Write a C++ program to print triangle of same alphabets pattern in each row using for loop.
#include<iostream> using namespace std; int main() { int rows; cout << "Enter Triangle of Same Row Alphabets Rows = "; cin >> rows; cout << "Printing Triangle of Same Alphabets in each Row\n"; int alphabet = 65; for (int i = 0; i <= rows - 1; i++) { for (int j = rows - 1; j > i; j--) { cout << " "; } for (int k = 0; k <= i; k++) { cout << char(alphabet + i) << " "; } cout << "\n"; } }
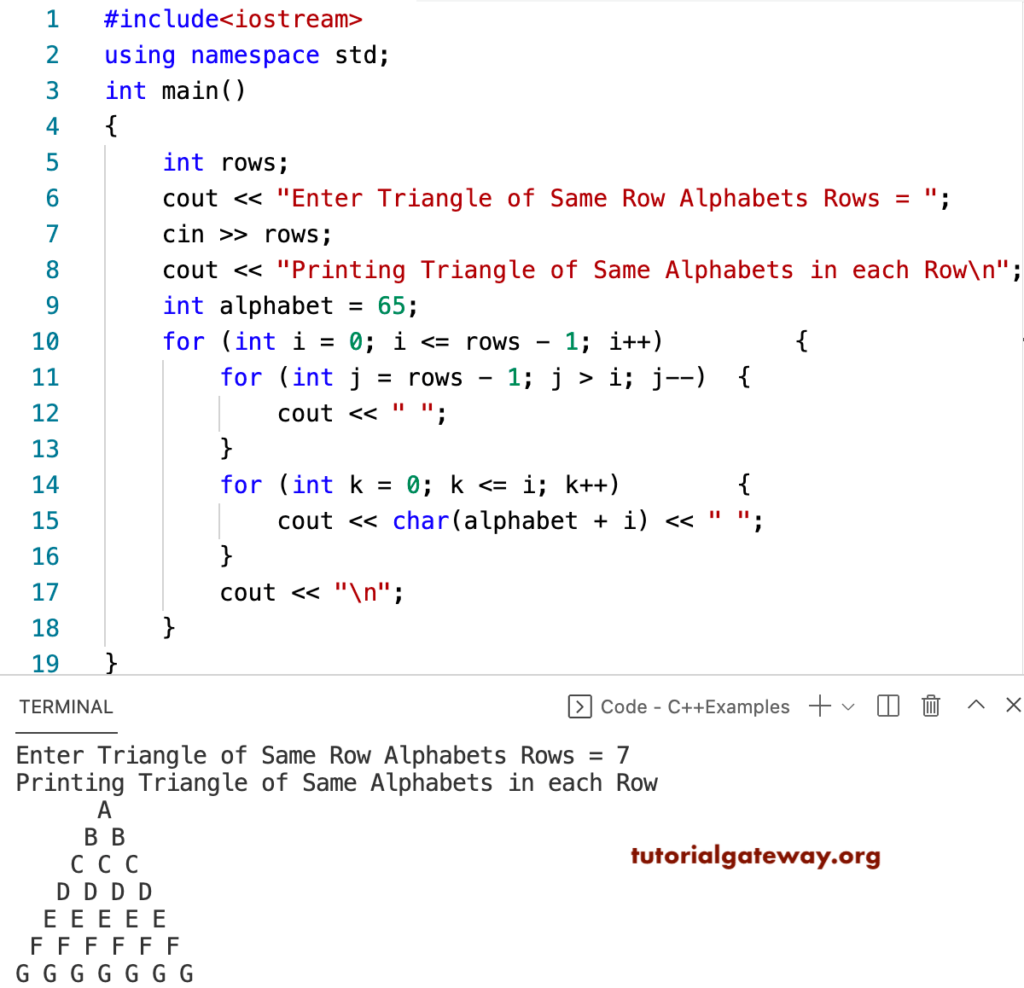
C++ program to print the triangle pattern of same row alphabets using a while loop.
#include<iostream> using namespace std; int main() { int rows; cout << "Enter Triangle of Same Row Alphabets Rows = "; cin >> rows; cout << "Printing Triangle of Same Alphabets in each Row\n"; int i, j, k, alphabet = 65; i = 0; while (i <= rows - 1) { j = rows - 1; while (j > i) { cout << " "; j--; } k = 0; while (k <= i) { cout << char(alphabet + i) << " "; k++; } cout << "\n"; i++; } }
Enter Triangle of Same Row Alphabets Rows = 13
Printing Triangle of Same Alphabets in each Row
A
B B
C C C
D D D D
E E E E E
F F F F F F
G G G G G G G
H H H H H H H H
I I I I I I I I I
J J J J J J J J J J
K K K K K K K K K K K
L L L L L L L L L L L L
M M M M M M M M M M M M M
This C++ pattern example prints the triangle of same alphabet in each single row using the do while loop.
#include<iostream> using namespace std; int main() { int rows, i, j, k, alphabet = 65; cout << "Enter Triangle of Same Row Alphabets Rows = "; cin >> rows; cout << "Printing Triangle of Same Alphabets in each Row\n"; i = 0; do { j = rows - 1; do { cout << " "; } while (j-- > i); k = 0; do { cout << char(alphabet + i) << " "; } while (++k <= i); cout << "\n"; } while (++i <= rows - 1); }
Enter Triangle of Same Row Alphabets Rows = 17
Printing Triangle of Same Alphabets in each Row
A
B B
C C C
D D D D
E E E E E
F F F F F F
G G G G G G G
H H H H H H H H
I I I I I I I I I
J J J J J J J J J J
K K K K K K K K K K K
L L L L L L L L L L L L
M M M M M M M M M M M M M
N N N N N N N N N N N N N N
O O O O O O O O O O O O O O O
P P P P P P P P P P P P P P P P
Q Q Q Q Q Q Q Q Q Q Q Q Q Q Q Q Q