The C# Logical Operators are used to perform logical operations on operands. Use these logical operators to analyze multiple conditions. For instance, to find the largest of the three numbers, we use a > b && a > c.
The logical Operator’s conditions are usually enclosed in the parenthesis. The following table shows logical operators in C#.
Symbol | Operation | Example |
---|---|---|
&& | AND | 3 != 2 && 4==4 returns true |
|| | OR | 4= =4 || 6 = = 6 returns true |
&& Operator returns true if both the first and second condition is true.
|| returns true if either the first or second condition is true.
Here is the truth table for the C# Logical Operators && and || operations.
Operand 1 | Operand 2 | && Operation | || Operation |
---|---|---|---|
True | True | True | True |
True | False | False | True |
False | True | False | True |
False | False | False | False |
C# Logical operators Example
Let us see an example of the C# logical operators. x = 10 and y = 15 are the two integer variables, and the result is the boolean variable.
using System; class Logical_OPerators { static void Main() { int x = 10; int y = 15; bool result; result = (x == 10) && (y < 10); Console.WriteLine("x && y is {0}", result); result = (x == 10) || (y < 10); Console.WriteLine("x || y is {0}", result); } }
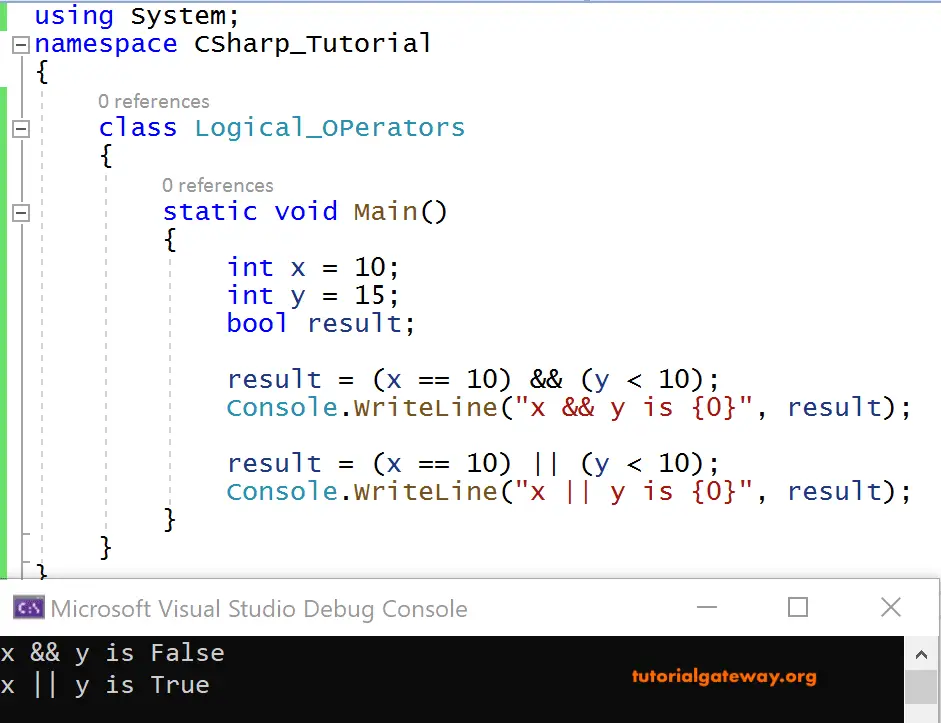
ANALYSIS
We have assigned x to 10, i.e., x = 10 and y to 15, i.e., y = 15.
Next, x ==10 returns true, y < 10 returns false. Comparing both the results, i.e., True && False returns False.
Now coming to ||, x = = 10 returns true, y < 10 returns false. As per the truth table, True || False returns True.