The array class is the base class for all the arrays and is defined from the System namespace. The C# Array class provides functions for creating, manipulating, sorting, and searching arrays.
The C# Array class has the following properties.
Property | Usage |
---|---|
IsFixedSize | It gets a value based on whether the Array is of fixed size. |
IsReadOnly | Gets the value based on whether the Array is ReadOnly. |
Length | Gets a 32-bit integer, representing the Array elements in all the dimensions. |
LongLength | Gets a 64-bit integer, representing the elements of an Array in all the dimensions. |
Rank | It gets the total number of dimensions in an Array. |
Let us see an example of C# code demonstrating the properties of the Array class.
using System; class Program { static void Main(string[] args) { int[] arr = new int[] { 2, 8, 9, 4, 6 }; if (arr.IsFixedSize) { Console.WriteLine("Array is of fixed size"); Console.WriteLine("Array Size :" + arr.Length); Console.WriteLine("Rank :" + arr.Rank); } if (arr.IsReadOnly) Console.WriteLine("Array is read-only"); else Console.WriteLine("Array is not read-only"); } }
OUTPUT
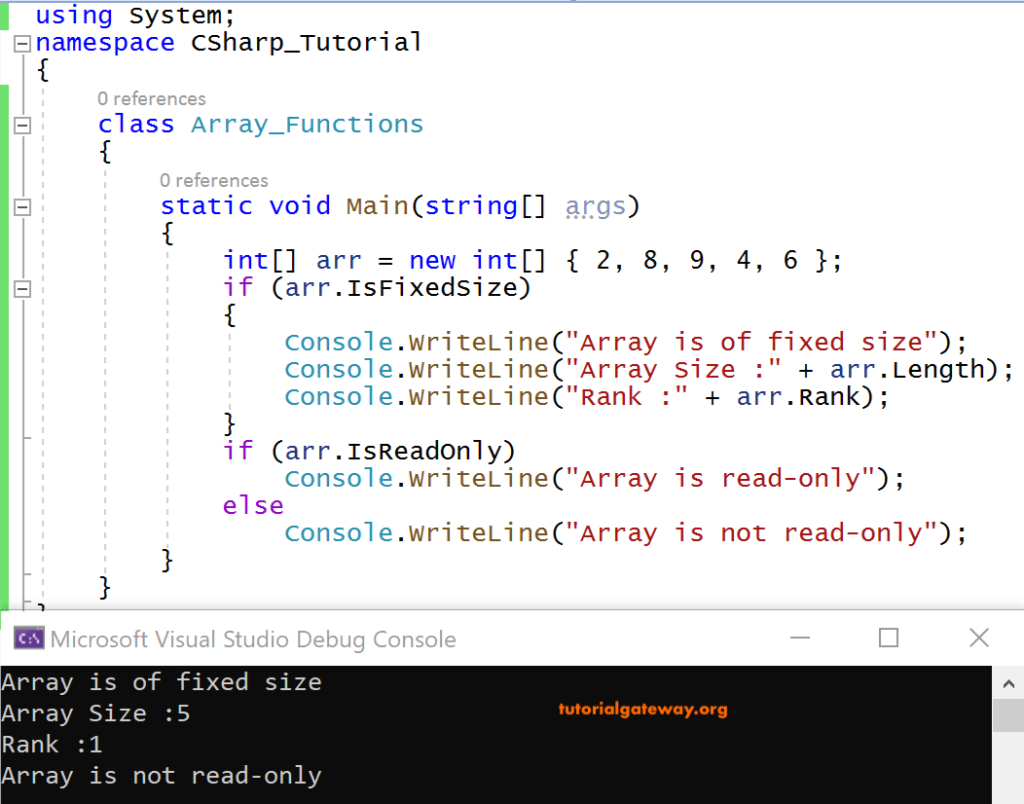
ANALYSIS
arr is an integer array that initializes with some values.
arr.IsFixedSize returns true since it has given a fixed number of values.
arr.Length will give the total number of elements in the C# array, which is 5.
arr.Rank will give the number of dimensions, which in this case, 1.
C# Array Class Functions
The DotNet framework provides various methods in the Array class. Let us see some of the most commonly used C# array functions.
Create Instance(<datatype> elementtype, int length)
CreateInstance() is used to construct an array.
The syntax for creating a single-dimensional array in C#
public static Array CreateInstance(typeof(<element type>), int length)
where typeof is the keyword, and the element type is the type of array to be created. And the length is the size of the array
The Syntax for creating two dimensional array in C#
public static Array CreateInstance(typeof(<element type>), int length1, int length2)
The element type is the type of array to be created
length1 is the first dimension of the array
length1 is the second dimension of the array
GetLowerBound(int dimension)
GetLowerBound() gets the index of the first element of the dimension specified in the array. The C# array function syntax is as shown below.
GetLowerBound(int dimension)
GetUpperBound(int dimension)
GetUpperBound() gets the index of the last element of the dimension specified in the array. The syntax of this C# array function is
GetUpperBound(int dimension)
GetValue(int32)
It gets the value at the specified position in one dimensional array. The index specified is a 32-bit integer.
GetValue(int32, int32)
It gets the value at the specified position in two dimensional array. The indexes specified are 32-bit integers.
C# Sort(Array) function
The static method Sort() is useful to sort items in an array.
Array.Sort() has many overloaded forms. The simplest form is the one that takes a single parameter, i.e., an array, which is to be sorted.
SetValue(object, int32)
This C# array function is useful to set values to the element at a specified position in the single dimensional array.
Reverse(Array)
Reverse() will take an array as a parameter and reverses the sequence of the items in one dimensional.
Clear()
This C# array function removes all items of it, and its length of it is set to zero.
Initialize()
This method is useful to initialize the elements of the array, which is the value type, by calling the default constructor of the value type.
Finalize()
This C# Array Function allows an object to free its resources to perform the operations such as cleanup before it goes for garbage collection.
Copy(source array, destination, int length)
This C# array function will copy the number of elements specified in length from the source array to the destination.
The number of dimensions in the destination array must be equal to the number of dimensions in the source. Apart from that, the destination must already have been dimensioned. And the sufficient number of elements to accommodate the data copied from the source array.
C# Array Functions Example
In this C# example, we demonstrate a few of the array functions such as createinstance, setvalue, copy, sort, and reverse.
using System; class Program { static void Array1(Array ar) { foreach (int i in ar) { Console.Write(i + " "); } } static void Main(string[] args) { Array arr = Array.CreateInstance(typeof(int), 6); arr.SetValue(8, 0); arr.SetValue(25, 1); arr.SetValue(64, 2); arr.SetValue(5, 3); arr.SetValue(10, 4); arr.SetValue(18, 5); Console.WriteLine("Elements in array arr are: "); foreach(int j in arr) Console.Write(j + " "); Array arr1 = Array.CreateInstance(typeof(int), 6); Array.Sort(arr); Console.WriteLine("\nElements of array arr after sorting: "); Array1(arr); Array.Copy(arr, arr1, arr.Length); Console.WriteLine("\nElements after copy from arr to array arr1 are: "); Array1(arr1); Array.Reverse(arr); Console.WriteLine("\nElements of array arr in reverse order: "); Array1(arr); Console.ReadKey(); } }
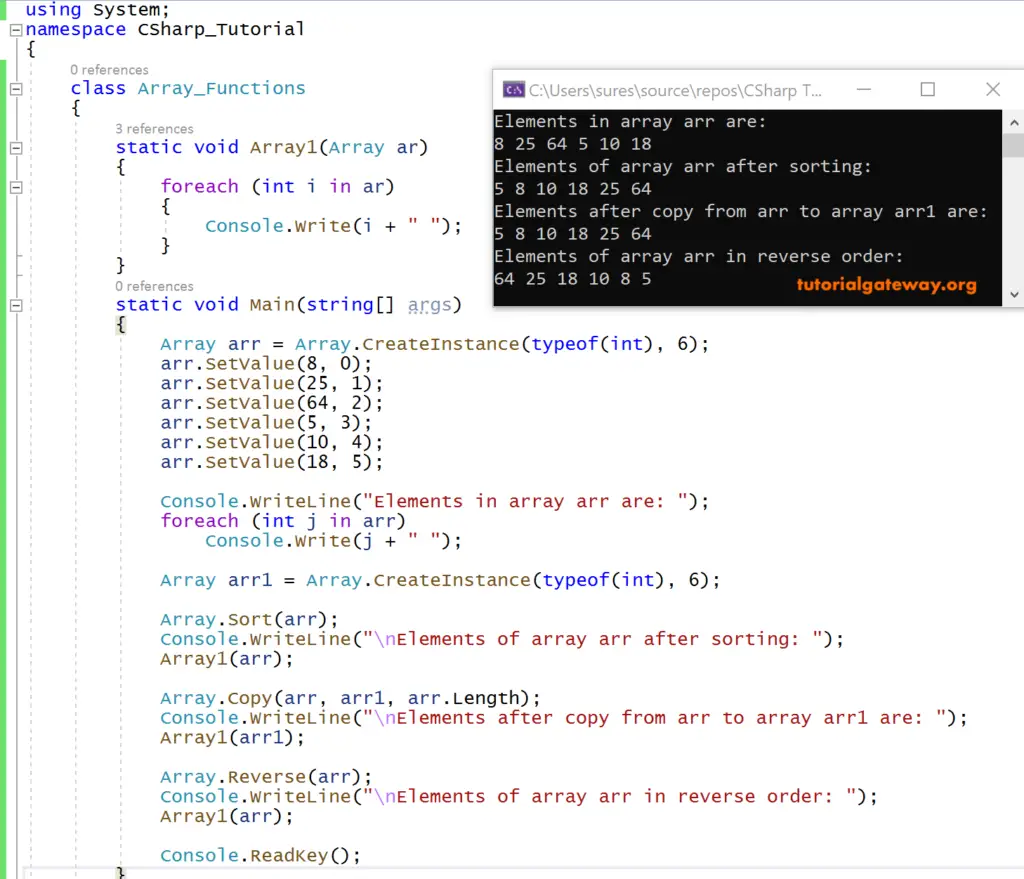
ANALYTICS
By using the CreateInstance() method, an arr of type integer and size six is created.
Using the SetValue() method, arr initialized with six elements of type integer.
Elements of arr are printed onto the console using a foreach loop.
We have declared a method outside the Main() method with the name Array1 with one parameter of type Array.
This method is defined using a foreach loop to print all the elements in the array passed as an argument to it.
Sort(arr) will sort all the elements in the arr.
Array1(arr), here arr is passed as an argument to Array1() method so that it will print all the elements in arr.
Array.Copy(arr, arr1, arr.Length)
This C# array function will copy all the elements of arr to the arr1. Now, arr1 has elements copied from arr.
Array1(arr1), here, arr1 is passed as an argument to the Array1() method so that it will print all the elements in arr1.
Reverse(arr) – It will reverse the sequence of all the elements in arr. Now arr has a reversed sequence of items in it.
Array1(arr), here arr is passed as an argument to Array1() method so that it will print all the elements in arr.