The Java hashCode method is one of the String Methods, which is to find and return the hashCode of the User specified string. An empty string’s hashCode value is Zero, and this article will show how to use it with an example.
The formula behind the hashcode is: s[0]*31(n-1) + s[1]*31(n-2) + .. s(n-2). Here, s[i] is the ith character of the user-specified string, and n is the string length. The syntax of the string hashCode in Java Programming language is
public int hashCode() // It will return the integer Value as Output //In order to use in program String_Object.hashCode()
- String_Object: Please specify the valid Object.
How to find String hashCode in Java?
In this program, we use this Java method to find the hashCode value of the user-specified string.
package StringFunctions; public class HashcodeMethod { public static void main(String[] args) { String str = "Hi"; int a = str.hashCode(); int b = "Hello".hashCode(); int c = "Java Programming".hashCode(); int d = "hello world".hashCode(); int e = "tutorial gateway".hashCode(); System.out.println("Hashcode of the String str = " + a); System.out.println("Hashcode of the String = " + b); System.out.println("Hashcode of the String = " + c); System.out.println("Hashcode of the String = " + d); System.out.println("Hashcode of the String = " + e); } }
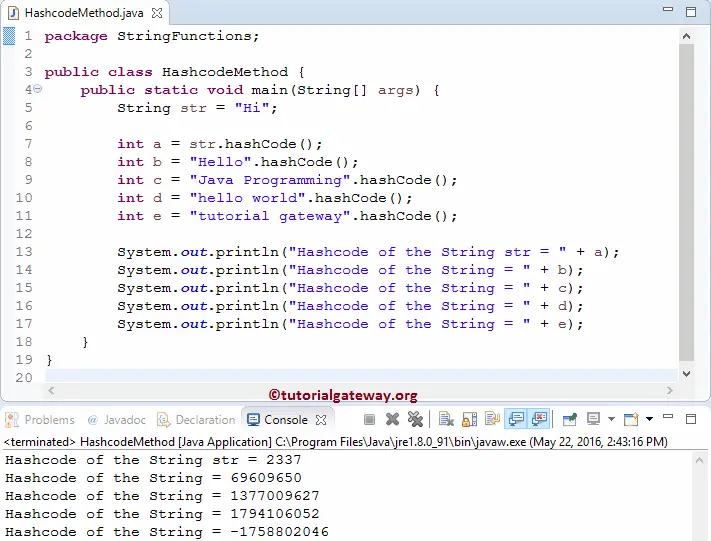
The following Java. string hashcode statements will find the value of str.
int a = str.hashCode();
If you observe the above Java screenshot, the statement returns 2337 as output. Let us find the same using the standard formula:
HashCode = s[0]*31(n-1) + s[1]*31(n-2) + .. s(n-2)
As we all know, the character at position 0 is H, the Character at position 1 is i, and the length is 2.
==> H*31(2-1) + i*31(2-2)
As we all know, the ASCII code of H is 72, and i is 105. It means,
==> 72 * 31 + 105 * 1 (Anything Power 0 is 1)
==> 2232 + 105 = 2337
The following four statements will find the Java string hashCode of the sentence or text and then assign the values to the integer variables b, c, d, and e.
TIP: Please refer ASCII Table to check the character codes of every character.
The last five System.out.println statements in this program will print the String Method output.