray, 0, 4); System.out.println("New Short Array after Copying:"); arrayPrint(ShortArray); } public static void arrayPrint(short[] anShortArray) { for (short Number: anShortArray) { System.out.println("Array Elelment = " + Number); } } }
New Short Array after Copying:
Array Elelment = 2
Array Elelment = 6
Array Elelment = 4
Array Elelment = 8
Java Arrays.copyOfRange to copy Integer Array
In this program, we declare the integer array with random elements. Then we call the public static int[] copyOfRange (int[] anIntegerArray, int from, int to) method to copy the Int array to a new array of the specified range.
package ArrayMethods; import java.util.Arrays; public class CopyOfRangeIntegers { public static void main(String[] args) { int[] intArray = {20, 40, 60, 80, 100, 120, 150}; int[] integerArray = Arrays.copyOfRange(intArray, 2, 6); System.out.println("New Integer Array after Copying:"); arrayPrint(integerArray); int[] inteArray = Arrays.copyOfRange(intArray, 4, 9); System.out.println("New Integer Array after Copying:"); arrayPrint(inteArray); } public static void arrayPrint(int[] anIntArray) { for (int Number: anIntArray) { System.out.println("Array Elelment = " + Number); } } }
New Integer Array after Copying:
Array Elelment = 60
Array Elelment = 80
Array Elelment = 100
Array Elelment = 120
New Integer Array after Copying:
Array Elelment = 100
Array Elelment = 120
Array Elelment = 150
Array Elelment = 0
Array Elelment = 0
Java Arrays.copyOfRange to copy Long Array
In this program, we declared the long array with random elements. Then we call the public static Long[] copyOfRange (long[] anLongArray, int from, int to) method to copy the Long array to a new array of the specified range.
package ArrayMethods; import java.util.Arrays; public class CopyOfRangeLong { public static void main(String[] args) { long[] LngArray = {540, 605, 1250, 2250, 3590, 9865}; long[] longArray = Arrays.copyOfRange(LngArray, 1, 4); System.out.println("New Long Array after Copying:"); arrayPrint(longArray); long[] anLongArray = Arrays.copyOfRange(LngArray, 2, 8); System.out.println("New Long Array with Vlaues:"); arrayPrint(anLongArray); } public static void arrayPrint(long[] anLongArray) { for (long Number: anLongArray) { System.out.println("Array Elelment = " + Number); } } }
New Long Array after Copying:
Array Elelment = 605
Array Elelment = 1250
Array Elelment = 2250
New Long Array with Vlaues:
Array Elelment = 1250
Array Elelment = 2250
Array Elelment = 3590
Array Elelment = 9865
Array Elelment = 0
Array Elelment = 0
Java Arrays.copyOfRange to copy Double Array
In this program, we declared the double array with random elements. Then we call the public static Double[] copyOfRange (double[] anDoubleArray, int from, int to) method to copy the double array to a new one of the specified range.
package ArrayMethods; import java.util.Arrays; public class CopyOfRangeDoubles { public static void main(String[] args) { double[] DoubleArray = {143.96, 346.452, 67.89, 897.559, 655.689}; double[] dblArray = Arrays.copyOfRange(DoubleArray, 1, 5); System.out.println("New Double Array after Copying:"); arrayPrint(dblArray); double[] dbArray = Arrays.copyOfRange(DoubleArray, 2, 7); System.out.println("New Double Array after Copying:"); arrayPrint(dbArray); } public static void arrayPrint(double[] anDoubleArray) { for (double Number: anDoubleArray) { System.out.println("Array Elelment = " + Number); } } }
New Double Array after Copying:
Array Elelment = 346.452
Array Elelment = 67.89
Array Elelment = 897.559
Array Elelment = 655.689
New Double Array after Copying:
Array Elelment = 67.89
Array Elelment = 897.559
Array Elelment = 655.689
Array Elelment = 0.0
Array Elelment = 0.0
Java Arrays.copyOfRange to copy Float Array
In this program, we declared the floating-point array with random elements. Then we call the public static float[] copyOfRange (float[] anFloatArray, int from, int to) method to copy the float array to a new array of the specified range.
package ArrayMethods; import java.util.Arrays; public class CopyOfFloat { public static void main(String[] args) { float[] floatArray = {24.54f, 33.98f, 46.68f, 19.25f, 3.95f}; float[] fltArray = Arrays.copyOfRange(floatArray, 1, 4); System.out.println("New Float Array after Copying:"); arrayPrint(fltArray); float[] ftArray = Arrays.copyOfRange(floatArray, 3, 7); System.out.println("New Float Array with Changed Vlaues:"); arrayPrint(ftArray); } public static void arrayPrint(float[] anfloatArray) { for (float Number: anfloatArray) { System.out.println("Array Elelment = " + Number); } } }
New Float Array after Copying:
Array Elelment = 33.98
Array Elelment = 46.68
Array Elelment = 19.25
New Float Array with Changed Vlaues:
Array Elelment = 19.25
Array Elelment = 3.95
Array Elelment = 0.0
Array Elelment = 0.0
Java Arrays.copyOfRange to copy Char Array
In this program, we declared the character array. Then we call public static Char[] copyofRange (char[] anCharArray, int from, int to) method to copy the Char array to a new character array of a specified range.
package ArrayMethods; import java.util.Arrays; public class CopyOfRangeChar { public static void main(String[] args) { char[] CharArray = {'T', 'u', 't', 'o', 'r', 'i', 'a', 'l'}; char[] ChArray = Arrays.copyOfRange(CharArray, 1, 5); System.out.println("New Character Array after Copying:"); arrayPrint(ChArray); char[] ChrArray = Arrays.copyOfRange(CharArray, 4, 10); System.out.println("New Character Array with Vlaues:"); arrayPrint(ChrArray); } public static void arrayPrint(char[] anCharacterArray) { for (char Number: anCharacterArray) { System.out.println("Array Elelment = " + Number); } } }
New Character Array after Copying:
Array Elelment = u
Array Elelment = t
Array Elelment = o
Array Elelment = r
New Character Array with Vlaues:
Array Elelment = r
Array Elelment = i
Array Elelment = a
Array Elelment = l
Array Elelment =
Array Elelment =
Java Arrays.copyOfRange to copy Object Array
In this program, we declared the String array (string is an Object) with random elements. Then we call public static T[] copyOfRange(T[] anArray, int from, int to) method to copy the Object array to a new array of the specified range.
package ArrayMethods; import java.util.Arrays; public class CopyOfRangeObject { public static void main(String[] args) { String[] stringArray = {"India", "USA", "UK", "Japan", "China", "Canada"}; String[] strArray = Arrays.copyOfRange(stringArray, 1, 5); System.out.println("New Object Array after Copying:"); arrayPrint(strArray); String[] strngArray = Arrays.copyOfRange(stringArray, 2, 8); System.out.println("New Object Array with Vlaues:"); arrayPrint(strngArray); } public static void arrayPrint(Object[] anObjectArray) { for (Object Number: anObjectArray) { System.out.println("Array Elelment = " + Number); } } }
New Object Array after Copying:
Array Elelment = USA
Array Elelment = UK
Array Elelment = Japan
Array Elelment = China
New Object Array with Vlaues:
Array Elelment = UK
Array Elelment = Japan
Array Elelment = China
Array Elelment = Canada
Array Elelment = null
Array Elelment = null
Java Arrays.copyOfRange to copy Array to User specified type
In this Java program, we declared the Object. Then we call the Java public static <T, U> T[] copyOfRange (U[] anObjectArray, int from, int to) method to copy the Object array to new specified array.
package ArrayMethods; import java.util.Arrays; public class CopyOfRangeT { public static void main(String[] args) { Object[] objectArray = {"Kiwi", "Apple", "Bannana", "Grape", "Cherry"}; String[] objArray = Arrays.copyOfRange(objectArray, 1, 4, String[].class); System.out.println("New Object Array after Copying:"); arrayPrint(objArray); } public static void arrayPrint(Object[] anObjectArray) { for (Object Number: anObjectArray) { System.out.println("Array Elelment = " + Number); } } }
New Object Array after Copying:
Array Elelment = Apple
Array Elelment = Bannana
Array Elelment = Grape