An array with more than one dimension is said to be a C# multi dimensional array. In C#, the 2D array is the purest form of the multi dimensional. In this section, we discuss the multi dimensional arrays.
C# Multi Dimensional Arrays Syntax
For the two dimensional
<datatype>[,] = new <datatype>[size];
For the three dimensional
<datatype>[,,] = new <datatype>[size]; . .
The two dimensional is also said to be rectangular, and it looks like
Int[,] ar = int[2,3]{{2,3,4}{5,6,7}};
Int[2,3] in a sense 2 rows and 3 columns
{2,3,4} is the first row, having three columns
{5,6,7} is the second row, having three columns
Accessing the C# 2d or multi dimensional array,
ar[0,0] = 2
ar[0,1] = 3
ar[0,2] = 4
ar[1,0] = 5
ar[1,1] = 6
ar[1,2] = 7
which looks like
2 3 4
5 6 7
Let us see an example code to demonstrate the multi dimensional.
C# Multi Dimensional Array Example
To demonstrate the same, we are using the C# 2d array in this example.
using System; class program { public static void Main() { int[,] ar = new int[2, 4] { { 1, 5, 8, 7 }, { 6, 4, 3, 2 } }; Console.WriteLine(ar[0, 0] + " " + ar[0, 1] + " " + ar[0, 2] + " " + ar[0, 3]); Console.WriteLine(ar[1, 0] + " " + ar[1, 1] + " " + ar[1, 2] + " " + ar[1, 3]); Console.WriteLine("Fourth element in first row of ar is ar[0,3]: {0}", ar[0, 3]); Console.WriteLine("Number of elements in first dimension: {0}", ar.GetLength(0)); Console.WriteLine("Number of elements in second dimension: {0}", ar.GetLength(1)); Console.WriteLine("Number of elements in ar: {0}", ar.Length); Console.WriteLine("Number of dimensions in ar: {0}", ar.Rank); Console.ReadLine(); } }
OUTPUT
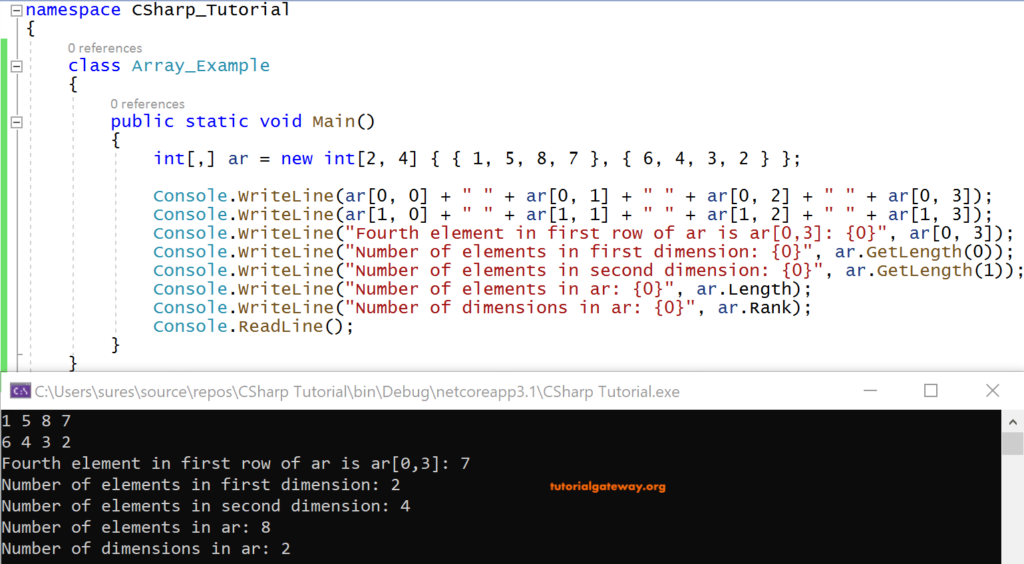
ANALYSIS
In this C# example, we have taken a two dimensional integer array having two rows and four columns.
Apart from printing those elements, we have also found out the length of each dimension.
Length of first dimension ar.GetLength{0} gives output 2.
Similarly ar.GetLength{1} is 4.
To know the total length of it is ar.Length whose output is 8.
And finally, the rank, nothing but the number of dimensions in it is ar.Rank, which is obviously 2.